How to Sort Objects in ArrayList by Date in Java
Asad Riaz
Feb 02, 2024
-
comparable<>
Method to Sort Objects inArrayList
by Dates in Java -
collections.sort()
Method to Sort Objects in ArrayList by Date in Java -
list.sort()
Method to Sort Objects inArrayList
by Date in Java
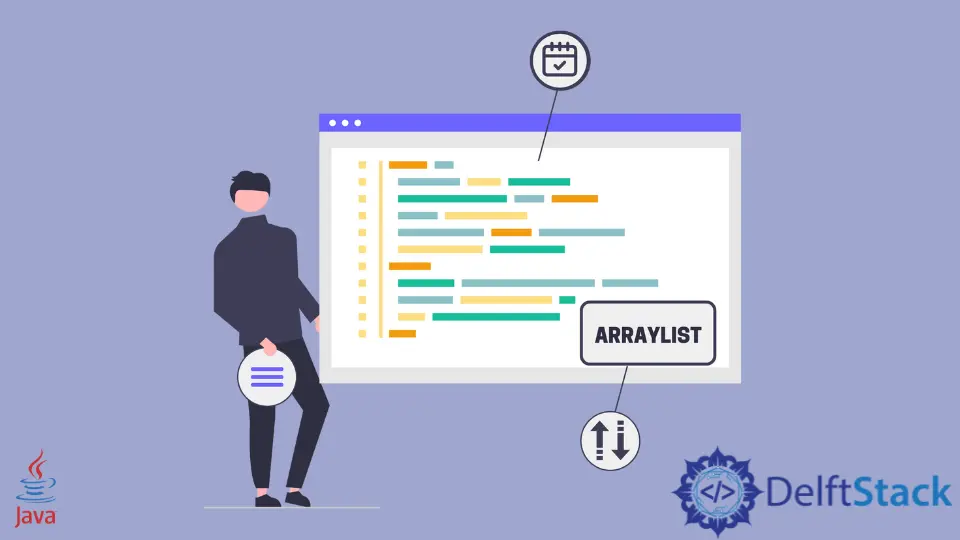
We have multiple methods to sort objects in ArrayList
by date in Java. These sorting can be done based on the date or any other condition. For example, making object comparable<>
, Collections.sort()
and list.sort()
methods.
comparable<>
Method to Sort Objects in ArrayList
by Dates in Java
The first method works by making the object comparable<>
along with using classes compareTo()
, compare()
and collections.sort()
. We will create a new class of DateItem
object and implement Comparator<DateItem>
interface to sort the array.
Example Codes:
Java
javaCopyimport java.util.*;
public class SimpleTesing {
static class DateItem {
String datetime;
DateItem(String date) {
this.datetime = date;
}
}
static class SortByDate implements Comparator<DateItem> {
@Override
public int compare(DateItem a, DateItem b) {
return a.datetime.compareTo(b.datetime);
}
}
public static void main(String args[]) {
List<DateItem> dateList = new ArrayList<>();
dateList.add(new DateItem("2020-03-25"));
dateList.add(new DateItem("2019-01-27"));
dateList.add(new DateItem("2020-03-26"));
dateList.add(new DateItem("2020-02-26"));
Collections.sort(dateList, new SortByDate());
dateList.forEach(date -> { System.out.println(date.datetime); });
}
}
Output:
textCopy2019-01-27
2020-02-26
2020-03-25
2020-03-26
collections.sort()
Method to Sort Objects in ArrayList by Date in Java
The collections.sort()
method could perform the sorting by date in an ArrayList
.
Example Codes:
Java
javaCopyimport java.util.*;
public class SimpleTesting {
public static void main(String[] args) {
List<String> dateArray = new ArrayList<String>();
dateArray.add("2020-03-25");
dateArray.add("2019-01-27");
dateArray.add("2020-03-26");
dateArray.add("2020-02-26");
System.out.println("The Object before sorting is : " + dateArray);
Collections.sort(dateArray);
System.out.println("The Object after sorting is : " + dateArray);
}
}
Output:
textCopyThe Object before sorting is : [2020-03-25, 2019-01-27, 2020-03-26, 2020-02-26]
The Object after sorting is : [2019-01-27, 2020-02-26, 2020-03-25, 2020-03-26]
list.sort()
Method to Sort Objects in ArrayList
by Date in Java
The list.sort()
method of Java combines with a lambda expression to perform the sorting by date
in an ArrayList
.
Example Codes:
Java
javaCopyimport java.util.*;
public class SimpleTesting {
public static void main(String[] args) {
List<String> dateArray = new ArrayList<String>();
dateArray.add("2020-03-25");
dateArray.add("2019-01-27");
dateArray.add("2020-03-26");
dateArray.add("2020-02-26");
System.out.println("The Object before sorting is : " + dateArray);
dateArray.sort((d1, d2) -> d1.compareTo(d2));
System.out.println("The Object after sorting is : " + dateArray);
}
}
Output:
textCopyThe Object before sorting is : [2020-03-25, 2019-01-27, 2020-03-26, 2020-02-26]
The Object after sorting is : [2019-01-27, 2020-02-26, 2020-03-25, 2020-03-26]