How to Read a Large Text File Line by Line in Java
-
BufferedReader
to Read File Line by Line in Java -
Stream
to Read File Line by Line in Java -
Scanner
to Read File Line by Line in Java
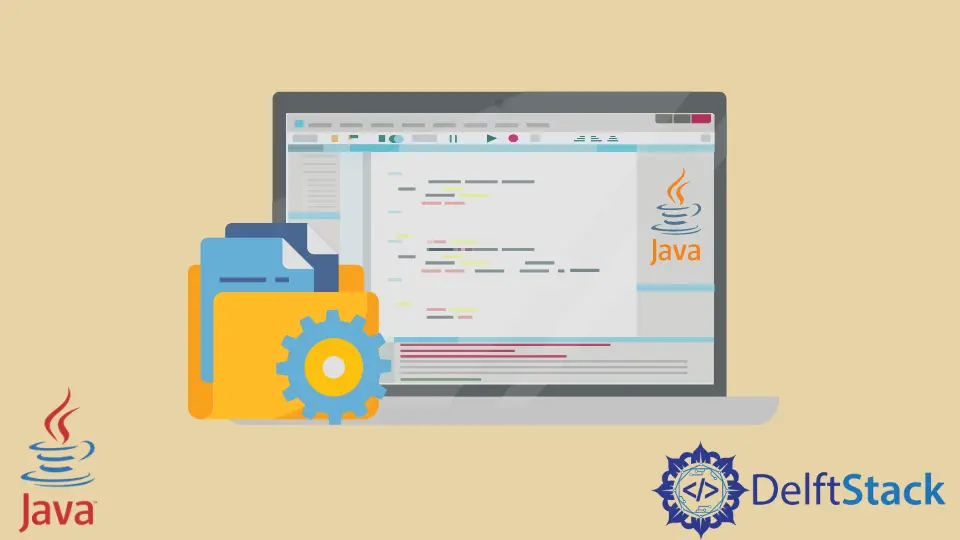
This tutorial discusses methods to read a large text file line by line efficiently in Java.
There are numerous methods to read a text file in Java. However, this tutorial is specific to reading large text files, and we will discuss the three most efficient methods to read large text files quickly.
BufferedReader
to Read File Line by Line in Java
BufferedReader
class in Java reads text from a given character-input stream, buffering characters to provide for the efficient reading of characters, arrays, and lines. This method provides an efficient line by line reading for the input files with a considerably large file size.
The below example illustrates the use of BufferedReader
to read a txt
file and output its content line by line.
import java.io.*;
public class Main {
public static void main(String[] args) {
String file = "my-file.txt";
try (BufferedReader br = new BufferedReader(new FileReader(file))) {
String line;
while ((line = br.readLine()) != null) {
System.out.println(line);
}
} catch (IOException e) {
System.out.println("An error occurred.");
e.printStackTrace();
}
}
}
Stream
to Read File Line by Line in Java
Java 8 and above users can also use Stream
to read large files line by line. The below example illustrates the use of Stream
to read a txt
file and output its content line by line.
import java.io.*;
import java.nio.file.*;
import java.util.stream.*;
public class Main {
public static void main(String[] args) {
String file = "my-file.txt";
try (Stream<String> stream = Files.lines(Paths.get(fileName))) {
stream.forEach(System.out::println);
} catch (IOException e) {
System.out.println("An error occurred.");
e.printStackTrace();
}
}
}
The above two methods discussed would read the input file line by line instead of reading the whole file into memory. Therefore these two methods are highly efficient if we have a huge file that can not be fully read into the memory.
However, if our memory is large enough to read the input file fully, we can also try the below method.
Scanner
to Read File Line by Line in Java
Scanner
class in Java is a simple text scanner which can parse primitive types and strings using regular expressions. Scanner(File source)
reads the complete file into memory and then processes it line by line.
The example below illustrates the use of Scanner
to read a txt
file and output its content line by line.
import java.io.*;
import java.util.*;
public class Main {
public static void main(String[] args) throws IOException {
String fileName = "my-file.txt";
Scanner scan = new Scanner(new File(fileName));
while (scan.hasNextLine()) {
String line = scan.nextLine();
System.out.println(line);
}
}
}
We discussed three methods to read a large text file in Java and process it line by line. Each method had some constraints and advantages that we must consider while deciding which method to use in a particular scenario.