Double Division in Java
- Understanding Integer Division
- Understanding Double Division
- Mixing Integer and Double Division
- Tips for Avoiding Division Confusion
- Conclusion
- FAQ
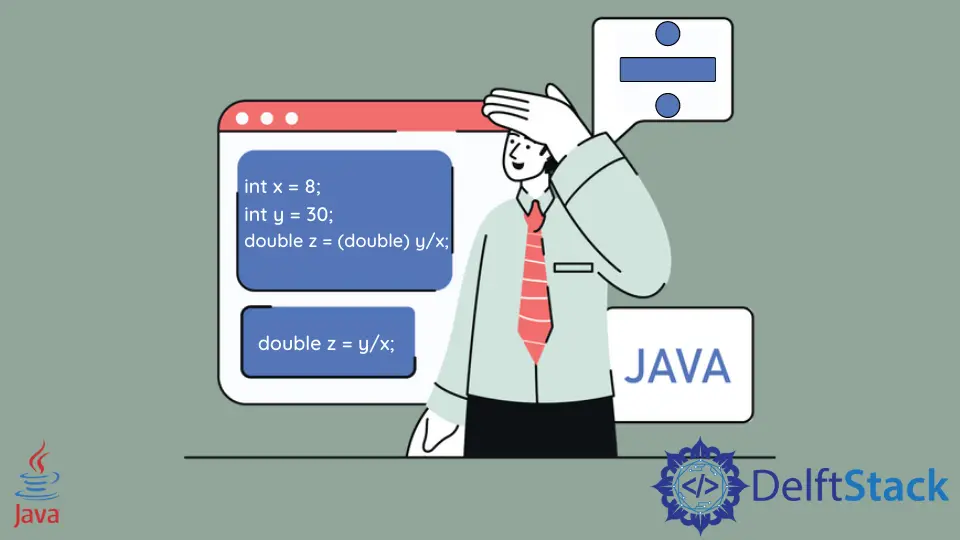
In the world of programming, particularly in Java, understanding how different data types interact during division operations is crucial. One common source of confusion arises from the differences between integer division and double division. Integer division truncates the decimal, while double division retains it, leading to potential pitfalls if not properly understood.
This article will clarify these concepts, helping you navigate the intricacies of division in Java. We will explore the nuances of integer and double division, illustrate with code examples, and provide clear explanations to enhance your understanding. Whether you’re a beginner or looking to refresh your knowledge, this guide will equip you with the insights needed to avoid common mistakes in division operations.
Understanding Integer Division
When you perform division with two integers in Java, the result is also an integer. This means that any fractional part is discarded. For example, dividing 5 by 2 will yield 2, not 2.5. This behavior can lead to unexpected results if you are not aware of it. Here’s a simple code example to illustrate this concept:
public class IntegerDivision {
public static void main(String[] args) {
int a = 5;
int b = 2;
int result = a / b;
System.out.println("Integer Division Result: " + result);
}
}
Output:
Integer Division Result: 2
In this code, we define two integers, a
and b
, and perform division. The output shows that the result is 2, which is the truncated value of the actual division. This behavior can be misleading, especially for those new to Java. Understanding this is essential to avoid errors in calculations, especially when precision is required.
Understanding Double Division
On the other hand, double division occurs when at least one operand is a double (or float). In this case, Java performs floating-point division, which retains the decimal portion of the result. This can be particularly useful when you need precise calculations. Let’s look at an example:
public class DoubleDivision {
public static void main(String[] args) {
double a = 5;
double b = 2;
double result = a / b;
System.out.println("Double Division Result: " + result);
}
}
Output:
Double Division Result: 2.5
In this example, both a
and b
are defined as doubles. As a result, the division operation retains the decimal, yielding a result of 2.5. This distinction is vital for performing accurate calculations in Java. Remember, if you want to achieve double division, ensure that at least one of the operands is a double.
Mixing Integer and Double Division
A common scenario developers face is mixing integers and doubles in division. When you divide an integer by a double, Java automatically promotes the integer to a double for the operation. This means you will receive a double result, which can sometimes lead to confusion. Let’s see how this works:
public class MixedDivision {
public static void main(String[] args) {
int a = 5;
double b = 2.0;
double result = a / b;
System.out.println("Mixed Division Result: " + result);
}
}
Output:
Mixed Division Result: 2.5
In this code, a
is an integer, and b
is a double. The division operation promotes a
to a double, resulting in a final output of 2.5. Understanding this behavior is crucial for ensuring that you get the expected results in your calculations. Always be mindful of your data types when performing division in Java.
Tips for Avoiding Division Confusion
To avoid confusion when working with division in Java, consider the following tips:
-
Explicit Casting: If you want to ensure a double result from integer division, explicitly cast one of the integers to double. For example,
double result = (double) a / b;
will give you the expected result. -
Use Descriptive Variable Names: Naming your variables descriptively can help you keep track of their types, reducing the likelihood of errors during division.
-
Test with Different Data Types: Experiment with integer and double combinations to see how Java handles them. This hands-on approach can solidify your understanding.
By following these tips, you can navigate Java’s division operations with confidence and clarity.
Conclusion
In summary, understanding the differences between integer and double division in Java is essential for any developer. Integer division truncates decimal points, while double division retains them, leading to different outcomes based on the data types used. By being aware of these distinctions and following best practices, you can avoid common pitfalls and ensure accurate calculations in your Java applications. Whether you are a novice or an experienced programmer, mastering these concepts will enhance your coding skills and confidence in handling division operations effectively.
FAQ
-
What happens when I divide two integers in Java?
The result is an integer, and any decimal portion is truncated. -
How can I ensure a double result when dividing integers?
You can cast one of the integers to double before performing the division. -
Is there any difference between float and double in Java?
Yes, float is a single-precision 32-bit IEEE 754 floating point, while double is a double-precision 64-bit IEEE 754 floating point. -
Can I mix integer and double types in division?
Yes, when an integer is divided by a double, the integer is promoted to double, and the result will be a double. -
Why is understanding division important in Java?
Understanding division helps avoid errors in calculations, especially in applications requiring precision.
Haider specializes in technical writing. He has a solid background in computer science that allows him to create engaging, original, and compelling technical tutorials. In his free time, he enjoys adding new skills to his repertoire and watching Netflix.
LinkedIn