Difference Between void and Void in Java
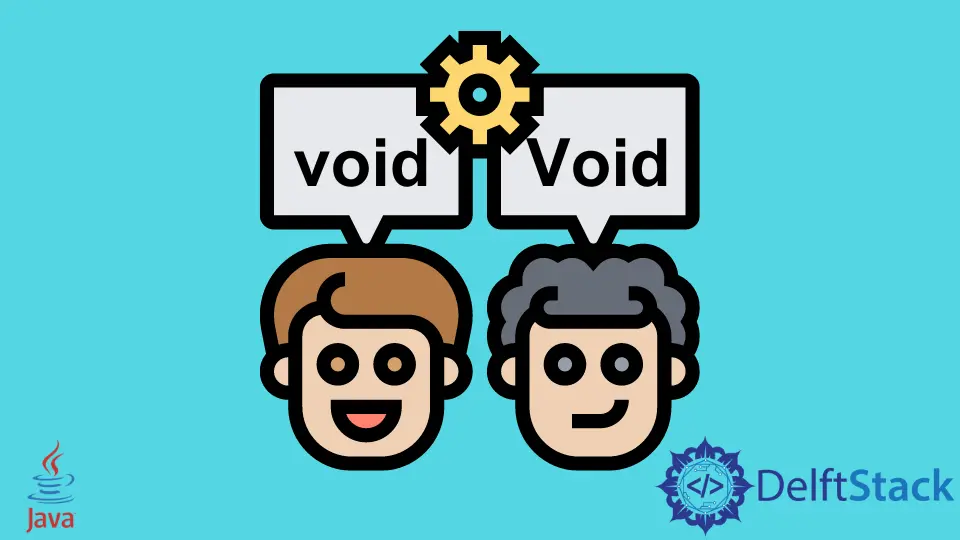
The java.lang.Void
type is analogous to the java.lang.Integer
. The Void
wraps around void
and extends the object class, and the void
does not have any possible values, making the java.lang.Void
un-instantiable.
We aim to find and understand the difference between Void
and void
in Java programming. We will also learn how and where we can use Void
or void
in Java programming.
Difference Between void
and Void
in Java
The basic difference between both (Void
& void
) is that void
is a primitive type while Void
, a reference type that inherits from the Object
. Although none of them has any possible values, both are of different types.
The void
(all lowercase) is the improper type used to refer to the value’s absence. Due to not having any denotable value, void
means no value will be returned by a function or method.
On the other hand, java.lang.Void
returns the object, we can return null
to do things legally, and it is the only way to do it. It is not instantiable because it has a private constructor that can not be accessed outside.
Use the void
and Void
in Java
We use void
(all lowercase) where we want a method to not return any value. Like in the following example, the printMessage()
method does not return anything but prints a message on the screen.
Example Code for void
(Main.java
):
public class Main {
static void printMessage() {
System.out.println("printMessage function just got executed!");
}
public static void main(String[] args) {
printMessage();
}
}
We can use Void
(capitalize the first letter) while doing reflection in Java programming because there are some situations where we need to present the void
keyword as the object.
At the same time, we are not allowed to create the object of the Void
class, and that’s why its constructor is private and can not be accessed from outside.
Moreover, we can’t inherit the Void
class because it is a final
class. This leads us to use Void
in reflection, where we get the return type of a method as void
.
Example Code for Void
(Main.java
):
public class Main {
public static void main(String[] args) throws SecurityException, NoSuchMethodException {
Class c1 = TestOne.class.getMethod("Test", (Class<?>[]) null).getReturnType();
System.out.println(c1 == Void.TYPE); // true
System.out.println(c1 == Void.class); // false
}
}
Example Code for Void
(TestOne.java
):
class TestOne {
public void Test() {}
}
Output:
True
False
We can also get the return type of a method as void
with generics. We want to write the code for a generic class that can return void for something.
abstract class Foo<T> {
abstract T bar();
}
class Bar extends Foo<Void> {
Void bar() {
return (null);
}
}
Output:
True
False