How to Split String to Array in Java
-
Use
split(delimiter)
to Split String to an Array in Java -
Use
split(regex, limit)
to Split String to an Array in Java and Keep the Trailing Empty Strings
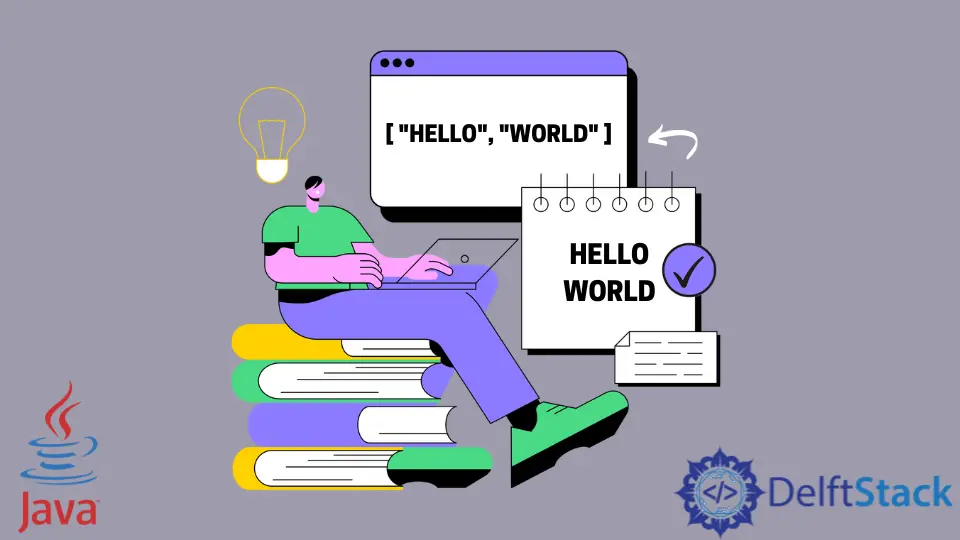
This tutorial discusses methods to split string to an array in Java.
Suppose we have a comma-separated string John, 23, $4500
. We need to split the data based on the comma and store it in an array like this: ["John", "23", "$4500"]
. This is where the split()
method comes in handy.
Use split(delimiter)
to Split String to an Array in Java
We need to pass the delimiter to split the string based on it. The split()
method would break the string on every delimiter occurrence and store each value in the array. The below example illustrates this.
public class MyClass {
public static void main(String args[]) {
String data = "1,2,3,,5,6,,";
String[] split = data.split(",");
for (int i = 0; i < split.length; i++) System.out.println(split[i]);
System.out.println("Done");
}
}
Output:
1
2
3
5
6
Although we have two empty strings at the end, the resulting array doesn’t contain the last two empty strings. It is because the default behavior of split()
removes all the trailing empty strings from the resulting array.
Use split(regex, limit)
to Split String to an Array in Java and Keep the Trailing Empty Strings
We can override this behavior by passing an additional parameter to the split()
function: split(regex, limit)
. Passing a negative limit
would ensure that the regex pattern is applied as many times as possible, thereby including all the trailing empty strings from the split.
The below example illustrates this:
public class MyClass {
public static void main(String args[]) {
String data = "1,2,3,,5,6,,";
String[] split = data.split(",", -1);
for (int i = 0; i < split.length; i++) System.out.println(split[i]);
System.out.println("Done");
}
}
Output:
1
2
3
5
6
Done
We can see that it has printed the last two trailing empty strings now.