GC Overhead Limit Exceeded Error in Java
- Brief Introduction to GC Overhead Limit Exceeded Error in Java
- Resolve the GC Overhead Limit Exceeded Error in Java
- Conclusion
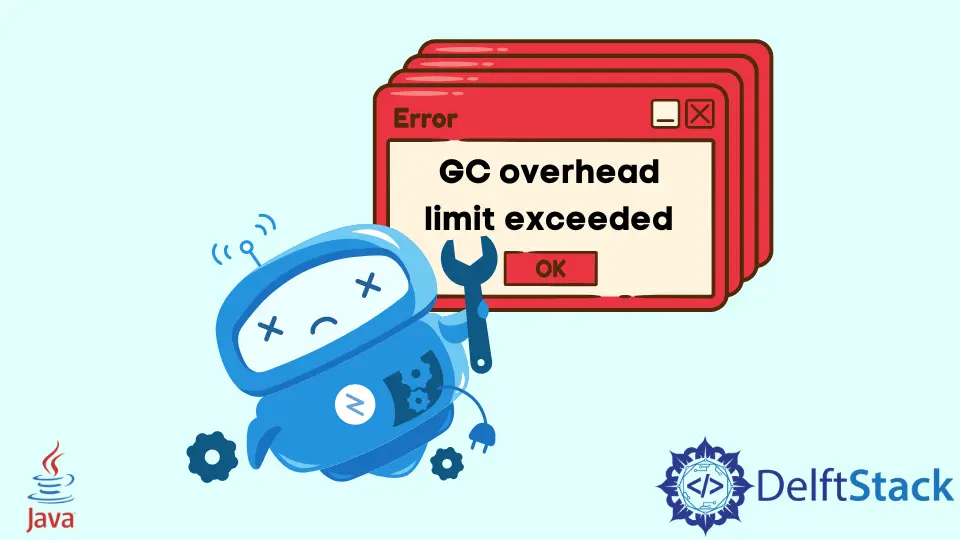
This article will discuss the GC overhead limit exceeded
error in Java.
Brief Introduction to GC Overhead Limit Exceeded Error in Java
In Java, JVM (Java Virtual Machine) frees the memory when the program no longer uses the objects. The process of freeing up memory is called Garbage Collection (GC).
The GC overhead limit exceeded
error shows that resources/memory is getting exhausted. This error falls under java.lang.OutOfMemoryError
family of errors.
As per official Java documentation, JVM (Java Virtual Machine) throws this error to indicate that the program or the application is spending more time in garbage collection than doing any valuable work, i.e., when 98% of the time is spent in garbage collection by the application.
Now, let’s see an example to understand it better.
import java.util.*;
public class test {
public static void main(String args[]) throws Exception {
Map<Integer, String> map = new HashMap<>();
Random rnd = new Random();
while (true) {
map.put(rnd.nextInt(), "val");
}
catch (Exception e) {
System.out.println("ye");
}
}
}
We have created a map
in the above code and inserted values infinitely. A note point to remember is that maps
use heap memory.
And we execute the code with a parallel garbage collector to get GC overhead limit exceeded error
.
java - Xmx100m - XX : +UseParallelGC test
When the above code is executed with the parallel garbage collector, we will get the java.lang.OutOfMemoryError: GC overhead limit exceeded
message. But, this may differ from computer to computer due to Java heap size or a different GC algorithm.
Resolve the GC Overhead Limit Exceeded Error in Java
The idea here is to stop memory leaks in our application. We can be cautious by following the below points to avoid this error in our program.
- Identify the location of objects where memory allocation in a heap is done.
- Avoid weakly-referenced objects and the huge amount of temporary objects as they increase the probability of memory leakage.
- Identify the objects which occupy a large amount of space in a heap.
Conclusion
In this article, we learned about the GC overhead limit exceeded
error which occurs when a program spends more time in garbage collection than a useful task. We understood that this could be prevented by keeping a good check at the positions where heap memory is allocated to objects so that memory leaks could be avoided, thus preventing the program from getting this error.
Related Article - Java Error
- How to Fix the Error: Failed to Create the Java Virtual Machine
- How to Fix the Missing Server JVM Error in Java
- How to Fix the 'No Java Virtual Machine Was Found' Error in Eclipse
- How to Fix Javax.Net.SSL.SSLHandShakeException: Remote Host Closed Connection During Handshake
- How to Fix the Error: Failed to Create the Java Virtual Machine
- How to Fix Java.Lang.VerifyError: Bad Type on Operand Stack