How to Format Date in the SimpleDateFormat Class in Java
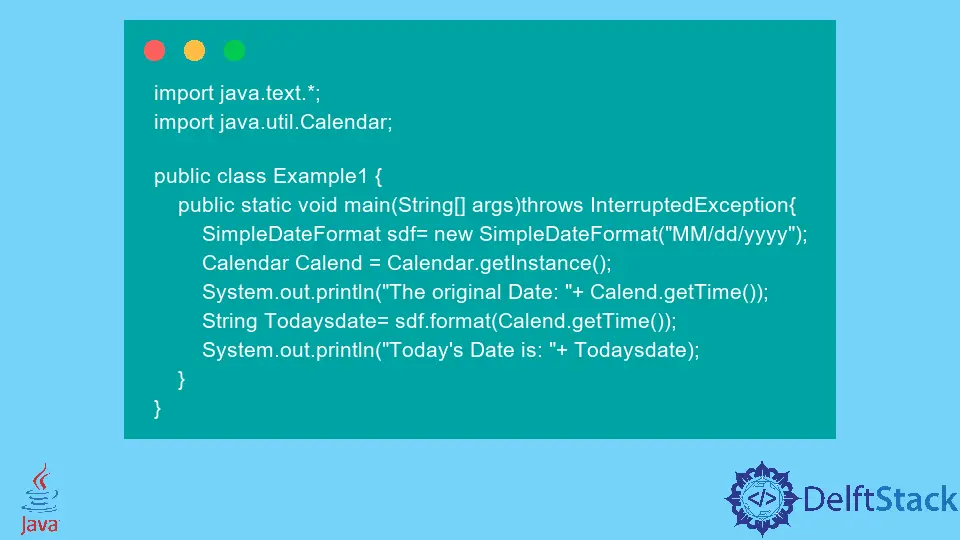
This article will show you what date formats are available in Java’s SimpleDateFormat class.
Date Format in the SimpleDateFormat Class in Java
The SimpleDateFormat class facilitates data formatting and processing. We can switch from one date format to another.
It allows the user to convert a date object from a string date format. To convert a given date into a DateTime string, utilize the format()
method of the SimpleDateFormat class.
This method converts the date and time into a particular format, such as MM/dd/yyyy. Click here to view more date and time patterns used in specifying date formats.
Syntax:
public final String format(Date date)
The method accepts one argument for the date of the Date
object type. It returns a date or time in the MM/dd/yyyy
format.
The following example will demonstrate how to implement the format()
method of SimpleDateFormat.
Example:
First, import the following libraries.
import java.text.*;
import java.util.Calendar;
Create a SimpleDateFormat type object named sdf
and pass the month, date, and year format as the argument in the main class.
SimpleDateFormat sdf = new SimpleDateFormat("MM/dd/yyyy");
Now, create a Calendar type object named Calend
.
Calendar Calend = Calendar.getInstance();
We can get the actual time by printing out the Calend
object using the .getTime()
method:
System.out.println("The original Date: " + Calend.getTime());
We can convert using the format()
method and then retrieve today’s date by printing the Todaysdate
object, which will output today’s current time in the MM/dd/yyyy format.
String Todaysdate = sdf.format(Calend.getTime());
System.out.println("Today's Date is: " + Todaysdate);
Example source code:
import java.text.*;
import java.util.Calendar;
public class Example1 {
public static void main(String[] args) throws InterruptedException {
SimpleDateFormat sdf = new SimpleDateFormat("MM/dd/yyyy");
Calendar Calend = Calendar.getInstance();
System.out.println("The original Date: " + Calend.getTime());
String Todaysdate = sdf.format(Calend.getTime());
System.out.println("Today's Date is: " + Todaysdate);
}
}
Output:
java -cp /tmp/gMkhJcqRJw Example1
The original Date: Fri Mar 25 03:55:00 GMT 2022
Today's Date is: 03/25/2022
I have been working as a Flutter app developer for a year now. Firebase and SQLite have been crucial in the development of my android apps. I have experience with C#, Windows Form Based C#, C, Java, PHP on WampServer, and HTML/CSS on MYSQL, and I have authored articles on their theory and issue solving. I'm a senior in an undergraduate program for a bachelor's degree in Information Technology.
LinkedIn