How to Convert an Array to a List in Java
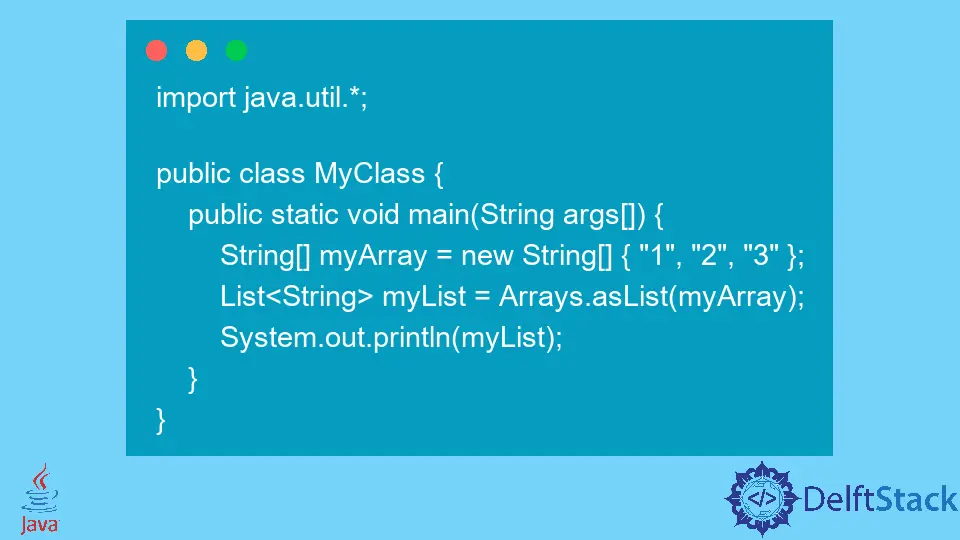
Converting an array to a list in Java is a common task that many developers encounter. Whether you are working with legacy code or modern applications, understanding how to make this conversion is essential for effective data manipulation. Java provides several methods to achieve this, each with its own advantages.
In this article, we will explore the most efficient ways to convert an array to a list in Java. From using the built-in Arrays.asList()
method to leveraging Java Streams, we will break down each approach with clear examples and explanations. By the end of this article, you will have a solid grasp of how to seamlessly transition between arrays and lists in your Java applications.
Using Arrays.asList() Method
The Arrays.asList()
method is one of the most straightforward ways to convert an array to a list in Java. This method takes an array as an argument and returns a fixed-size list backed by the specified array. This means that any changes made to the list will reflect in the original array and vice versa.
Here’s how to use it:
import java.util.Arrays;
import java.util.List;
public class ArrayToListExample {
public static void main(String[] args) {
String[] array = {"Apple", "Banana", "Cherry"};
List<String> list = Arrays.asList(array);
System.out.println("List: " + list);
}
}
Output:
List: [Apple, Banana, Cherry]
The Arrays.asList()
method is particularly useful when you need a quick conversion without the overhead of creating a new list. However, it’s important to note that the list returned is of fixed size. This means you cannot add or remove elements from it. If you need a resizable list, you can create a new ArrayList
from the fixed-size list.
Using ArrayList Constructor
If you require a dynamic list that allows for modifications, you can use the ArrayList
constructor that takes a collection as an argument. This approach creates a new ArrayList
which can be modified freely.
Here’s how you can do it:
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
public class ArrayToListExample {
public static void main(String[] args) {
String[] array = {"Apple", "Banana", "Cherry"};
List<String> list = new ArrayList<>(Arrays.asList(array));
list.add("Date");
System.out.println("List after adding an element: " + list);
}
}
Output:
List after adding an element: [Apple, Banana, Cherry, Date]
In this example, we first convert the array to a fixed-size list using Arrays.asList()
. We then pass that list to the ArrayList
constructor, creating a new list that we can modify. This method is particularly useful when you need to perform operations like adding or removing elements from the list without constraints.
Using Java Streams
Java 8 introduced the Stream API, which provides a powerful way to handle collections and arrays. You can use streams to convert an array to a list in a more functional style. This method is particularly handy when you want to perform additional operations during the conversion, such as filtering or mapping.
Here’s an example:
import java.util.Arrays;
import java.util.List;
import java.util.stream.Collectors;
public class ArrayToListExample {
public static void main(String[] args) {
String[] array = {"Apple", "Banana", "Cherry"};
List<String> list = Arrays.stream(array)
.collect(Collectors.toList());
System.out.println("List using streams: " + list);
}
}
Output:
List using streams: [Apple, Banana, Cherry]
In this example, we create a stream from the array and then collect the elements into a list using Collectors.toList()
. This method is highly flexible and allows you to easily apply transformations, such as filtering or mapping, during the conversion process. If you are working with larger datasets or need to perform complex operations, using streams can greatly enhance the readability and maintainability of your code.
Conclusion
Converting an array to a list in Java is a fundamental skill that can enhance your coding efficiency. Whether you choose the simple Arrays.asList()
method for quick conversions, the ArrayList
constructor for dynamic lists, or the Stream API for more complex operations, each method has its unique advantages. By mastering these techniques, you can manipulate data more effectively and write cleaner, more efficient Java code. Don’t hesitate to experiment with these methods in your own projects to see which one fits best for your specific needs.
FAQ
-
What is the difference between
Arrays.asList()
andArrayList
?
Arrays.asList()
returns a fixed-size list, whileArrayList
allows for dynamic resizing. -
Can I modify the list created by
Arrays.asList()
?
No, the list returned byArrays.asList()
is fixed-size and does not support adding or removing elements. -
How can I convert a primitive array to a list in Java?
You can convert a primitive array to a list by using the wrapper classes, such asInteger[]
forint[]
, and then usingArrays.asList()
. -
Is using Java Streams for conversion slower than the other methods?
Generally, using streams may have a slight performance overhead due to the additional processing, but it provides more flexibility and readability. -
Can I convert a multi-dimensional array to a list?
Yes, but you will need to flatten the array first, asArrays.asList()
does not handle multi-dimensional arrays directly.
Related Article - Java Array
- How to Concatenate Two Arrays in Java
- How to Convert Byte Array in Hex String in Java
- How to Remove Duplicates From Array in Java
- How to Count Repeated Elements in an Array in Java
- Natural Ordering in Java
- How to Slice an Array in Java