How to Initialize an Array in Java
-
dataType arrayName[];
to Declare a New Array -
arrayName = new dataType[size];
to Allocate the Size of the Array -
arrayName[index] = value/element
to Initialize Array With Values/Elements -
dataType[] arrayName = new dataType[]{elements}
to Initialize Array Without Size
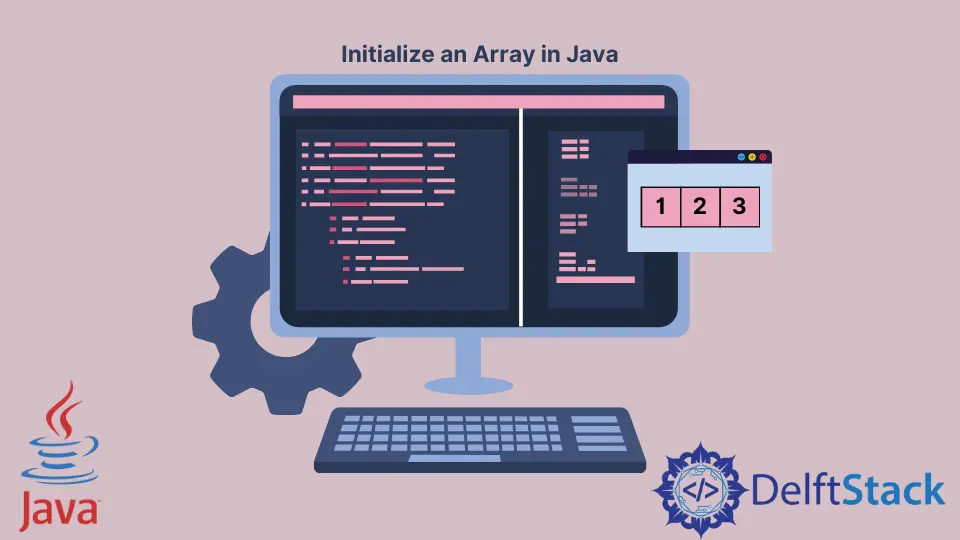
This article shows how to declare and initialize an array with various examples. There are two ways to initialize an array in Java.
dataType arrayName[];
to Declare a New Array
The most common syntax is dataType arrayName[];
.
Below is an example of declaring an array which will hold Integer values in it.
public class Main {
public static void main(String[] args) {
int[] arrayInt;
}
}
arrayName = new dataType[size];
to Allocate the Size of the Array
Arrays in Java holds a fixed number of elements which are of the same type. It means that it is necessary to specify the array size at the time of initialization. When the array is initialized, it is stored in a shared memory in which the memory locations are given to that array according to its size.
A simple example can explain this much better.
public class Main {
public static void main(String[] args) {
int[] arrayInt = new int[10];
System.out.println("The size of the array is: " + arrayInt.length);
}
}
In the above example,
arrayInt
is an array which has been allotted the size of 10.
The new
keyword must be used to instantiate an array.
The output shows the total size of the array, but there are no values inside it.
Output:
The size of the array is: 10
arrayName[index] = value/element
to Initialize Array With Values/Elements
The first method to initialize an array is by index number where the value is to be stored.
Let’s take a look at the example to understand it clearly.
public class Main {
public static void main(String[] args) {
int[] arrayInt = new int[5];
arrayInt[0] = 10;
arrayInt[1] = 20;
arrayInt[2] = 30;
arrayInt[3] = 40;
arrayInt[4] = 50;
for (int i = 0; i < arrayInt.length; i++) {
System.out.println(arrayInt[i] + " is stored at index " + i);
}
}
}
Output:
10 is stored at index 0
20 is stored at index 1
30 is stored at index 2
40 is stored at index 3
50 is stored at index 4
dataType[] arrayName = new dataType[]{elements}
to Initialize Array Without Size
We have another way to initialize an array meanwhile the array elements are directly stored during the declaration of the array. It is useful when the size of the array is already known and also to make the code more clear to read.
Below is the example of an array that contains string values.
public class Main {
public static void main(String[] args) {
String[] arrayString = new String[] {"one", "two", "three", "four", "five"};
for (int i = 0; i < arrayInt.length; i++) {
System.out.println(arrayInt[i] + " is stored at index " + i);
}
}
}
Output:
one is stored at index 0
two is stored at index 1
three is stored at index 2
four is stored at index 3
five is stored at index 4
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedIn