Final Class in Java
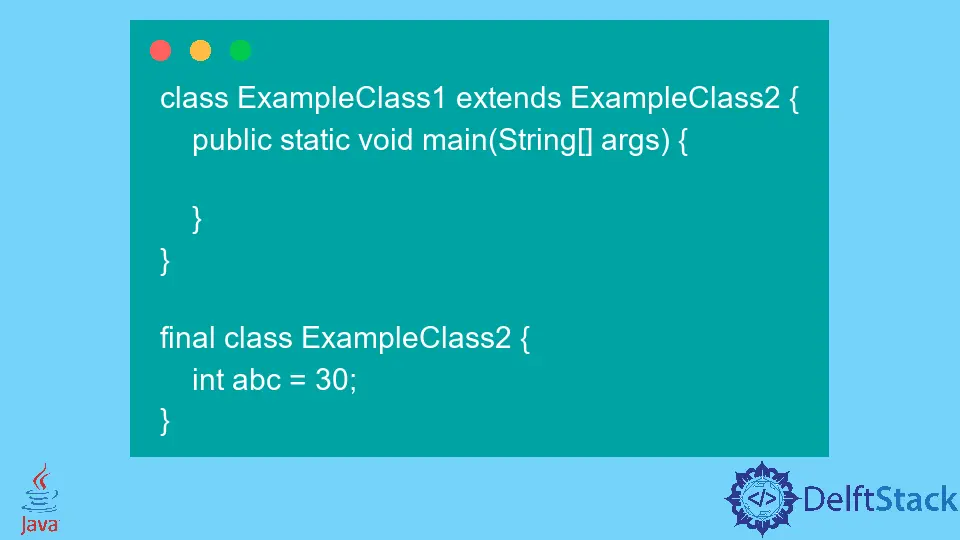
This tutorial goes through the topic of the final
class with an example. The final
is a keyword in Java that can be used in different contexts with variables, methods, and classes.
It restricts access in every context to some extent. We will discuss the final class.
Use the final
Class to Restrict the Class in Java
A class with the keyword final
is called a final class. We use the final
keyword with a class to restrict the class to be inherited by other classes.
We make the class final when we want to secure any extensions. One of its advantages is security because a final class is immutable, which cannot be changed.
In the below example, we have two classes. The first-class ExampleClass1
has the main()
method, while in the second class ExampleClass2
, we use the final
keyword to make it a final class.
When we extend the ExampleClass2
using the extends
keyword in ExampleClass1
, we get an error in the output that says that we cannot inherit a final class.
class ExampleClass1 extends ExampleClass2 {
public static void main(String[] args) {}
}
final class ExampleClass2 { int abc = 30; }
Output:
java: cannot inherit from final com.tutorial.ExampleClass2
We cannot extend a final class, but what if we want to access a property or method of the class?
Note that the class cannot be extended when using the final
keyword, but we can create its object to access its functions and variables.
There are two classes in the example; one is the final class ExampleClass2
while another class ExampleClass1
is needed for the main()
method to execute.
We create an object of the ExampleClass2
class and then print the value of abc
, an integer variable.
We can access the class and its variable even when final because we do not use inheritance here.
class ExampleClass1 {
public static void main(String[] args) {
ExampleClass2 exampleClass2 = new ExampleClass2();
System.out.println(exampleClass2.abc);
}
}
final class ExampleClass2 { int abc = 30; }
Output:
30
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedIn