How to Convert List to Array in Java
- Convert a List to an Array of in Java
-
Use
toArray()
to Convert a List to an Array of Reference Types in Java -
Use
stream()
to Convert a List to an Array in Java
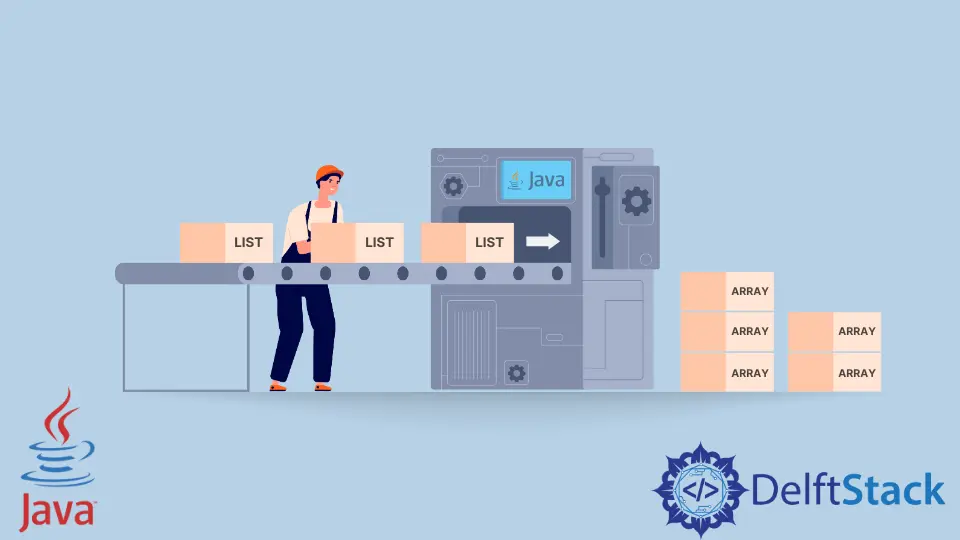
This tutorial discusses methods to convert a list to an array in Java.
Convert a List to an Array of in Java
This method simply creates a new array with the size same as the list and iterates over the list filling the array with elements. The below example illustrates this:
import java.util.*;
public class MyClass {
public static void main(String args[]) {
List<Integer> list = new ArrayList();
list.add(1);
list.add(2);
int[] array = new int[list.size()];
for (int i = 0; i < list.size(); i++) array[i] = list.get(i);
}
}
The resulting array contains all the elements from the list. Note that this method should not be used if the resulting array is of non-primitive types.
Use toArray()
to Convert a List to an Array of Reference Types in Java
This method is used if the list contains elements of reference types such as objects of a class. We can use the built-in method toArray()
to convert a list of such type to an array. The below example illustrates this:
import java.util.*;
public class MyClass {
public static void main(String args[]) {
// Define the Foo class
class Foo {
private int value;
public Foo(int value) {
this.value = value;
}
public int getValue() {
return value;
}
}
// Create instances of Foo
Foo obj1 = new Foo(42);
Foo obj2 = new Foo(99);
// Create a List of Foo objects
List<Foo> list = new ArrayList<>();
// Add the obj1 and obj2 to the list
list.add(obj1);
list.add(obj2);
// Convert the list to an array
Foo[] array = list.toArray(new Foo[0]);
// Print the values from the array
for (Foo foo : array) {
System.out.println("Value: " + foo.getValue());
}
}
}
Output:
Value: 42
Value: 99
Use stream()
to Convert a List to an Array in Java
For Java 8 and above, we can also use Stream API’s stream()
method to convert a list to an array in Java. The below example illustrates this:
import java.util.*;
public class MyClass {
public static void main(String args[]) {
List<Integer> list = new ArrayList<>();
list.add(1);
list.add(2);
Integer[] integers = list.stream().toArray(Integer[] ::new);
// Print the converted array
System.out.println(Arrays.toString(integers));
}
}