How to Convert File to a Byte Array in Java
- Method 1: Using FileInputStream
- Method 2: Using Apache Commons IO
- Method 3: Using NIO (New Input/Output)
- Conclusion
- FAQ
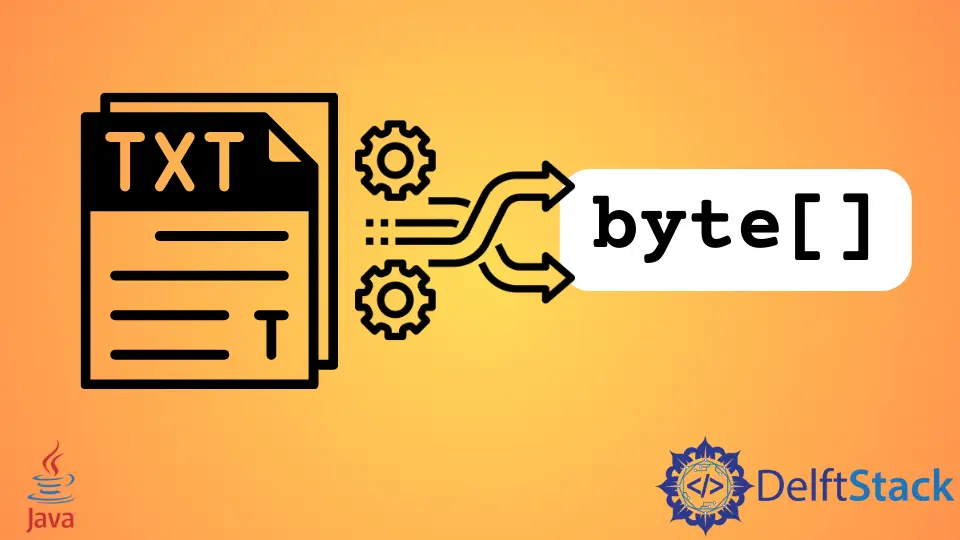
When working with files in Java, you might find yourself needing to convert a file into a byte array. This conversion is crucial for various applications, such as file uploads, data processing, or even when you need to manipulate file data in memory.
In this article, we will explore multiple methods to convert a file to a byte array in Java. Whether you’re a beginner or an experienced programmer, you’ll find these methods straightforward and easy to implement. So, let’s dive in and unlock the power of byte arrays in Java!
Method 1: Using FileInputStream
One of the most common ways to convert a file to a byte array in Java is by using the FileInputStream
class. This method is efficient and straightforward, making it a preferred choice for many developers.
Here’s how you can do it:
import java.io.File;
import java.io.FileInputStream;
import java.io.IOException;
public class FileToByteArray {
public static byte[] convertFileToByteArray(File file) throws IOException {
FileInputStream fileInputStream = new FileInputStream(file);
byte[] byteArray = new byte[(int) file.length()];
fileInputStream.read(byteArray);
fileInputStream.close();
return byteArray;
}
public static void main(String[] args) {
try {
File file = new File("example.txt");
byte[] byteArray = convertFileToByteArray(file);
System.out.println("File converted to byte array successfully.");
} catch (IOException e) {
System.out.println("Error: " + e.getMessage());
}
}
}
Output:
File converted to byte array successfully.
In this method, we first create a FileInputStream
object to read the file. We then allocate a byte array of the same size as the file. The read
method fills this byte array with the file’s contents. Finally, we close the stream to free up system resources. This approach is efficient for smaller files, but for larger files, you might want to consider alternative methods to avoid memory issues.
Method 2: Using Apache Commons IO
If you’re looking for a more robust solution, the Apache Commons IO library provides a convenient method to convert a file to a byte array. This library simplifies file handling and offers various utility methods that can save you time and effort.
Here’s how to use it:
import java.io.File;
import java.io.IOException;
import org.apache.commons.io.FileUtils;
public class FileToByteArray {
public static byte[] convertFileToByteArray(File file) throws IOException {
return FileUtils.readFileToByteArray(file);
}
public static void main(String[] args) {
try {
File file = new File("example.txt");
byte[] byteArray = convertFileToByteArray(file);
System.out.println("File converted to byte array successfully.");
} catch (IOException e) {
System.out.println("Error: " + e.getMessage());
}
}
}
Output:
File converted to byte array successfully.
In this method, we leverage the FileUtils.readFileToByteArray
method from the Apache Commons IO library. This method handles the reading process internally, making it less error-prone and more concise. It’s particularly useful for larger files, as it manages memory more effectively. Just remember to include the Apache Commons IO library in your project dependencies to use this method.
Method 3: Using NIO (New Input/Output)
Java NIO (New Input/Output) provides a modern approach to file handling and offers an efficient way to read files into byte arrays. This method is particularly advantageous for its non-blocking capabilities and better performance with larger files.
Here’s how to implement it:
import java.io.IOException;
import java.nio.file.Files;
import java.nio.file.Path;
public class FileToByteArray {
public static byte[] convertFileToByteArray(Path path) throws IOException {
return Files.readAllBytes(path);
}
public static void main(String[] args) {
try {
Path path = Path.of("example.txt");
byte[] byteArray = convertFileToByteArray(path);
System.out.println("File converted to byte array successfully.");
} catch (IOException e) {
System.out.println("Error: " + e.getMessage());
}
}
}
Output:
File converted to byte array successfully.
In this method, we use the Files.readAllBytes
method, which reads all bytes from the file at once. This approach is efficient and works well with large files, as it minimizes the number of I/O operations. Additionally, NIO provides better error handling and performance, making it a solid choice for file operations in modern Java applications.
Conclusion
Converting a file to a byte array in Java can be accomplished in several ways, each with its own advantages. Whether you choose to use FileInputStream
, the Apache Commons IO library, or Java NIO, understanding these methods will enhance your file handling capabilities in Java. Experiment with these techniques to find the one that best suits your needs, and you’ll be well on your way to mastering file manipulation in Java.
FAQ
- What is a byte array in Java?
A byte array in Java is an array that holds binary data and is often used for file handling, data transmission, and more.
-
Can I convert large files to byte arrays?
Yes, but be cautious with memory usage. Using NIO or Apache Commons IO is recommended for handling larger files efficiently. -
Do I need to close the FileInputStream?
Yes, it’s essential to close the FileInputStream to free up system resources and avoid memory leaks. -
Is Apache Commons IO necessary for file conversion?
No, it’s not necessary, but it simplifies file handling and provides additional utility methods. -
How does Java NIO improve file handling?
Java NIO offers non-blocking I/O operations, better performance, and improved error handling compared to traditional I/O methods.