How to Convert String to Double in Java
-
Double.parseDouble()
Method to Convert a String to a Double -
Double.valueOf()
Method to Convert a String to a Double -
DecimalFormat.parse
to Convert a String to a Double -
Handle
NumberFormatException
While Converting a String Value to a Double Value
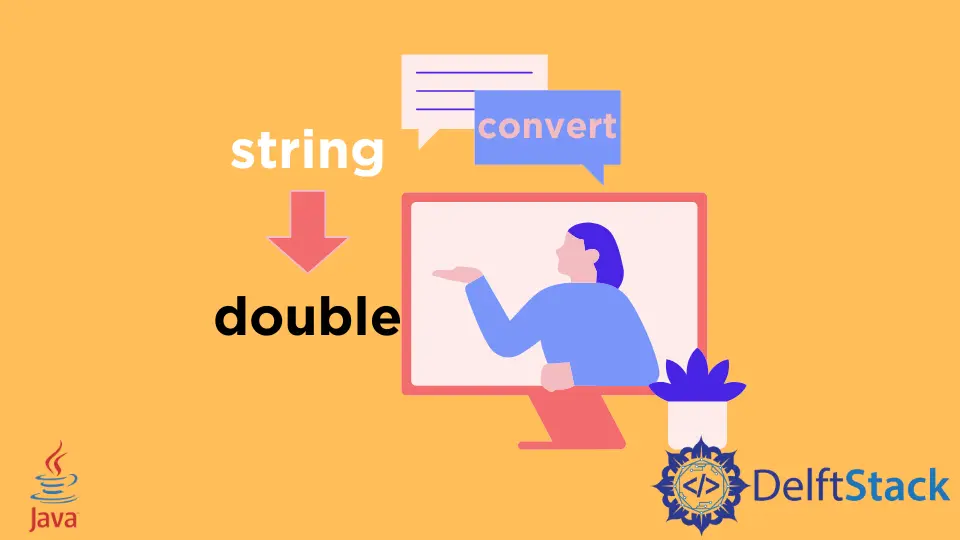
We will introduce different ways to convert the given string into a double in Java with some easy to understand examples.
Double.parseDouble()
Method to Convert a String to a Double
The best and easiest way to cast a string to double in Java is by using the static method parseDouble
of the class Double
.
Double.parseDouble
returns a primitive type.
public class Main {
public static void main(String[] args) {
String stringValue = "12.34";
double doubleValue = Double.parseDouble(stringValue);
System.out.println("Converted value: " + doubleValue);
}
}
Output:
Converted value: 12.34
It can be a negative or positive value in the string; this method will parse string to double
correctly.
public class Main {
public static void main(String[] args) {
String stringValueNegative = "-12.34";
String stringValuePositive = "+12.34";
double doubleValueNegative = Double.parseDouble(stringValueNegative);
double doubleValuePositive = Double.parseDouble(stringValuePositive);
System.out.println("Converted negative value: " + doubleValueNegative);
System.out.println("Converted positive value: " + doubleValuePositive);
}
}
Output:
Converted negative value: -12.34
Converted positive value: 12.34
Double.valueOf()
Method to Convert a String to a Double
When we need a Double
object instead of a primitive type double, we can use the Double.valueOf
method to get a double object value from a string.
public class Main {
public static void main(String[] args) {
String stringValueNegative = "-12.08d";
String stringValuePositive = "+12.3400";
Double doubleValueNegative = Double.valueOf(stringValueNegative);
Double doubleValuePositive = Double.valueOf(stringValuePositive);
System.out.println(doubleValueNegative);
System.out.println(doubleValuePositive);
}
}
Output:
-12.08
12.34
DecimalFormat.parse
to Convert a String to a Double
If the value we desire is something like 1,11,111.12d
, we can use the DecimalFormat
class to parse the string to a doubleValue()
.
But as the DecimalFormat
class can return different values like int
or byte
, it is necessary to do all the operations in the try-catch
block. And as we desire a doubleValue, we have to use the doubleValue()
method to definitively get the double
type.
import java.text.DecimalFormat;
import java.text.ParseException;
public class Main {
public static void main(String[] args) {
String stringValue = "1,11,111.12d";
try {
double doubleValue = DecimalFormat.getNumberInstance().parse(stringValue).doubleValue();
System.out.println(doubleValue);
} catch (ParseException e) {
e.printStackTrace();
}
}
}
Output:
111111.12
Handle NumberFormatException
While Converting a String Value to a Double Value
Sometimes we might get NumberFormatException
. It happens because the string value is not a valid double value.
To make it more clear, we will see a simple example which throws this exception.
public class Main {
public static void main(String[] args) {}
String stringValue = "1,11";
double d = Double.parseDouble(stringValue);
System.out.println(d);
}
}
Output:
Exception in thread "main" java.lang.NumberFormatException: For input string: "1,11"
at java.base/jdk.internal.math.FloatingDecimal.readJavaFormatString(FloatingDecimal.java:2054)
at java.base/jdk.internal.math.FloatingDecimal.parseDouble(FloatingDecimal.java:110)
at java.base/java.lang.Double.parseDouble(Double.java:543)
at com.company.Main.main(Main.java:14)
The exception happens because 1,11
is not a valid double value. The solution for this error is to replace comma with a decimal point like we are doing below.
public class Main {
public static void main(String[] args) {
String stringValue = "1,11";
double d = Double.parseDouble(stringValue.replace(",", "."));
System.out.println(d);
}
}
Output:
1.11
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedInRelated Article - Java Double
- How to Convert Int to Double in Java
- Double in Java
- How to Compare Doubles in Java
- Float and Double Data Type in Java
- How to Convert Double to String in Java
- How to Convert Double to Float in Java