What Is a Driver Class in Java
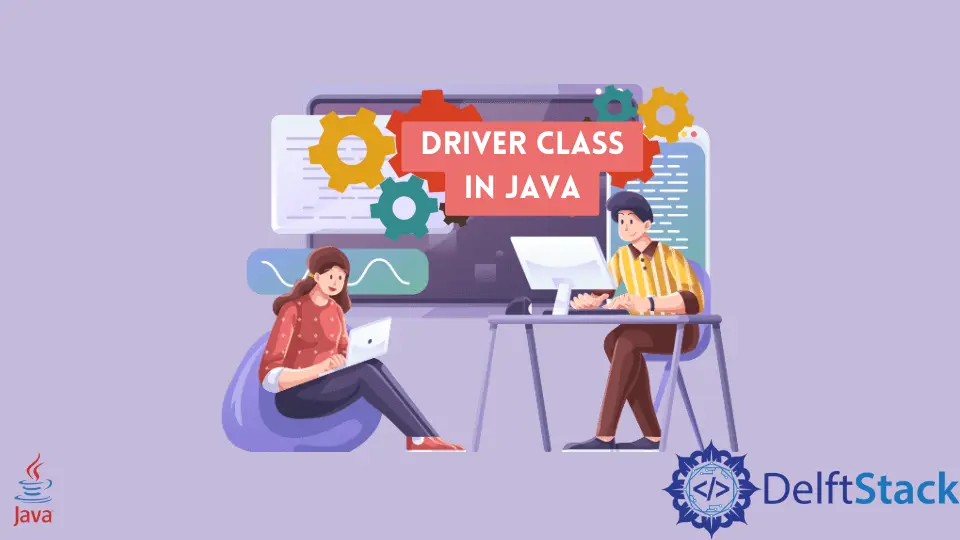
This tutorial introduces what is driver class in Java and how to use it in Java, and lists some example codes to understand the topic.
Driver classes are the utility classes that are used to carry out some task. In Java, driver classes are used in JDBC to connect a Java application to a database. Driver classes are vendor-specific i. e. MySQL database provides its own driver class, and Oracle database provides its own class as well.
So, if we want to connect a Java application with a MySQL database, we need to use the driver class provided by MySQL, and we will have to do the same for other databases as well.
To get driver class, we can refer to the official site and then download JARs. Later we can use these JARs in our Java application to connect the application with the database. For example, the OracleDriver
class is used for the Oracle database and the Driver
class for MySQL.
- Driver class for Oracle
oracle.jdbc.driver.OracleDriver
- Driver class for MySQL
com.mysql.jdbc.Driver
After getting the JARs, to load the Driver class in the Java application, Java provides a Class
class that has a forName()
method. This method is used to load the driver class.
The Class.forName()
method is used to load the class for connectivity.
Class.forName("oracle.jdbc.driver.OracleDriver");
Class.forName("com.mysql.jdbc.Driver");
MySQL Driver Class Example in Java
In this example, we used com.mysql.jdbc.Driver
class to connect to the MySQL database. We used JDBC API and its other class, such as DriverManager
, to establish the connection.
import java.sql.*;
public class SimpleTesting {
public static void main(String args[]) {
try {
Class.forName("com.mysql.jdbc.Driver");
Connection con = DriverManager.getConnection(
"jdbc:mysql://localhost:3306/dbname", "username", "userpassword");
Statement stmt = con.createStatement();
ResultSet rs = stmt.executeQuery("select * from mytable");
while (rs.next())
System.out.println(rs.getInt(1) + " " + rs.getString(2) + " " + rs.getString(3));
con.close();
} catch (Exception e) {
System.out.println(e);
}
}
}