Idempotent in Java
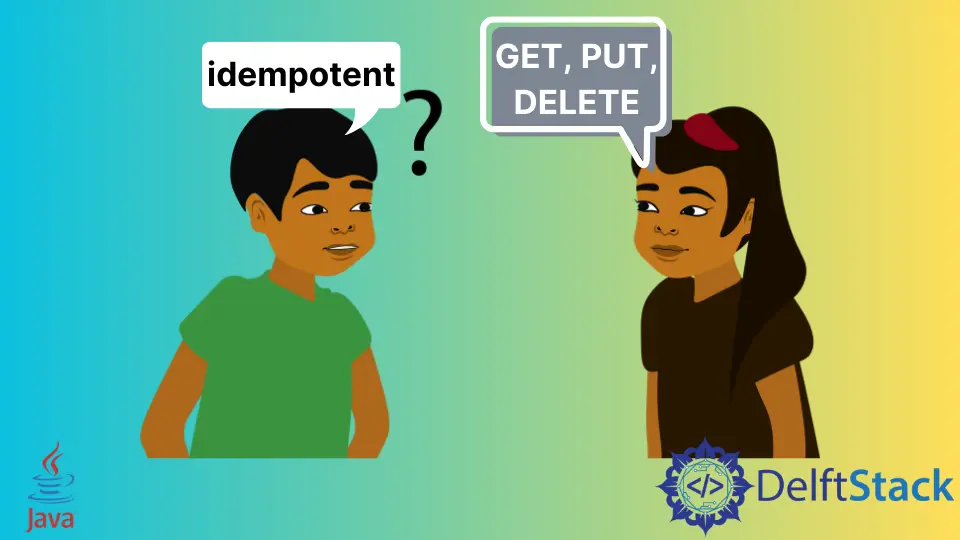
Idempotent means that we can apply an operation multiple times, but the result is always the same. For example, it doesn’t matter how often we call an idempotent method; the result will always be the same.
This tutorial demonstrates the use of idempotent in Java.
Idempotent in Java
As mentioned above, the Idempotent methods return the same results every time. For example, x=1;
is idempotent because it returns 1
every time, but x++;
cannot be idempotent because, with every call, it changes the results.
For example, if we close one object once, now we don’t have enough information to know if the object is idempotent after closing.
To check that, we need to close the object a second time, and if it throws an exception, that means the object is not idempotent, and if it returns the same results on closing, that means the object is idempotent.
Let’s try to implement an idempotent closeable
in Java.
package delftstack;
import java.io.Closeable;
public class Idempotent implements Closeable {
private static boolean closed;
public Idempotent() {
closed = false;
}
public void close() {
if (!Is_Closed()) {
closed = true;
}
}
public static boolean Is_Closed() {
return closed;
}
public static void main(String args[]) {
System.out.println(Is_Closed());
System.out.println(Is_Closed());
}
}
Closeable
is a class from java.io
. We implemented an idempotent closeable
by initializing a Boolean and setting it to false
in the Idempotent method; it will always return false
.
Output:
false
false
Idempotent Methods in Java
Idempotent methods term corresponds to the HTTP methods. The HTTP method is Idempotent when the result for the method is always the same no matter how many times we call the request.
Let’s try to understand the Idempotent HTTP methods with the example.
The GET
request: No matter how many times we make a GET
request, we will always get the same response if the request is implemented properly.
For example, we make a GET
request to see our results in the system. It will always be the same no matter how many times we request.
The HTTP methods are divided into two types one is Idempotent, and the other is Non-Idempotent. The table below demonstrates which HTTP methods are idempotent and which are not.
Method | Idempotent? |
---|---|
GET |
Yes |
PUT |
Yes |
POST |
No |
DELETE |
Yes |
HEAD |
Yes |
OPTIONS |
Yes |
PATCH |
No |
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook