How to Convert String to Date in Java
Hassan Saeed
Feb 02, 2024
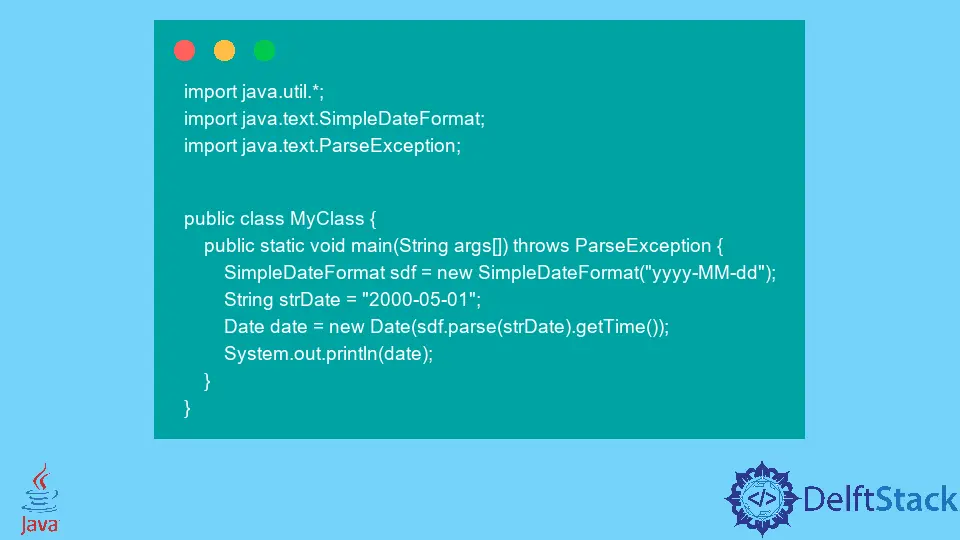
This tutorial discusses methods to convert a string to a date
in Java.
Use SimpleDateFormat
to Convert a String to date
in Java
The simplest way to convert a string to date
in Java is by using the SimpleDateFormat
class. First, we need to define the date format our string represents, such as yyyy-MM-dd
. The below example illustrates this:
import java.text.ParseException;
import java.text.SimpleDateFormat;
import java.util.*;
public class MyClass {
public static void main(String args[]) throws ParseException {
SimpleDateFormat sdf = new SimpleDateFormat("yyyy-MM-dd");
String strDate = "2000-05-01";
Date date = new Date(sdf.parse(strDate).getTime());
System.out.println(date);
}
}
Output:
Mon May 01 00:00:00 GMT 2000
Let us try this with a few more different date formats. The below example converts the string - "January 2, 2010"
to a date
in Java.
import java.text.ParseException;
import java.text.SimpleDateFormat;
import java.util.*;
public class MyClass {
public static void main(String args[]) throws ParseException {
SimpleDateFormat sdf = new SimpleDateFormat("MMMM d, yyyy");
String strDate = "January 2, 2010";
Date date = new Date(sdf.parse(strDate).getTime());
System.out.println(date);
}
}
Output:
Sat Jan 02 00:00:00 GMT 2010
Similarly, you can define use any date format and convert the string representation to date
in Java.