How to Get Keys From HashMap in Java
Hassan Saeed
Feb 02, 2024
-
Use
keySet()
to Get a Set of Keys From aHashMap
in Java -
Use
keySet()
to Get an Array of Keys From aHashMap
in Java
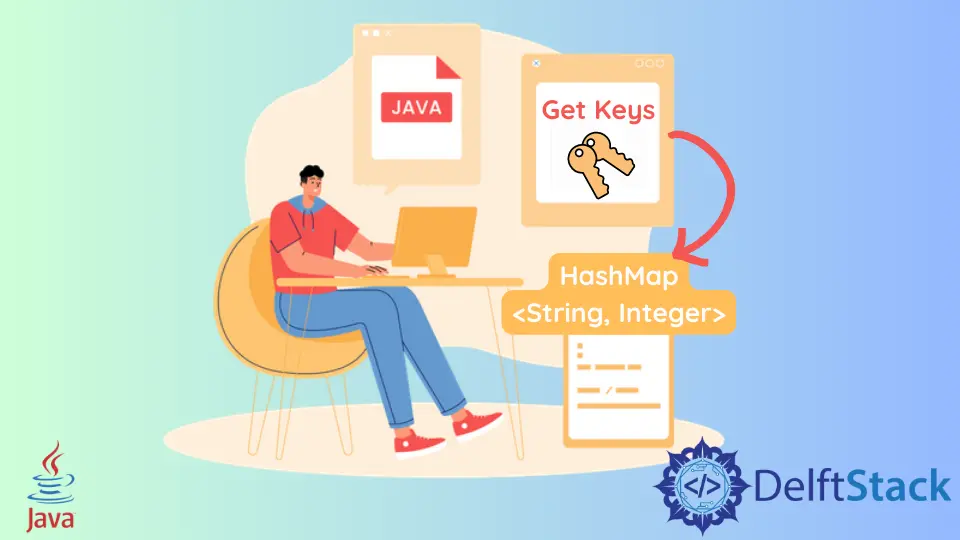
This tutorial discusses methods to get the keys from a HashMap
in Java.
Use keySet()
to Get a Set of Keys From a HashMap
in Java
The simplest way to get the keys from a HashMap
in Java is to invoke the keySet()
method on your HashMap
object. It returns a set
containing all the keys from the HashMap
.
In the example below, we will first create a HashMap
object, insert some values in it, and then use keySet()
to get the keys.
import java.util.*;
public class MyClass {
public static void main(String args[]) {
// Create a HashMap with some values
HashMap<String, Integer> map = new HashMap<String, Integer>();
map.put("Monday", 5);
map.put("Tuesday", 6);
map.put("Wednesday", 10);
// Invoke keySet() on the HashMap object to get the keys as a set
Set<String> keys = map.keySet();
for (String key : keys) {
System.out.println(key);
}
}
}
Output:
Monday
Wednesday
Tuesday
Use keySet()
to Get an Array of Keys From a HashMap
in Java
Often we prefer to work with an array instead of a set
. The below example illustrates how to use keySet()
to get an array of keys from a HashMap
in Java.
import java.util.*;
public class MyClass {
public static void main(String args[]) {
// Create a HashMap with some values
HashMap<String, Integer> map = new HashMap<String, Integer>();
map.put("Monday", 5);
map.put("Tuesday", 6);
map.put("Wednesday", 10);
// Invoke keySet() and use toArray() to get an array of keys
Object[] keys = map.keySet().toArray();
for (int i = 0; i < keys.length; i++) {
System.out.println(keys[i]);
}
}
}
Output:
Monday
Wednesday
Tuesday