How to Get the Length of a 2D Array in Java
- Understanding 2D Arrays in Java
- Using the Length Property
- Iterating Through the Array
- Using Arrays Utility Class
- Conclusion
- FAQ
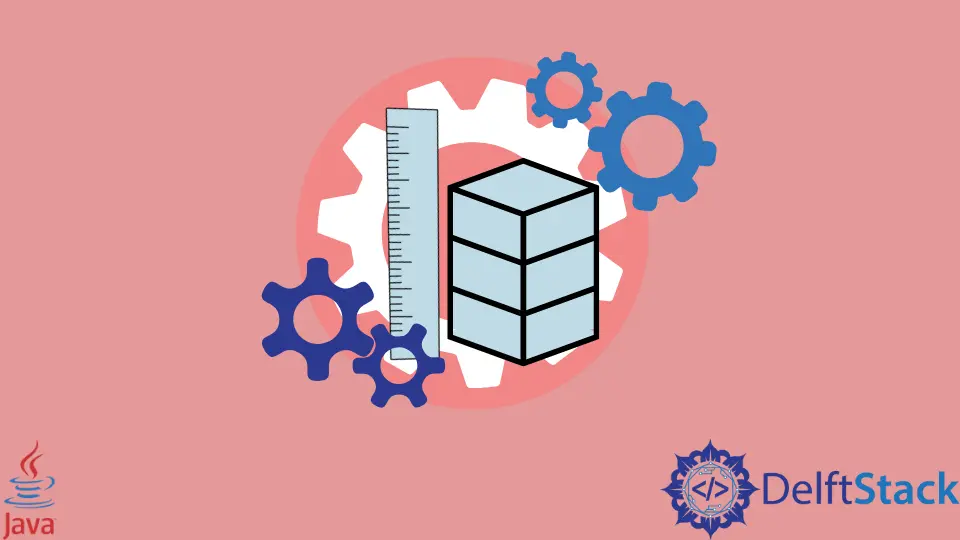
When working with arrays in Java, particularly 2D arrays, knowing how to determine their length is crucial. Whether you’re handling matrices for mathematical computations, grids for game development, or simply organizing data, understanding the structure of your 2D array is fundamental.
In this article, we’ll explore various methods to get the length of a 2D array in Java. We’ll break down the process step-by-step, ensuring that you not only grasp the concept but also feel confident applying it in your projects. Let’s dive in!
Understanding 2D Arrays in Java
Before we jump into the methods, it’s essential to understand what a 2D array is. In Java, a 2D array is essentially an array of arrays. Each element in the main array can hold another array, which allows you to create a grid-like structure. This is particularly useful for applications that require two-dimensional data representation, such as image processing or game boards.
To declare a 2D array in Java, you can use the following syntax:
dataType[][] arrayName = new dataType[rows][columns];
For example, if you want to create a 2D array of integers with 3 rows and 4 columns, you would write:
int[][] myArray = new int[3][4];
Now that we have a basic understanding of 2D arrays, let’s explore how to get their lengths.
Using the Length Property
One of the simplest methods to get the length of a 2D array in Java is by utilizing the length
property. This property can be used to find out how many rows and columns are present in the array.
Here’s how you can do it:
int[][] myArray = new int[3][4];
int numberOfRows = myArray.length;
int numberOfColumns = myArray[0].length;
System.out.println("Number of rows: " + numberOfRows);
System.out.println("Number of columns: " + numberOfColumns);
Output:
Number of rows: 3
Number of columns: 4
In this example, we first create a 2D array named myArray
with 3 rows and 4 columns. To find the number of rows, we simply access the length
property of the array itself. For the number of columns, we access the first row (myArray[0]
) and retrieve its length. This method is straightforward and effective for fixed-size 2D arrays.
However, it’s important to note that if the rows of the 2D array are not of equal length, accessing myArray[0].length
may not represent the lengths of all rows. Thus, it’s essential to be cautious when working with jagged arrays.
Iterating Through the Array
Another method to get the length of a 2D array in Java is by iterating through the array. This approach can be particularly useful when dealing with jagged arrays, where each row may have a different number of columns.
Here is how you can do it:
int[][] jaggedArray = {
{1, 2},
{3, 4, 5},
{6, 7, 8, 9}
};
int numberOfRows = jaggedArray.length;
System.out.println("Number of rows: " + numberOfRows);
for (int i = 0; i < numberOfRows; i++) {
int numberOfColumns = jaggedArray[i].length;
System.out.println("Number of columns in row " + i + ": " + numberOfColumns);
}
Output:
Number of rows: 3
Number of columns in row 0: 2
Number of columns in row 1: 3
Number of columns in row 2: 4
In this example, we create a jagged array named jaggedArray
. First, we retrieve the number of rows using the length
property. Then, we loop through each row to determine its length. This method provides a comprehensive view of the structure of a jagged array, allowing you to handle cases where the number of columns varies from one row to another.
Using Arrays Utility Class
Java provides a utility class called Arrays
that contains various methods for working with arrays. Although it doesn’t have a direct method to get the length of a 2D array, it can be helpful in certain scenarios.
Here’s a method that combines the use of the Arrays
class with our existing techniques:
import java.util.Arrays;
int[][] myArray = {
{1, 2, 3},
{4, 5},
{6, 7, 8, 9}
};
int numberOfRows = myArray.length;
System.out.println("Number of rows: " + numberOfRows);
for (int i = 0; i < numberOfRows; i++) {
System.out.println("Row " + i + ": " + Arrays.toString(myArray[i]));
System.out.println("Number of columns: " + myArray[i].length);
}
Output:
Number of rows: 3
Row 0: [1, 2, 3]
Number of columns: 3
Row 1: [4, 5]
Number of columns: 2
Row 2: [6, 7, 8, 9]
Number of columns: 4
In this example, we use the Arrays.toString()
method to print the contents of each row. This method not only allows us to see the values but also confirms the number of columns in each row. While this method may not directly provide the length, it enhances the readability of your output, making it easier to understand the structure of your 2D array.
Conclusion
Getting the length of a 2D array in Java is a fundamental skill that can significantly enhance your programming capabilities. Whether using the length
property, iterating through the array, or leveraging the Arrays
utility class, each method has its advantages and specific use cases. Understanding these techniques will help you better manage and manipulate 2D arrays in your Java applications. So the next time you find yourself working with multidimensional data, you’ll be well-equipped to handle it with ease.
FAQ
-
How do I create a 2D array in Java?
You can create a 2D array in Java using the syntaxdataType[][] arrayName = new dataType[rows][columns];
. -
Can the rows of a 2D array have different lengths?
Yes, in Java, you can create jagged arrays where each row can have a different number of columns. -
What happens if I try to access an index that is out of bounds?
Attempting to access an out-of-bounds index will result in anArrayIndexOutOfBoundsException
. -
Is it possible to store different data types in a 2D array?
No, all elements in a 2D array must be of the same data type. However, you can use an array ofObject
type to store different data types. -
How can I initialize a 2D array with values?
You can initialize a 2D array with values using curly braces, like this:int[][] myArray = {{1, 2}, {3, 4}};
.