How to Convert a String to Char in Java
Hassan Saeed
Feb 02, 2024
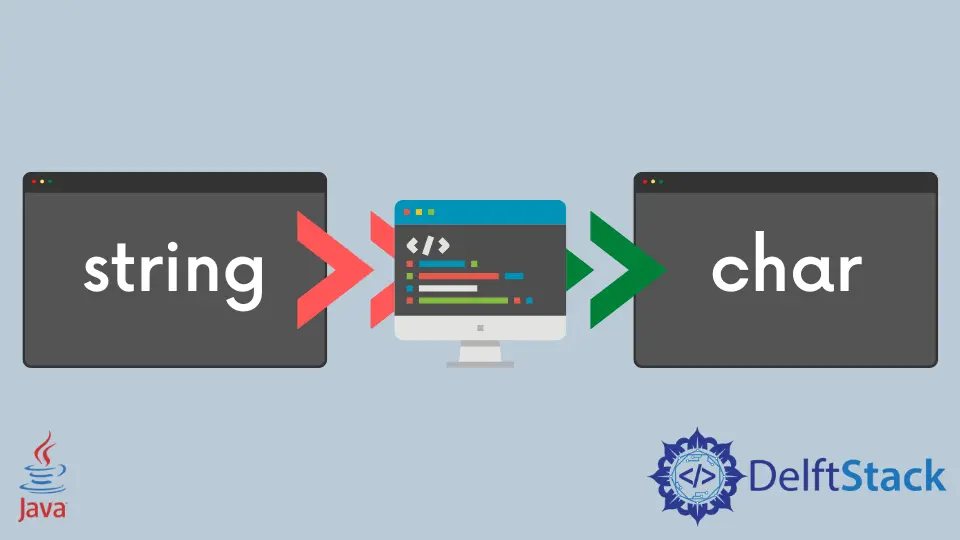
This tutorial discusses methods to convert a string to a char in Java.
charAt()
to Convert String to Char in Java
The simplest way to convert a character from a String
to a char
is using the charAt(index)
method. This method takes an integer as input and returns the character on the given index in the String
as a char
.
The below example illustrates this:
public class MyClass {
public static void main(String args[]) {
String myString = "string";
char myChar = myString.charAt(0);
System.out.println(myChar);
}
}
Output:
s
However, if you try to input an integer greater than the length of the String
, it will throw an error.
The below example illustrates this:
public class MyClass {
public static void main(String args[]) {
String myString = "string";
char myChar = myString.charAt(6);
System.out.println(myChar);
}
}
Output:
Exception in thread "main" java.lang.StringIndexOutOfBoundsException: String index out of range: 6
at java.base/java.lang.StringLatin1.charAt(StringLatin1.java:47)
at java.base/java.lang.String.charAt(String.java:693)
at MyClass.main(MyClass.java:4)
toCharArray()
to Convert String to Char in Java
We can use this method if we want to convert the whole string to a character array.
The below example illustrates this:
public class MyClass {
public static void main(String args[]) {
String myString = "string";
char[] myChars = myString.toCharArray();
for (int i = 0; i < myChars.length; i++) {
System.out.println(myChars[i]);
}
}
}
Output:
s
t
r
i
n
g
If we want to access a char
at a specific location, we can simply use myChars[index]
to get the char
at the specified location.
Related Article - Java Char
- How to Convert Int to Char in Java
- Char vs String in Java
- How to Initialize Char in Java
- How to Represent Empty Char in Java
- How to Convert Char to Uppercase/Lowercase in Java
- How to Check if a Character Is Alphanumeric in Java