How to Draw Rectangle in JavaFX
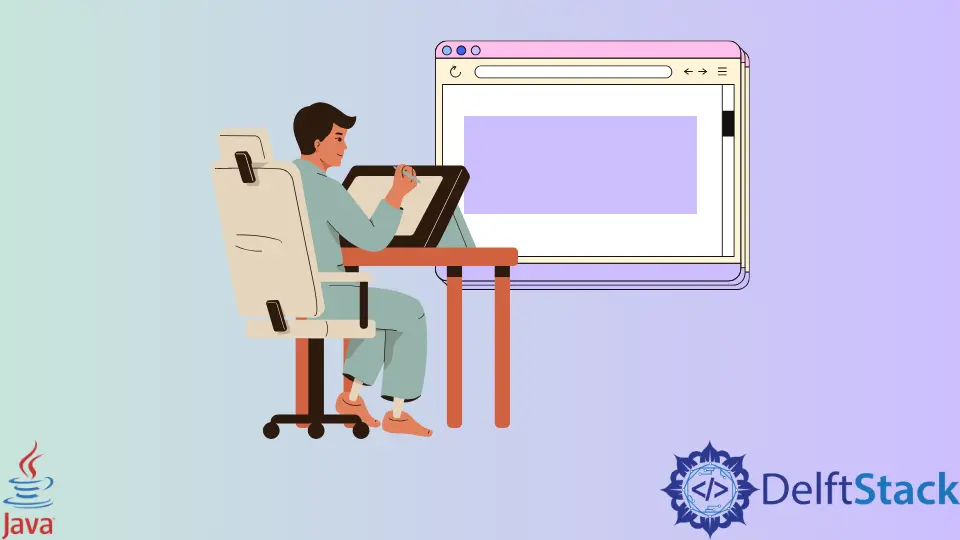
This tutorial demonstrates how to draw a rectangle in JavaFX.
Draw Rectangle in JavaFX
A closed polygon with four edges where the angle between two edges is right angle and sides at opposite are concurrent is called a rectangle. The rectangle can be defined by its width and height and the lengths of horizontal and vertical sides, respectively.
JavaFX package has a class named JavaFX.scene.shape.Rectangle
which can be used to generate rectangles in Java. This class has six properties that are used to generate a rectangle.
Property | Method | Description |
---|---|---|
height |
setHeight(Double height) |
Used to set the height of the rectangle. |
width |
setWidth(Double width) |
Used to set the width of the rectangle. |
ArcHeight |
setArcHeight(Double height) |
Used to set the vertical diameter of the arc at the four corners of the rectangle. |
ArcWidth |
setArcWidth(Double Width) |
Used to set the horizontal diameter of the arc at the four corners of the rectangle. |
x |
setX(Double X-value) |
Used to set the x coordinate of the upper left corner. |
y |
set(Double( Y-value) |
Used to set the y coordinate of the upper left corner. |
As we know about the properties now, let’s try an example to draw a rectangle without the arc.
-
First of all, instantiate the class
Rectangle
. -
Set the parameters of the rectangle using the
height
,width
,x
, andy
properties. -
Add the created rectangle to the root group object.
-
Launch the Application.
Code:
package delftstack;
import javafx.application.Application;
import javafx.scene.Group;
import javafx.scene.Scene;
import javafx.scene.paint.Color;
import javafx.scene.shape.Rectangle;
import javafx.stage.Stage;
public class Example extends Application {
public void start(Stage Demo_Stage) {
// Draw a Rectangle
Rectangle rectangle = new Rectangle();
// Set the properties of the rectangle
rectangle.setX(200.0f);
rectangle.setY(100.0f);
rectangle.setWidth(400.0f);
rectangle.setHeight(200.0f);
// Set other properties
rectangle.setFill(Color.BLUE);
rectangle.setStrokeWidth(8.0);
rectangle.setStroke(Color.LIGHTBLUE);
// Set the Scene
Group Demo_Root = new Group(rectangle);
Scene Demo_Scene = new Scene(Demo_Root, 800, 400, Color.BEIGE);
Demo_Stage.setTitle("Draw Rectangle");
Demo_Stage.setScene(Demo_Scene);
Demo_Stage.show();
}
public static void main(String args[]) {
launch(args);
}
}
The code above will create a rectangle with given parameters.
Output:
Let’s try to use ArcWidth
and ArcHeight
to create a rounded rectangle.
-
Follow all the steps described above.
-
Add the arc parameters using
ArcWidth
, andArcHeight
properties.
Code:
package delftstack;
import javafx.application.Application;
import javafx.scene.Group;
import javafx.scene.Scene;
import javafx.scene.paint.Color;
import javafx.scene.shape.Rectangle;
import javafx.stage.Stage;
public class Example extends Application {
public void start(Stage Demo_Stage) {
// Draw a Rectangle
Rectangle rectangle = new Rectangle();
// Set the properties of the rectangle
rectangle.setX(200.0f);
rectangle.setY(100.0f);
rectangle.setWidth(400.0f);
rectangle.setHeight(200.0f);
rectangle.setArcHeight(40.0);
rectangle.setArcWidth(40.0);
// Set other properties
rectangle.setFill(Color.BLUE);
rectangle.setStrokeWidth(8.0);
rectangle.setStroke(Color.LIGHTBLUE);
// Set the Scene
Group Demo_Root = new Group(rectangle);
Scene Demo_Scene = new Scene(Demo_Root, 800, 400, Color.BEIGE);
Demo_Stage.setTitle("Draw Rounded Rectangle");
Demo_Stage.setScene(Demo_Scene);
Demo_Stage.show();
}
public static void main(String args[]) {
launch(args);
}
}
As shown in the code above, we set the arc parameters. The rectangle will be rounded.
Output:
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook