How to Create An Empty Array in Java
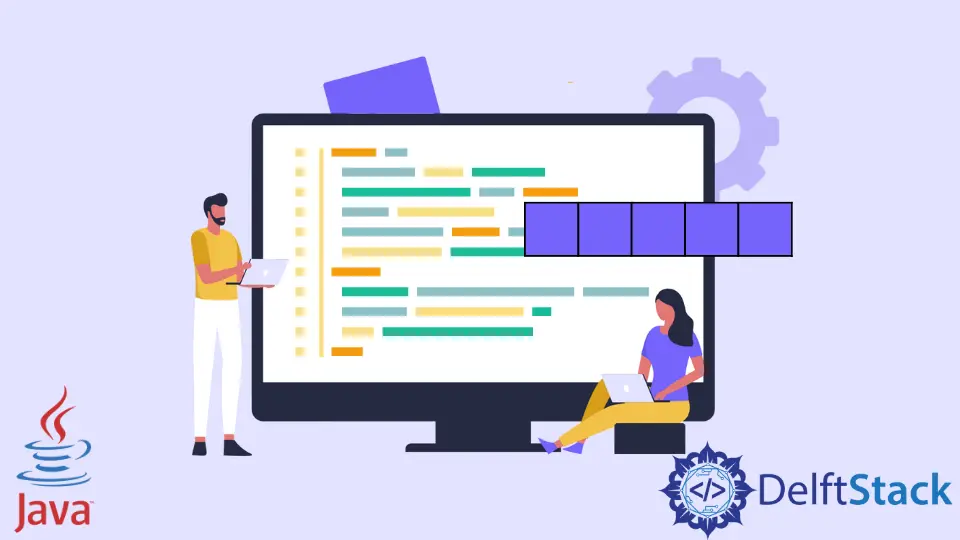
This tutorial introduces the difference between a null array and an empty array in Java and also lists some example codes to understand the topic.
An array that points to null reference is called null array in Java whereas an array that does not have null reference but initialized to default values is called an empty array. Although these are not standard terms but more technical.
In Java, array is an object and if we only declare an array then this object points to a null reference in the memory. A typical array declaration looks like: int[] arr;
.
The array creation is combination of both declaration and initialization (also refer as creation) so if we only declare array without initialization then the array would be called a null array, and an array that is declared and initialized by default values would be called empty array. A typical array creation is like: int[] arr = new int[5];
.
Let’s understand and take a close look at some examples.
Create Empty Array in Java
As we already discussed an array which is created and initialized with default values by the compiler is known as empty array. The default values depend on the type of array. For example, default value for integer array is 0 and and 0.0 for the float type.
Let’s take an example, where we are creating an integer type array. This array will hold default values. Let’s check by printing the array.
public class SimpleTesting {
public static void main(String[] args) {
int[] arr = new int[10];
System.out.println(arr[0]);
}
}
Output:
0
Null Array in Java
In this example, we are creating an array that holds null value. Basically an array that is declared only also holds null. So, be careful if the array is null because accessing its elements will raise an exception. See the example below.
public class SimpleTesting {
public static void main(String[] args) {
int[] arr = null;
System.out.println(arr[0]); // null pointer exception
}
}
Output:
Exception in thread "main" java.lang.NullPointerException
NullPointerException Handling in Java Array
In this example, we are handling exception that occurs if array is not created.
public class SimpleTesting {
public static void main(String[] args) {
try {
int[] arr = null;
System.out.println(arr[0]); // null pointer exception
} catch (Exception e) {
System.out.println("Array is Null");
}
}
}
Output:
Array is Null