How to Convert Char to Int in Java
Hassan Saeed
Feb 02, 2024
-
Use
Character.getNumericValue(ch)
to Convert achar
to anint
-
Subtract the Given
char
From0
in Java
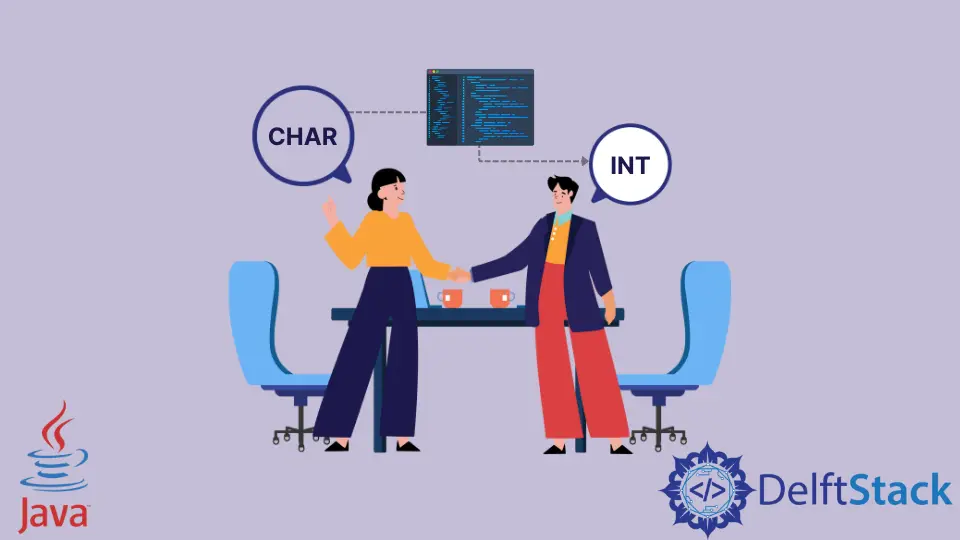
This tutorial discusses methods to convert a char
to an int
in Java.
Use Character.getNumericValue(ch)
to Convert a char
to an int
The simplest way to convert a char
to an int
in Java is to use the built-in function of the Character
class - Character.getNumericValue(ch)
. The below example illustrates this:
public class MyClass {
public static void main(String args[]) {
char myChar = '5';
int myInt = Character.getNumericValue(myChar);
System.out.println("Value after conversion to int: " + myInt);
}
}
Output:
Value after conversion to int: 5
Subtract the Given char
From 0
in Java
Another way to convert a char
to an int
is to subtract it from the char 0
. The below example illustrates this:
public class MyClass {
public static void main(String args[]) {
char myChar = '5';
int myInt = myChar - '0';
System.out.println("Value after conversion to int: " + myInt);
}
}
Output:
Value after conversion to int: 5
These are the two commonly used methods to convert a char
to an int
in Java. However, keep in mind that even if the given char
does not represent a valid digit, the above methods will not give any error. Consider the example below.
public class MyClass {
public static void main(String args[]) {
char myChar = 'A';
int myInt = myChar - '0';
System.out.println("Value after conversion to int: " + myInt);
}
}
Output:
Value after conversion to int: 17
Even though the input char
was an alphabet, the code ran successfully. Therefore, we must ensure that the input char
represents a valid digit.
Related Article - Java Int
- How to Convert Int to Char in Java
- How to Convert Int to Double in Java
- List of Ints in Java
- How to Convert Integer to Int in Java
- How to Check if Int Is Null in Java
- How to Convert Int to Byte in Java