Extends Comparable in Java
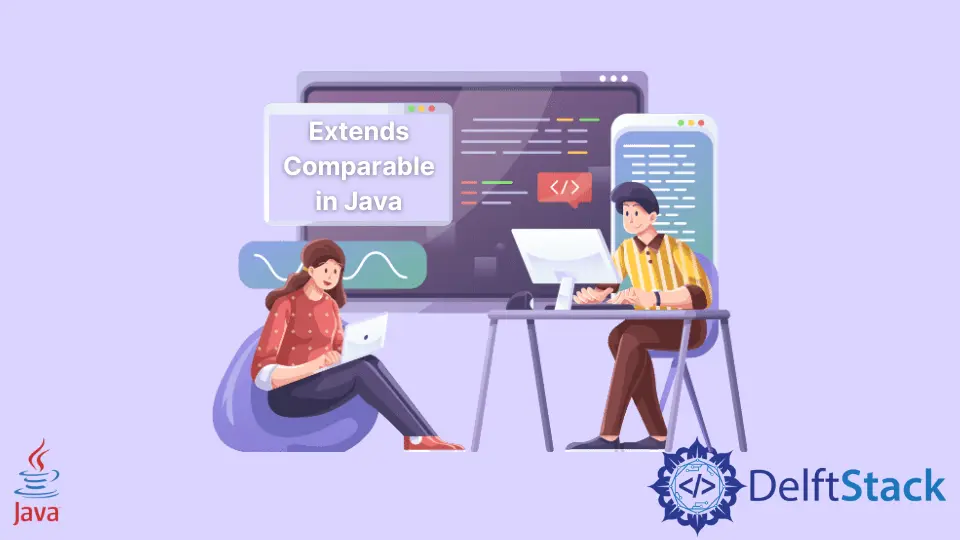
This guide will learn about the Extends Comparable Interface in Java. It is written as Comparable<T>
. It is an interface that is implemented by many classes in Java. Let’s learn more about its aspects.
Implementing the Extends Comparable<T>
Interface in Java
This Interface has only one method, compareTo(Object o)
. This method compares the object with the specified object for the order.
It returns a negative integer if the object is less than specified. It will return zero if the object and the specified object are equal.
Similarly, it returns a positive integer if the object is greater than the specified object.
Keep in mind that a class can’t extend the Java interface in Java.
The Interface can only extend the Interface. The Java class can only extend a Java class.
As Comparable<T>
is an interface in Java, we must create a custom interface that will extend the Comparable
Interface. The custom class will implement the custom interface.
public class Main {
public static void main(String[] args) {
Student s1 = new Student("Bill Gates");
Student s2 = new Student("James");
int res = s1.compareTo(s2);
// comaprison of name of two students using iherited and extended method of
// compareable.
System.out.println(res);
}
}
interface CustomComparable extends Comparable<Student> {
// Custom interface which extends Comparable.
// So CustomComparable have inherited method Compareto(Object o)
public String getName();
}
class Student implements CustomComparable {
// calss student implements CustomCompareable
private String name; // String variable
Student(String s) {
this.name = s;
}
public int compareTo(Student other) { // overiding super method........... .
return this.name.compareTo(other.getName());
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
}
Output:
-8
We are simply creating two student class objects that implement our custom comparator class, extending the actual Comparable<T>
. So, that’s how we can use this compareTo()
method here.
Haider specializes in technical writing. He has a solid background in computer science that allows him to create engaging, original, and compelling technical tutorials. In his free time, he enjoys adding new skills to his repertoire and watching Netflix.
LinkedIn