How to Fill a 2D Array in Java
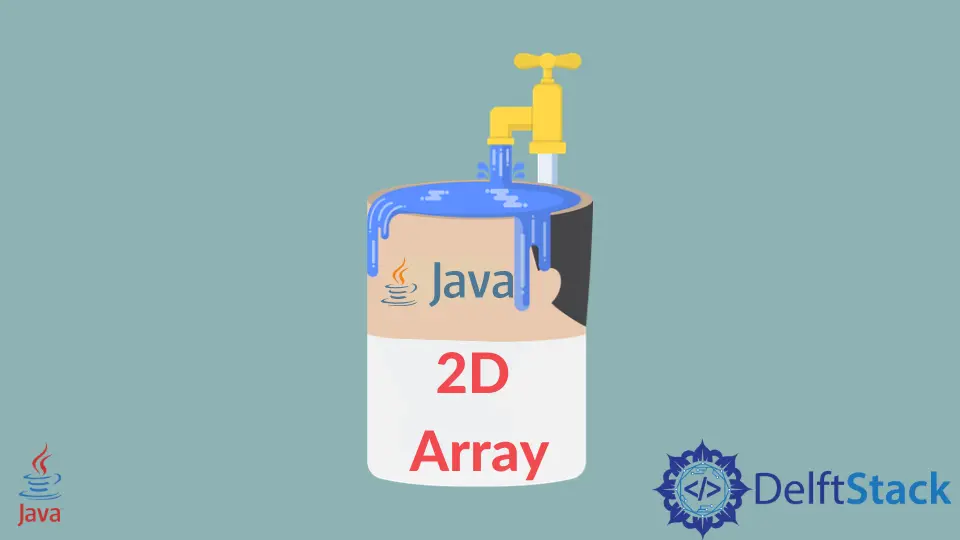
The 2D array is based on a table structure which means rows and columns, and filling the 2d array cannot be done with simple adding to array operation. This tutorial demonstrates how to fill a 2d array in Java.
Fill a 2D Array in Java
The arrays in Java are zero-based, which means the range of the array is 0
to the array.Length – 1
. To fill the 2D array, we have to start the filling from the index 0 – 0
.
We need to use two-dimensional loops to fill a 2d array. The example below demonstrates how to fill a 2d array in Java.
Code Example:
package delftstack;
import java.util.Scanner;
public class Fill_Array {
public static void main(String[] args) {
System.out.print("Number of rows for 2d array: ");
Scanner input = new Scanner(System.in);
int Row = input.nextInt();
System.out.print("Number of columns for 2d array: ");
int Column = input.nextInt();
// 2d array declaration
int[][] Demo_Array = new int[Row][Column];
for (int x = 0; x < Row; x++) {
for (int y = 0; y < Column; y++) {
System.out.print(String.format("Enter the array member at Demo_Array[%d][%d] : ", x, y));
Demo_Array[x][y] = input.nextInt(); // add the member to specific index
}
}
// Print the 2d Array
for (int x = 0; x < Demo_Array.length; x++) {
for (int y = 0; y < Demo_Array[0].length; y++) {
System.out.print(Demo_Array[x][y] + "\t");
}
System.out.println();
}
// close the scanner object
input.close();
}
}
The code above will ask to input the number of rows and columns first, and then it asks for the array member at each index.
Output:
Number of rows for 2d array: 3
Number of columns for 2d array: 3
Enter the array member at Demo_Array[0][0] : 1
Enter the array member at Demo_Array[0][1] : 2
Enter the array member at Demo_Array[0][2] : 4
Enter the array member at Demo_Array[1][0] : 5
Enter the array member at Demo_Array[1][1] : 6
Enter the array member at Demo_Array[1][2] : 7
Enter the array member at Demo_Array[2][0] : 8
Enter the array member at Demo_Array[2][1] : 9
Enter the array member at Demo_Array[2][2] : 10
1 2 4
5 6 7
8 9 10
We used a two-dimensional loop to fill the array. It can also be done manually using the Demo_Array[0][0] = number;
syntax, but this can be a long way to fill the array.
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook