How to Convert Byte Array to String in Java
Hassan Saeed
Feb 02, 2024
-
Use
new String()
to Convert a Byte Array to a String in Java -
Use
getBytes()
to Convert a String to a Byte Array in Java
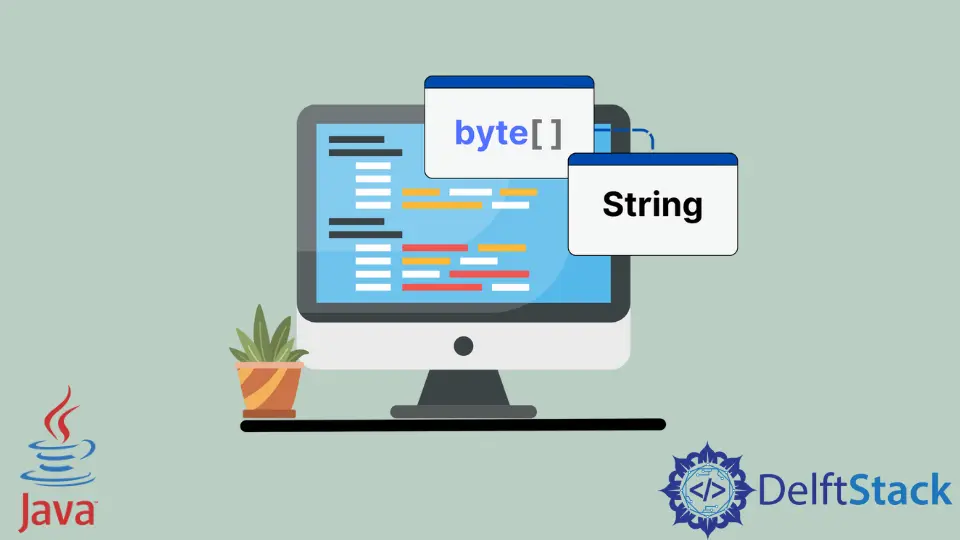
This tutorial discusses methods to convert a byte array to string in Java
Use new String()
to Convert a Byte Array to a String in Java
The simplest way to convert a byte array to a string in Java is to create a new string from the byte array with the encoding defined. The below example illustrates this:
import java.util.*;
public class MyClass {
public static void main(String args[]) {
byte[] bytes = {};
String str = new String(bytes, java.nio.charset.StandardCharsets.UTF_8);
}
}
We used StandardCharsets.UTF_8
in the above example. Depending on the encoding of our byte array, we will have to change it respectively.
Use getBytes()
to Convert a String to a Byte Array in Java
The simplest way to convert a string to a byte array in Java is by using getBytes()
. The below example illustrates this:
import java.util.*;
public class MyClass {
public static void main(String args[]) {
String str = "Sample text";
str.getBytes(java.nio.charset.StandardCharsets.UTF_8);
}
}