How to Create Array of Arrays in Java
- Create an Array of Arrays in Java by Direct Initialization
- Create an Array of Arrays in Java by Assigning a List of Arrays
-
Create an Array of Arrays in Java Using the
new
Keyword - Conclusion
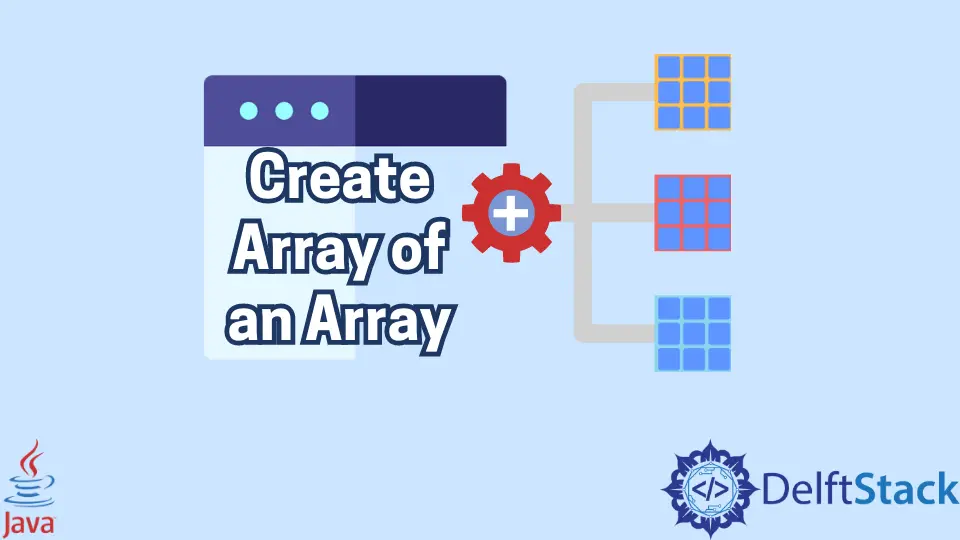
Arrays serve as fundamental data structures in Java, providing a means to organize and store elements of the same type efficiently. When it comes to multidimensional arrays, particularly arrays of arrays, Java offers several approaches to create these structures.
In this article, we will explore various methods to create a 2D array in Java, commonly known as an array of arrays. Understanding these methods not only facilitates the manipulation of data in a structured manner but also allows developers to choose the approach that best aligns with their specific requirements.
Whether it involves direct initialization, utilizing the new
keyword, or assigning a list of arrays, each method brings its advantages to the table.
Create an Array of Arrays in Java by Direct Initialization
Direct initialization during declaration is one of the simplest ways to create a 2D array in Java. This method allows developers to define the array and assign values to its elements in a concise manner.
To create a 2D array using direct initialization, we simply declare the array variable and immediately provide the values for its elements. The outer curly braces encapsulate the rows, and within each row, another set of curly braces contains the individual elements.
This approach is convenient for small-sized arrays where the elements are known at compile time.
Let’s explore a complete code example demonstrating direct initialization during declaration:
public class DirectInitializationExample {
public static void main(String[] args) {
// Creating a 2x4 2D array using direct initialization
int[][] array2D = {{1, 2, 3, 4}, {5, 6, 7, 8}};
// Displaying the 2D array
displayArray(array2D);
}
// Utility method to display the 2D array
private static void displayArray(int[][] array) {
for (int[] row : array) {
for (int value : row) {
System.out.print(value + " ");
}
System.out.println();
}
}
}
In this example, we declare and initialize a 2x4 2D array named array2D
directly during its declaration. The outer curly braces encapsulate the rows, and each row is represented by a set of curly braces containing the individual elements.
We then call the displayArray
utility method to print the contents of the 2D array to the console. This method uses nested enhanced for
loops to iterate through each row and column, printing each element followed by a space and adding a new line character after printing the elements of a row.
Code Output:
This output confirms the successful creation and display of a 2D array using direct initialization during declaration. The concise syntax makes it easy to visualize and input the array elements directly within the code.
Create an Array of Arrays in Java by Assigning a List of Arrays
One approach to creating an array of arrays, also known as a 2D array, involves assigning a list of arrays to create a flexible and dynamic structure.
The process involves using a List
to hold arrays, each representing a row in the 2D array. The List
is then converted to a 2D array using the toArray()
method.
This method provides a straightforward and concise way to initialize a 2D array with a dynamic number of rows and columns.
