How to Check if a String Is Empty or Null in Java
Hassan Saeed
Feb 02, 2024
-
Use
str == null
to Check if a String Isnull
in Java -
Use
str.isEmpty()
to Check if a String Is Empty in Java
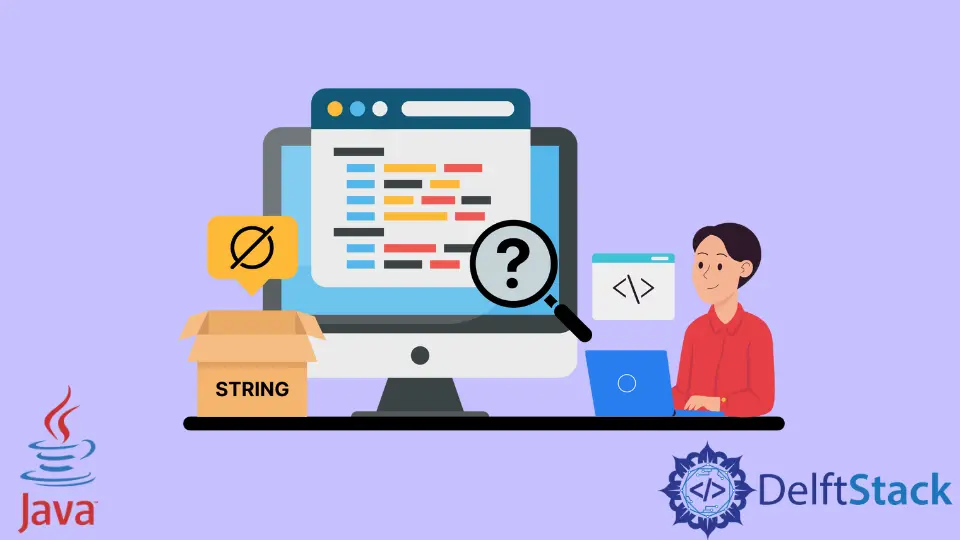
This tutorial discusses methods to check if a string is empty or null in Java.
Use str == null
to Check if a String Is null
in Java
The simplest way to check if a given string is null
in Java is to compare it with null
using str == null
. The below example illustrates this:
public class MyClass {
public static void main(String args[]) {
String str1 = null;
String str2 = "Some text";
if (str1 == null)
System.out.println("str1 is a null string");
else
System.out.println("str1 is not a null string");
if (str2 == null)
System.out.println("str2 is a null string");
else
System.out.println("str2 is not a null string");
}
}
Output:
str1 is a null string
str2 is not a null string
Use str.isEmpty()
to Check if a String Is Empty in Java
The simplest way to check if a given string is empty in Java is to use the built-in method of String
class - isEmpty()
. The below example illustrates this:
public class MyClass {
public static void main(String args[]) {
String str1 = "";
String str2 = "Some text";
if (str1.isEmpty())
System.out.println("str1 is an empty string");
else
System.out.println("str1 is not an empty string");
if (str2.isEmpty())
System.out.println("str2 is an empty string");
else
System.out.println("str2 is not an empty string");
}
}
Output:
str1 is an empty string
str2 is not an empty string
If we are interested in checking for both of the conditions at the same time, we can do so by using logical OR
operator - ||
. The below example illustrates this:
public class MyClass {
public static void main(String args[]) {
String str1 = "";
if (str1.isEmpty() || str1 == null)
System.out.println("This is an empty or null string");
else
System.out.println("This is neither empty nor null string");
}
}
Output:
This is an empty or null string