How to Define a Static Method in Java Interface
-
static
Method in Javainterface
-
Importance of Using
static
Methods in aninterface
-
Rules for
static
Methods in aninterface
-
Reasons for Not Having
static
Methods in Interfaces Before Java 8 -
Reasons for Not Being Able to Override a
static
Method
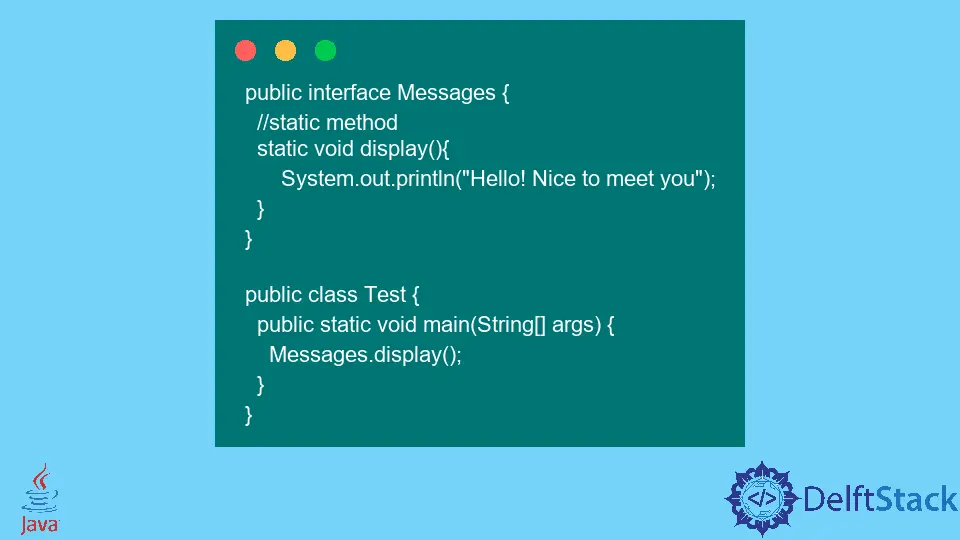
This tutorial lists the rules for having static
methods in Java interface
and demonstrates how to define them and why we can’t override them. We’ll also explore th reasons for not having static
methods in interfaces before Java 8.
static
Method in Java interface
Before going into the static
methods in Java interfaces, it is important to know about an interface
, which contains one or multiple abstract
methods (the methods without body) defined in the class that implements
this interface
.
Note that these abstract
methods are public
methods whether we write the public
modifier or not.
Example Code:
public interface Messages {
// public & abstract
public void display();
}
public class Test implements Messages {
// implementation of abstract method
public void display() {
System.out.println("Hi! Welcome to the DelftStack");
}
public static void main(String[] args) {
Test test = new Test();
test.display();
}
}
Output:
Hi! Welcome to the DelftStack
Remember, the abstract
method named display()
is a public
method, we have to instantiate the Test
class to execute display()
method.
On the other hand, the static
method is associated with the class itself in which they are defined, and we don’t need to instantiate the class to use these static
methods. As of Java 8, we can now have static
interface methods.
Now, we have a complete body and all the required instructions for that specific static
method in the interface
. Remember that the static
methods can only be accessed at the class level, not the instance level.
So, we can access the static
methods as follows.
Example Code:
public interface Messages {
// static method
static void display() {
System.out.println("Hello! Nice to meet you");
}
}
public class Test {
public static void main(String[] args) {
Messages.display();
}
}
Output:
Hello! Nice to meet you
The question is can we override a static
method? No, we can’t override a static
method. Otherwise, we will get a compilation error.
Additionally, we can not call them via an instance of an implementing class since the static
methods are hidden. See the following example producing a compilation error.
Example Code:
public interface Messages {
static void display() {
System.out.println("Hello! Nice to meet you");
}
}
public class Test implements Messages {
@Override
public void display() {
System.out.println("Override");
}
public static void main(String[] args) {
Test test = new Test();
test.display();
}
}
Following is the error description produced by the above code fence.
Output:
Test.java:25: error: method does not override or implement a method from a supertype
@Override
^
1 error
Importance of Using static
Methods in an interface
We can get the following benefits from using static
methods in Java interfaces.
- A
static
method of aninterface
encapsulates the behavior we don’t want sub-classes or sub-interfaces to inherit or override. - These
static
methods of a Javainterface
are useful for developing reusable utilities that are not restricted to particular implementing types of classes.
Rules for static
Methods in an interface
The following section shows the rules for the above advantages in using static
methods.
The default methods of an interface hold the instruction for default behavior, and the implementing class can select to provide more concrete instructions via overriding it or inheriting it as is. Similar to default methods, the static
methods contain a body (set of instructions) for their behavior, but an implementing class is not allowed to inherit or override a static
method of its interface
as we have learned earlier.
One with a static
method in a Java interface
should remember the following rules.
- These methods must have a body.
- We can only execute these
static
methods using aninterface
name. - These methods must have a
static
modifier in the method’s declaration. If you don’t specify, it would bepublic
by default. Remember, we can also make themprivate
if we want. - They can not invoke other
abstract
or default methods. - Sub-classes or sub-interfaces are not allowed to override or inherit these
static
methods.
Now, at this point, you might have two questions in your mind.
- Why couldn’t we have
static
methods in the old days (before the release of Java 8)? - Why we can’t override
static
methods?
Let’s figure out the answers to both of these questions.
Reasons for Not Having static
Methods in Interfaces Before Java 8
There was no strong and primary technical cause why Java interfaces did not have static
methods before the release of Java 8. These static
methods were considered small language updates or changes, and then there was an official proposal to add it in Java 7, but later, it was dropped due to some complications.
Finally, in Java 8, we were introduced to static
methods in interfaces. As of Java 8, we also learned about override-able instance functions/methods with default implementations.
Remember, they still didn’t contain instance fields. These features are the part of lambda
expression support that you can read here (JSR 335 Part H).
Reasons for Not Being Able to Override a static
Method
The static
methods are resolved at compile time. The dynamic dispatches make sense about instance methods where a compiler cannot determine the object’s concrete type; thus, we cannot resolve a method to invoke.
But we need a class to execute a static
method; since that specific class is known statically
—at a compile time— a dynamic dispatch is unnecessary.
Suppose every class has a HashTable
which maps the signature of a method (name & parameter types) to the actual piece of code to implement the method. When the VM tries to execute a method on an instance, it queries an object for its class and looks for the requested signature in a table of classes.
It is invoked if the method body is found; otherwise, the class’ parent class is obtained where the lookup is repeated. This process continues until a method is found or no more parent classes are left (which produces NoSuchMethodError
).
If the respective tables have an entry for a subclass and a superclass for the same method signature, the subclass’s version would be encountered first, and the superclass’s version will never be used—this is an override
.
Assuming we skip an object instance and start with the subclass, the resolution could proceed as given above, providing you with a kind of overridable
static
method. Note that this resolution can only happen at compile time.
However, we know that a compiler starts from the known class instead of waiting until runtime to query an unspecified type’s object for its class. So, there would be no point in overriding a static
method.