Difference Between & and && in Java
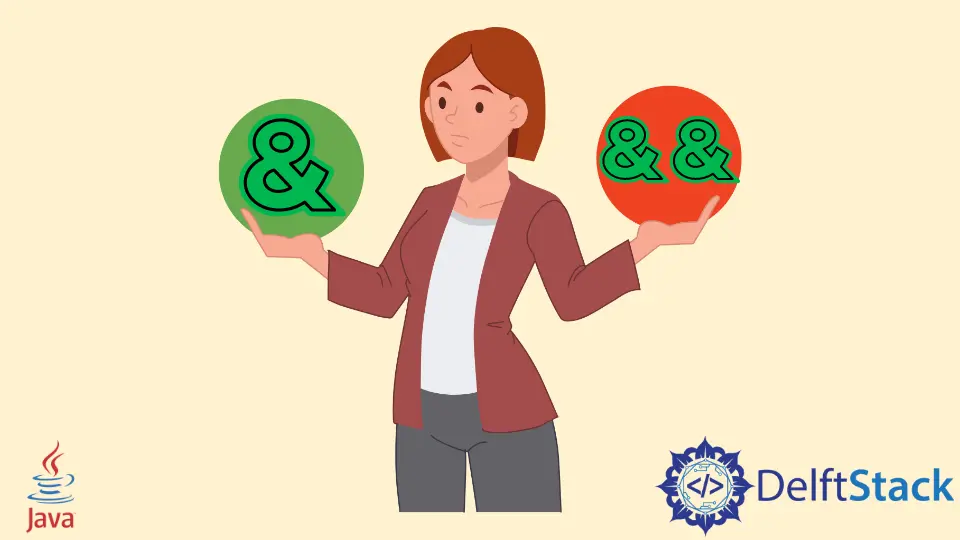
An operator in Java is a symbol that can perform some desired operation on a set of values. Java has different types of operator types like logical, arithmetic, bitwise, and more.
In this tutorial, we will try to study and understand the difference between &
and &&
operator in Java.
The &
operator is a bitwise operator. Bitwise operators are basically used for working and manipulating individual bits of the numbers. It operates on both sides of the operator. Bitwise &
operator is a binary operator which performs the AND operation bit by bit on its operands.
The following code demonstrates the use of &
operator.
public class operators {
public static void main(String[] args) {
int a = 5;
int b = 7;
System.out.println("a&b = " + (a & b)); // 0101 & 0111=0101 = 5
}
}
Output:
a&b = 5
The &&
is the logical AND
operator which works with boolean operands. As the name suggests, logical operators can perform the logical operation and combine two or more conditions. These can be used with any form of data type.
The logical &&
operator returns true when both the conditions are true. It evaluates the operands from left to the right. It converts each operand into a boolean value and returns the original value after operating.
The following code demonstrates the && operator.
import java.io.*;
class Logical_Operator {
public static void main(String[] args) {
int a = 10, b = 20, c = 20 System.out.println("Var1 = " + a);
System.out.println("Var2 = " + b);
System.out.println("Var3 = " + c);
if ((a < b) && (b == c)) {
System.out.println("True Conditions");
} else
System.out.println("False conditions");
}
}
Output:
A = 10
B = 20
C = 20
True Conditions
In the above example, both the conditions are true. That is why True Conditions
is printed. Even if one of them were false, then False Conditions
would have been published.