Let’s take a look at the complete code example:
import java.util.ArrayList;
import java.util.List;
public class ArrayOfArraysExample {
public static void main(String[] args) {
// Creating a List of Arrays
List<int[]> listOfArrays = new ArrayList<>();
// Adding arrays to the list
listOfArrays.add(new int[] {1, 2, 3});
listOfArrays.add(new int[] {4, 5, 6});
listOfArrays.add(new int[] {7, 8, 9});
// Converting the List to a 2D array
int[][] array2D = listOfArrays.toArray(new int[0][]);
// Displaying the 2D array
displayArray(array2D);
}
// Utility method to display the 2D array
private static void displayArray(int[][] array) {
for (int[] row : array) {
for (int value : row) {
System.out.print(value + " ");
}
System.out.println();
}
}
}
In this Java code example, firstly, a List
of integer arrays, named listOfArrays
, is created using the ArrayList
class. This list will be used to store individual arrays, each representing a row in the 2D array.
Three arrays are then added to the list using the add
method. These arrays, created using the new int[]{...}
syntax, contain integer values to populate the rows of the 2D array.
In this case, we have three rows, each with three elements.
Next, the toArray
method is used to convert the List
of arrays (listOfArrays
) into a 2D array (array2D
). The method takes an array of the desired type as an argument (in this case, new int[0][]
), and it dynamically determines the size of the resulting array based on the size of the list.
Finally, a utility method named displayArray
is called to print the contents of the 2D array to the console. This method uses nested enhanced for
loops to iterate through each row and column of the array, printing each element followed by a space.
After printing the elements of a row, a newline character is added to move to the next row.
Code Output:
This output demonstrates the successful creation and display of a 2D array initialized by assigning a list of arrays. The rows and columns are populated as specified in the arrays added to the list.
Create an Array of Arrays in Java Using the new
Keyword
Another method to create a 2D array in Java involves using the new
keyword. This approach provides explicit control over the array size and allows for a manual population of the array elements.
To create a 2D array using the new
keyword, we declare a variable for the array, specifying the number of rows and columns within square brackets. We then use nested loops to iterate over each row and column, assigning values to individual elements.
This method offers flexibility in determining the size of the array and provides fine-grained control over element initialization.
Here’s the basic syntax of the new
keyword:
dataType[][] arrayName = new dataType[rows][columns];
Let’s take a look at a complete code example:
public class NewKeywordArrayExample {
public static void main(String[] args) {
// Creating a 3x4 2D array using the new keyword
int[][] array2D = new int[3][4];
// Populating the array with values
for (int i = 0; i < array2D.length; i++) {
for (int j = 0; j < array2D[i].length; j++) {
array2D[i][j] = i * array2D[i].length + j + 1;
}
}
// Displaying the 2D array
displayArray(array2D);
}
// Utility method to display the 2D array
private static void displayArray(int[][] array) {
for (int[] row : array) {
for (int value : row) {
System.out.print(value + " ");
}
System.out.println();
}
}
}
This Java program begins by declaring a 3x4 2D array named array2D
using the new
keyword: int[][] array2D = new int[3][4];
. This statement allocates memory for a 2D array with three rows and four columns, initializing each element to its default value (zero for integers).
Next, a nested loop structure is employed to iterate over each row and column of the array. The outer loop (for (int i = 0; i < array2D.length; i++)
) iterates over the rows, and the inner loop (for (int j = 0; j < array2D[i].length; j++)
) iterates over the columns.
Within the inner loop, the elements of the array are populated using the expression i * array2D[i].length + j + 1
, ensuring each element receives a unique sequential value starting from 1.
After populating the array, a utility method named displayArray
is called to print the contents of the 2D array to the console. This method uses nested enhanced for
loops to iterate through each row and column, printing each element followed by a space.
A newline character is added after printing the elements of a row to format the output in a matrix-like structure.
Code Output:
This output showcases the successful creation of a 2D array using the new
keyword, where the array is explicitly sized and populated with sequential values. The nested loops provide an organized way to iterate through each row and column of the array, facilitating precise element initialization.
Conclusion
Creating an array of arrays in Java provides developers with diverse methods catering to different scenarios and preferences. Whether through direct initialization during declaration, the use of the new
keyword, or assigning a list of arrays, Java offers the flexibility needed for efficient handling of multidimensional arrays.
Each method has its strengths, and the choice depends on factors such as array size, element values, and the level of control required during array creation. Understanding these approaches empowers developers to select the most suitable method for their specific use cases, enhancing the versatility of Java in array manipulation.
Ultimately, Java’s array capabilities allow for the construction of complex data structures, facilitating the development of robust and scalable applications.