How to Create and Run a Java JAR File
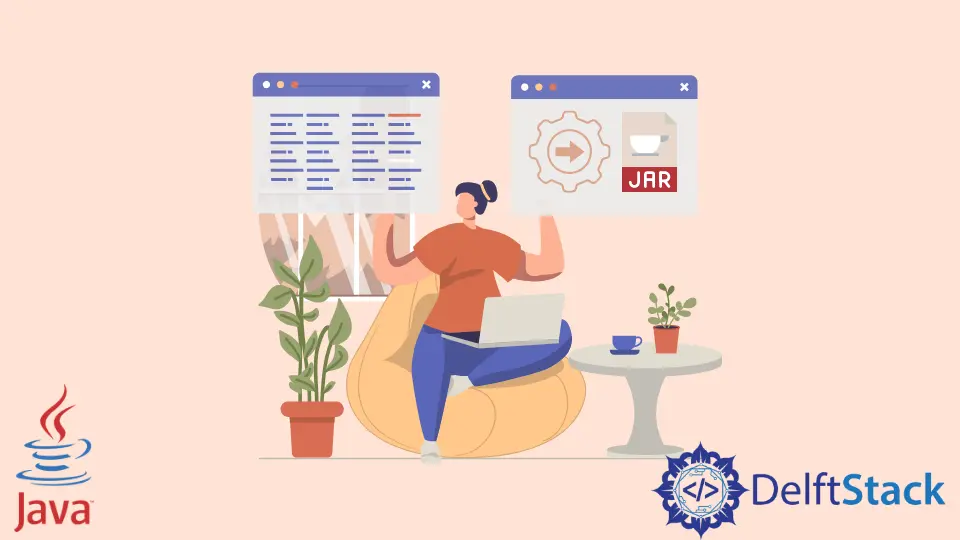
The JAR file is a bundle of different Java class files and the metadata you need to execute a program.
This article discusses the method of running a JAR file in Java.
Create and Run a Java JAR File
Although the JAR file primarily contains the Java class files and metadata, you can not simply bundle them together in a file and execute it.
Instead, you need a manifest file that mentions the information required to execute the program. The most important information is the main class.
Therefore, you need to mention the main class in the manifest file and create a JAR file, including the manifest file in the bundle.
When you execute the JAR file, the class mentioned in the manifest file is the beginning point of the program execution.
Let’s create a JAR file with the following Java class.
public class MyClass {
public static void main(String[] args) {
System.out.println("Hi! We are Delftstack!");
}
}
The manifest file (manifest.mf
) is given below.
Manifest-version: 1.0
Main-Class: MyClass
You must put a newline at the end of the manifest file. Otherwise, the file will not be executed.
You can create a JAR file by executing the following commands on the terminal.
javac MyClass.java
jar cfm myjar.jar manifest.mf MyClass.class
You can execute the following command to run the JAR file thus created.
java -jar myjar.jar
Create a Java JAR File Without Manifest
If you do not want to include the manifest file to create a JAR file, you can use the alternative method that specifies the main class name with the command.
java -classpath myjar.jar MyClass
Yet another method to create a JAR file is to specify the classpath
and the package qualified class name. You can use the command provided below to create a JAR file.
jar cvfe myjar.jar MyClass *.class
Conclusion
Apart from running the JAR file from the bash terminal, you can also double-click the JAR file to execute it. This is all about running a JAR file.