How to Find the Day of the Week Using Zellers Congruence in Java
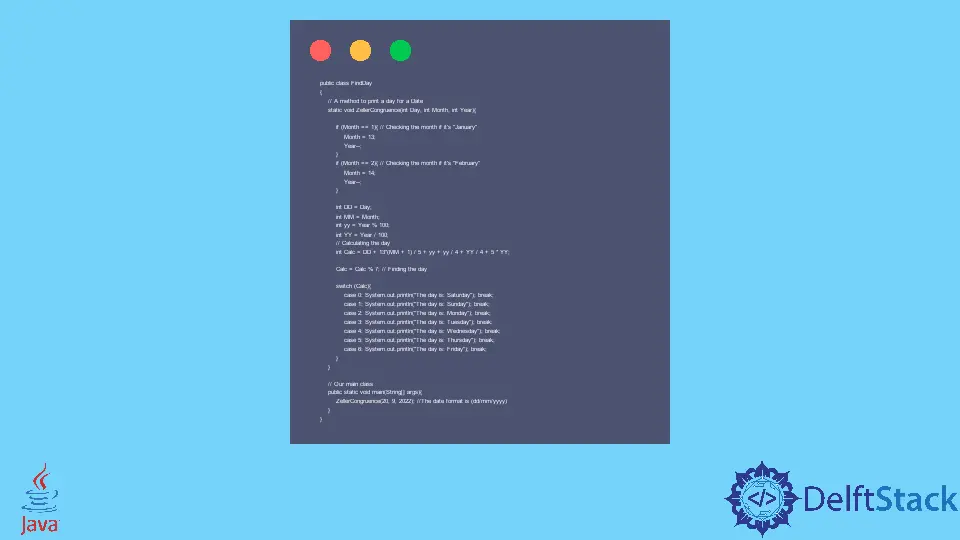
This article shows how we can use Java to implement Zeller’s congruence to find the day of the week. Also, we’ll look at an example with line by line explanation to make the topic easier.
Find the Day of the Week Using Zeller’s Congruence in Java
Remember, the algorithm we are using in this article is to count the month January
as 13
and the month February
as 14
of the previous year.
For example, if a date is 13 January 2009
, the algorithm will count it as the 13th month of 2008
. In the following code fence, we will demonstrate how to find the day for a week.
Example Code:
public class FindDay {
// A method to print a day for a Date
static void ZellerCongruence(int Day, int Month, int Year) {
if (Month == 1) { // Checking the month if it's "January"
Month = 13;
Year--;
}
if (Month == 2) { // Checking the month if it's "February"
Month = 14;
Year--;
}
int DD = Day;
int MM = Month;
int yy = Year % 100;
int YY = Year / 100;
// Calculating the day
int Calc = DD + 13 * (MM + 1) / 5 + yy + yy / 4 + YY / 4 + 5 * YY;
Calc = Calc % 7; // Finding the day
switch (Calc) {
case 0:
System.out.println("The day is: Saturday");
break;
case 1:
System.out.println("The day is: Sunday");
break;
case 2:
System.out.println("The day is: Monday");
break;
case 3:
System.out.println("The day is: Tuesday");
break;
case 4:
System.out.println("The day is: Wednesday");
break;
case 5:
System.out.println("The day is: Thursday");
break;
case 6:
System.out.println("The day is: Friday");
break;
}
}
// Our main class
public static void main(String[] args) {
ZellerCongruence(20, 9, 2022); // The date format is (dd/mm/yyyy)
}
}
We have already described the purpose of each line. Therefore, executing the above example code will get the below output in your console.
The day is: Tuesday
Aminul Is an Expert Technical Writer and Full-Stack Developer. He has hands-on working experience on numerous Developer Platforms and SAAS startups. He is highly skilled in numerous Programming languages and Frameworks. He can write professional technical articles like Reviews, Programming, Documentation, SOP, User manual, Whitepaper, etc.
LinkedIn