How to Fix Error: Class, Interface, or Enum Expected in Java
- Error on Extra Curly Braces After the Class Definition in Java
- Error on Function Definition After Class Definition in Java
- Extra Brace While Defining Enums in Java
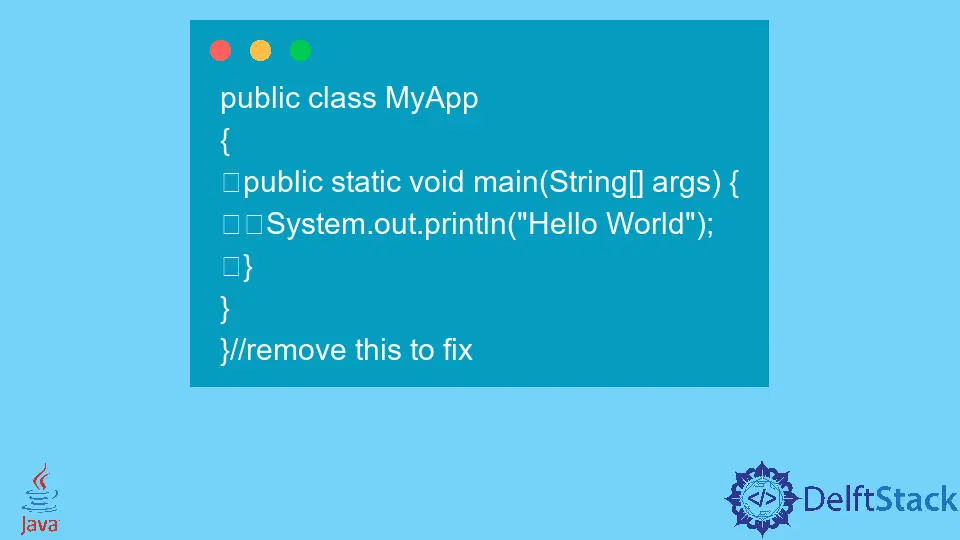
Java is an object-oriented, strongly typed and compiled language and has the concept of classes to leverage different aspects of programming like inheritance and polymorphism. This article will demonstrate the error: class, interface, or enum expected
compile time error.
Error on Extra Curly Braces After the Class Definition in Java
Consider the below code sample, where an extra brace has been deliberately added to the last line of the code sample.
public class MyApp {
public static void main(String[] args) {
System.out.println("Hello World");
}
}
} // remove this to fix
Here is the following error for the above code sample on the code compilation.
MyApp.java:7: error: class, interface, or enum expected
}
^
1 error
Error on Function Definition After Class Definition in Java
Consider the following code sample where an extra function is defined deliberately after the class definition.
public class MyApp {
public static void main(String[] args) {
System.out.println("Hello World");
}
}
public int add(int a) {
int b = a + 5;
return b;
} // move this function (add) inside the MyApp class to fix
Here is the error obtained when this code sample is compiled.
MyApp.java:8: error: class, interface, or enum expected
public int add(int a) {
^
MyApp.java:10: error: class, interface, or enum expected
return b;
^
MyApp.java:11: error: class, interface, or enum expected
}
^
3 errors
Extra Brace While Defining Enums in Java
Consider the code sample, which shows an enum
in Java with an extra brace at the end.
public enum Day { SUNDAY, MONDAY, TUESDAY, WEDNESDAY, THURSDAY, FRIDAY, SATURDAY }
} // remove this to fix
Here is the error obtained when the code sample is compiled.
Day.java:5: error: class, interface, or enum expected
}
^
1 error
Related Article - Java Error
- How to Fix the Error: Failed to Create the Java Virtual Machine
- How to Fix the Missing Server JVM Error in Java
- How to Fix the 'No Java Virtual Machine Was Found' Error in Eclipse
- How to Fix Javax.Net.SSL.SSLHandShakeException: Remote Host Closed Connection During Handshake
- How to Fix the Error: Failed to Create the Java Virtual Machine
- How to Fix Java.Lang.VerifyError: Bad Type on Operand Stack