Destructor in Java
- What is a Destructor?
- Memory Management in Java
- Finalization in Java
- Best Practices for Resource Management in Java
- Conclusion
- FAQ
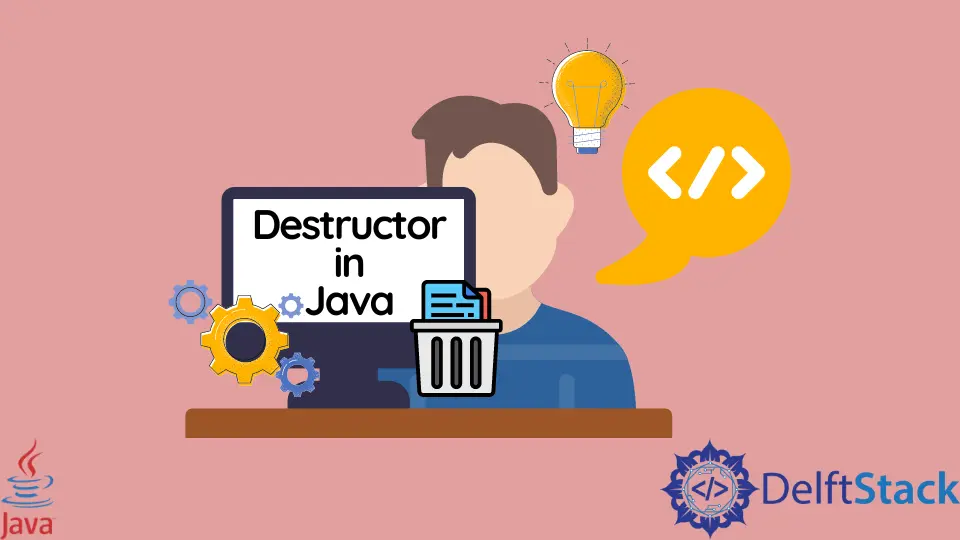
In this tutorial, we will discuss destructors in Java, a topic that often sparks confusion among both novice and experienced programmers. While many languages like C++ have explicit destructors, Java approaches resource management differently, primarily through its garbage collection mechanism. Understanding how Java handles object destruction is crucial for efficient memory management and overall application performance.
This article will delve into the concept of destructors, clarify common misconceptions, and explore how Java manages object lifecycle and memory cleanup. By the end, you’ll have a solid grasp of the topic, enabling you to write more efficient and effective Java code.
What is a Destructor?
In programming languages like C++, a destructor is a special method invoked when an object goes out of scope or is explicitly deleted. Its primary role is to free up resources that the object may be holding, such as memory or file handles. However, Java does not have a direct equivalent of destructors. Instead, it relies on a garbage collector, a built-in feature that automatically manages memory, freeing up space when objects are no longer in use.
Java’s garbage collector periodically scans for objects that are no longer reachable from any references in the program. Once identified, the garbage collector reclaims the memory, thus eliminating the need for explicit destructors. This automated memory management simplifies development but requires a good understanding of how Java handles object lifecycle to avoid memory leaks and ensure optimal performance.
Memory Management in Java
Java’s memory management is primarily handled through its garbage collection mechanism. This process is automatic, which means that developers do not need to manually allocate and deallocate memory. However, understanding how memory management works is essential for writing efficient Java applications.
When you create an object in Java, memory is allocated for that object on the heap. The garbage collector keeps track of these objects and their references. If an object is no longer referenced, it becomes eligible for garbage collection. This means that the memory occupied by the object can be reclaimed, preventing memory leaks.
Java provides several garbage collection algorithms, such as Mark-and-Sweep, Generational Garbage Collection, and G1 Garbage Collector. Each has its advantages and is suitable for different types of applications. By understanding these algorithms, you can write Java code that is not only efficient but also minimizes the risk of memory-related issues.
Finalization in Java
While Java does not have destructors, it offers a mechanism called finalization. The finalize()
method can be overridden in your class to perform cleanup actions before the object is garbage collected. However, relying on finalization is generally discouraged due to its unpredictability and performance overhead.
Here’s a simple example of how to use the finalize()
method:
class MyClass {
@Override
protected void finalize() throws Throwable {
try {
System.out.println("Cleaning up resources...");
} finally {
super.finalize();
}
}
}
public class Main {
public static void main(String[] args) {
MyClass obj = new MyClass();
obj = null; // Make the object eligible for garbage collection
System.gc(); // Request garbage collection
}
}
Output:
Cleaning up resources...
In this example, the finalize()
method is overridden to print a message when the object is about to be garbage collected. After setting the object reference to null
, we call System.gc()
to suggest that the garbage collector run. However, it’s important to note that there is no guarantee when or if the finalize()
method will be called, making it an unreliable method for resource management.
Best Practices for Resource Management in Java
To effectively manage resources in Java without relying on destructors or finalization, it’s crucial to follow best practices. One of the most effective methods is to use the try-with-resources statement, which automatically closes resources like files or database connections when done.
Here’s how you can implement it:
import java.io.BufferedReader;
import java.io.FileReader;
import java.io.IOException;
public class FileReadExample {
public static void main(String[] args) {
try (BufferedReader br = new BufferedReader(new FileReader("file.txt"))) {
String line;
while ((line = br.readLine()) != null) {
System.out.println(line);
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
Output:
Content of file.txt
In this code snippet, the try-with-resources
statement ensures that the BufferedReader
is closed automatically, even if an exception occurs. This approach eliminates the need for a finalizer and provides a clean way to manage resources effectively.
Conclusion
In summary, while Java does not have destructors in the traditional sense, it offers a robust garbage collection mechanism that simplifies memory management. Understanding how Java handles object lifecycle and memory cleanup is essential for writing efficient code. By utilizing features like the finalize()
method and the try-with-resources statement, developers can ensure proper resource management without the need for explicit destructors. This knowledge will not only improve your Java applications but also enhance your overall programming skills.
FAQ
-
What is a destructor in programming?
A destructor is a special method used in some programming languages to free resources when an object is no longer needed. -
Does Java have destructors?
No, Java does not have destructors. It uses a garbage collection mechanism to manage memory automatically. -
What is the purpose of the finalize() method in Java?
Thefinalize()
method allows you to perform cleanup actions before an object is garbage collected, but its use is discouraged due to unpredictability. -
How does garbage collection work in Java?
Java’s garbage collector automatically identifies and reclaims memory occupied by objects that are no longer reachable from any references. -
What is the try-with-resources statement?
The try-with-resources statement in Java ensures that resources are closed automatically when the try block is exited, preventing resource leaks.