How to Convert a Char to a String in Java
- Using String.valueOf() Method
- Using Character.toString() Method
- Using String Concatenation
- Using String Constructor
- Conclusion
- FAQ
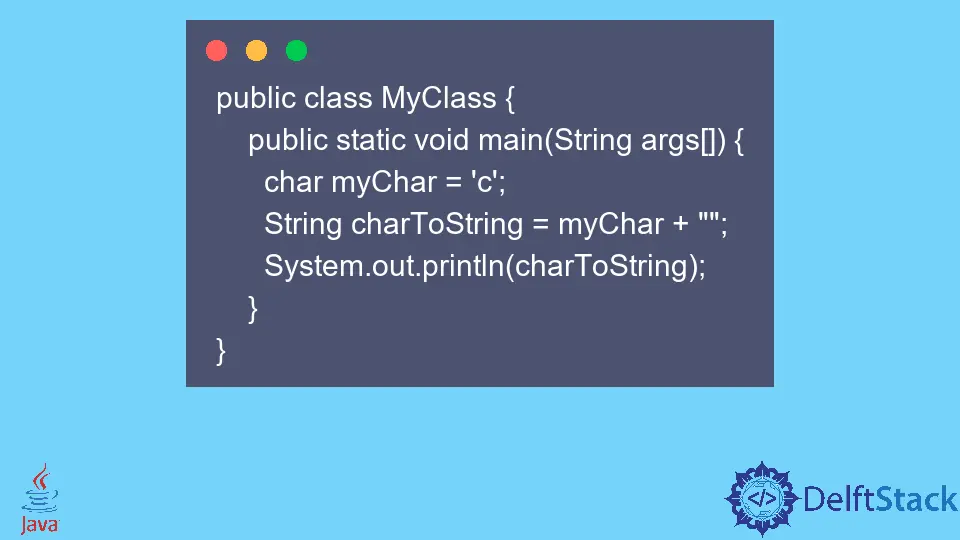
When working with Java, you may find yourself needing to convert a character (char) to a string. This is a common task that can arise in various programming scenarios, from simple string manipulations to more complex data handling. Fortunately, Java provides several straightforward methods to achieve this conversion.
In this article, we will explore multiple ways to convert a char to a String in Java, ensuring you have a solid understanding of each method. Whether you are a beginner or an experienced developer, these techniques will enhance your coding toolkit and streamline your workflow. Let’s dive in!
Using String.valueOf() Method
One of the simplest and most effective ways to convert a char to a String in Java is by using the String.valueOf()
method. This method is versatile and can handle various data types, including characters. By passing a single character to this method, you can easily obtain its string representation.
Here’s how you can do it:
char character = 'A';
String stringValue = String.valueOf(character);
System.out.println(stringValue);
Output:
A
The String.valueOf()
method takes the character as an argument and returns a new string that contains the character. This method is particularly useful because it can also convert other data types, making it a handy tool in your Java programming arsenal. It’s worth noting that this method is static, meaning you don’t need to create an instance of the String
class to use it.
Using Character.toString() Method
Another effective way to convert a char to a String in Java is by using the Character.toString()
method. This method is specifically designed to convert a character into its string representation, making it a clear and concise option for this task.
Here’s a simple example:
char character = 'B';
String stringValue = Character.toString(character);
System.out.println(stringValue);
Output:
B
The Character.toString()
method works similarly to String.valueOf()
, but it is specifically tailored for characters. This can make your code more readable, especially if you want to explicitly indicate that you are working with a character. The method returns a string that represents the specified character, allowing you to use it seamlessly in your applications.
Using String Concatenation
String concatenation is another straightforward method to convert a char to a String in Java. By concatenating the character with an empty string, you can easily create a string representation of the char. This method is simple and effective, making it a popular choice among Java developers.
Here’s how you can implement this:
char character = 'C';
String stringValue = character + "";
System.out.println(stringValue);
Output:
C
In this example, we concatenate the character with an empty string. The result is a new string that contains the character. This method is intuitive and can be easily understood by anyone reading your code. However, while it is effective, it may not be as clear as using the dedicated conversion methods, especially for those who are new to Java.
Using String Constructor
Lastly, you can also convert a char to a String using the String
constructor. This method allows you to create a new string from an array of characters, and since a single char can be placed into a character array, it serves as a viable option for conversion.
Here’s an example of how to do this:
char character = 'D';
String stringValue = new String(new char[]{character});
System.out.println(stringValue);
Output:
D
In this approach, we create a new character array containing the single character and then pass that array to the String
constructor. The constructor then returns a new string that represents the character. While this method is slightly more verbose than the previous ones, it demonstrates the flexibility of Java’s string handling capabilities.
Conclusion
Converting a char to a String in Java is a fundamental skill that every Java developer should master. Whether you choose to use String.valueOf()
, Character.toString()
, string concatenation, or the String
constructor, each method has its advantages and can be applied in different scenarios. By understanding these techniques, you can write cleaner, more efficient code, and handle string manipulations with ease. So, the next time you find yourself needing to perform this conversion, you’ll have the knowledge at your fingertips to do it seamlessly.
FAQ
-
What is the easiest way to convert a char to a String in Java?
The easiest way is to use the String.valueOf() method. -
Can I use string concatenation to convert a char to a String?
Yes, you can concatenate a char with an empty string to achieve the conversion. -
Is there a specific method for converting characters in Java?
Yes, the Character.toString() method is specifically designed for this purpose. -
What is the advantage of using the String constructor for conversion?
It allows you to create a string from an array of characters, offering flexibility. -
Are there performance differences between these methods?
Generally, the performance differences are negligible for small-scale conversions, but dedicated methods like String.valueOf() may be slightly more efficient.
Related Article - Java String
- How to Perform String to String Array Conversion in Java
- How to Remove Substring From String in Java
- How to Convert Byte Array in Hex String in Java
- How to Convert Java String Into Byte
- How to Generate Random String in Java
- The Swap Method in Java