Dice Game in Java
Lovey Arora
Oct 12, 2023
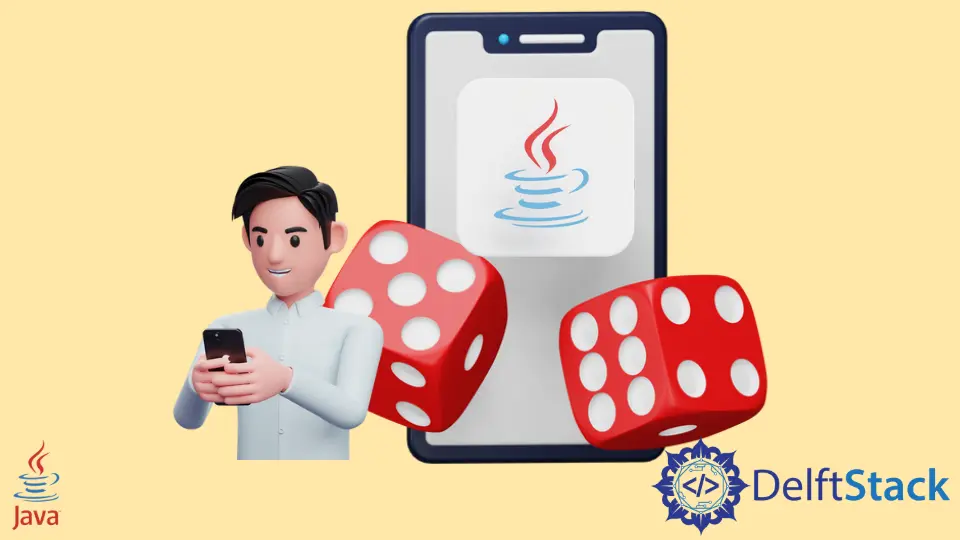
This tutorial will demonstrate a program to create a simple dice game in Java.
For this, we will use the java.util.Random
package to generate random numbers between 1 and 6 that will represent the numbers on the dice. In our example, we will emulate the N dice roller. We will throw N number of dies whose result we will add and print.
We can use this program as a base to design various other dice games.
See the code given below.
import java.util.Random;
import java.util.Scanner;
public class Main {
public static void main(String args[]) {
System.out.print("Enter the number of dice: ");
Scanner input = new Scanner(System.in);
int numberOfDice = input.nextInt();
Random ranNum = new Random();
System.out.print("Hey Coder! You rolled: ");
int total = 0;
int randomNumber = 0;
for (int i = 0; i < numberOfDice; i++) {
randomNumber = ranNum.nextInt(6) + 1;
total = total + randomNumber;
System.out.print(randomNumber);
System.out.print(" ");
}
System.out.println("");
System.out.println("Total: " + total);
input.close();
}
}
Output:
Enter the number of dice: 6
Hey Coder! You rolled: 2 6 3 2 2 4
Total: 19
In the above example, we ask the user to input the total number of dice. Since the user entered 6, we generated 6 random numbers between 1 and 6 to represent the result of each dice. We added the result for each observation and printed the result.
We can generate more complicated games by using this as a base.