Covariant Return Type in Java
- What is Covariant Return Type?
- Benefits of Using Covariant Return Types
- Practical Applications of Covariant Return Types
- Conclusion
- FAQ
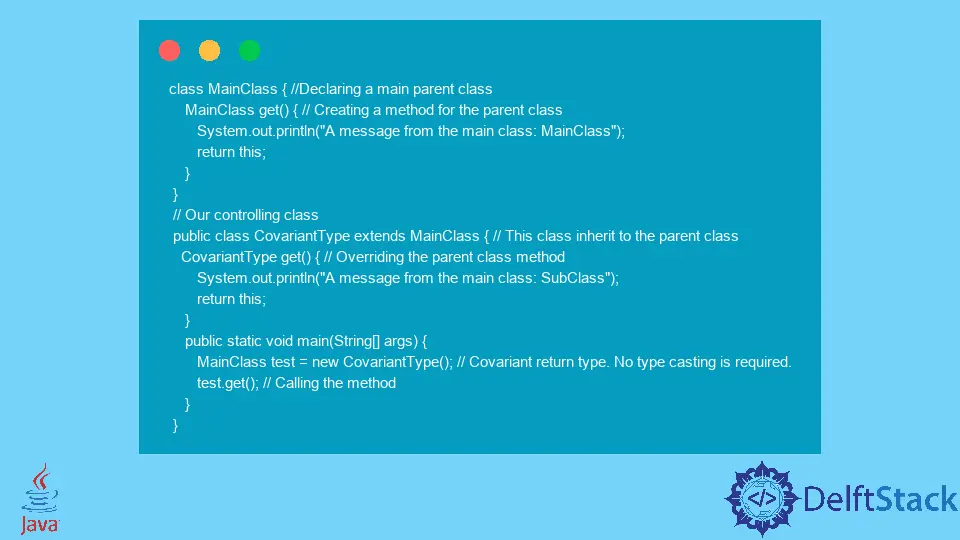
In the world of Java programming, understanding the nuances of return types can significantly enhance your coding skills. One such concept that often intrigues developers is the covariant return type. This feature allows a method in a subclass to override a method in its superclass while changing the return type to a subtype of the original return type.
This tutorial dives deep into the covariant return type in Java, exploring its utility, applications, and how it can improve code readability and maintainability. Whether you are a seasoned developer or a newcomer to Java, this guide will provide you with valuable insights and practical examples to help you grasp this essential concept.
What is Covariant Return Type?
Covariant return types were introduced in Java 5, allowing a method to return a more specific type than its superclass method. This means that if a superclass method returns an object of type A, a subclass can override that method to return an object of type B, where B is a subclass of A. This feature is particularly useful in object-oriented programming, as it enhances polymorphism and allows for more flexible and expressive code designs.
To illustrate this, consider the following example:
class Animal {
Animal getAnimal() {
return new Animal();
}
}
class Dog extends Animal {
Dog getAnimal() {
return new Dog();
}
}
In this example, the Dog
class overrides the getAnimal
method from the Animal
class. While the superclass method returns an Animal
type, the subclass method returns a Dog
type, demonstrating the covariant return type.
The ability to use covariant return types not only simplifies code but also enhances type safety. By allowing subclasses to specify more precise return types, developers can create more intuitive APIs and ensure that the returned objects are of the expected type.
Benefits of Using Covariant Return Types
Using covariant return types in Java comes with several benefits. First and foremost, it promotes code reusability. By allowing subclasses to return more specific types, developers can create a hierarchy of classes that share common behavior while also providing specialized functionality. This is particularly beneficial in large codebases where maintaining consistency and reducing redundancy is crucial.
Another advantage is improved readability. When methods return more specific types, it becomes easier for other developers (or even your future self) to understand what type of object is expected from a method call. This clarity can lead to fewer bugs and a smoother development process.
Additionally, covariant return types enhance the flexibility of your code. You can create a robust class hierarchy without being constrained by the return types of superclass methods. This flexibility can be particularly useful in frameworks and libraries where extensibility is key.
Practical Applications of Covariant Return Types
Covariant return types can be applied in various scenarios. One common use case is in the implementation of design patterns. For example, in the Factory Method pattern, you may have a base class that defines a method for creating objects. Subclasses can then override this method to return a more specific type, allowing for a cleaner and more maintainable code structure.
Consider the following example that demonstrates the Factory Method pattern using covariant return types:
abstract class Shape {
abstract Shape create();
}
class Circle extends Shape {
Circle create() {
return new Circle();
}
}
class Square extends Shape {
Square create() {
return new Square();
}
}
In this example, the Shape
class defines an abstract method create
that returns a Shape
. Each subclass implements this method, returning its specific type, either Circle
or Square
. This design allows for easy expansion of shape types without modifying existing code.
The use of covariant return types in this context not only adheres to the Open/Closed Principle of software design but also provides a clear and intuitive API for users of the Shape
class. This approach can significantly reduce the likelihood of errors and improve the overall structure of your code.
Conclusion
In conclusion, covariant return types in Java offer a powerful way to enhance your object-oriented programming skills. By allowing subclasses to return more specific types, you can create more flexible, maintainable, and readable code. This feature not only promotes code reuse but also improves clarity and reduces the risk of errors. As you continue your Java journey, consider leveraging covariant return types to take your coding practices to the next level. Embrace this powerful feature and watch your Java applications become more elegant and robust.
FAQ
- What is a covariant return type in Java?
A covariant return type allows a subclass method to override a superclass method and change the return type to a subtype of the original return type.
-
Why use covariant return types?
They promote code reusability, improve readability, and enhance flexibility by allowing subclasses to return more specific types. -
When were covariant return types introduced in Java?
Covariant return types were introduced in Java 5. -
Can you provide an example of covariant return types?
Yes, a method in a subclass can return a specific type while overriding a method in its superclass that returns a more general type. -
How do covariant return types improve polymorphism?
They allow for more specific return types in subclasses, enhancing the polymorphic behavior of objects in Java.
Aminul Is an Expert Technical Writer and Full-Stack Developer. He has hands-on working experience on numerous Developer Platforms and SAAS startups. He is highly skilled in numerous Programming languages and Frameworks. He can write professional technical articles like Reviews, Programming, Documentation, SOP, User manual, Whitepaper, etc.
LinkedIn