console.log in Java
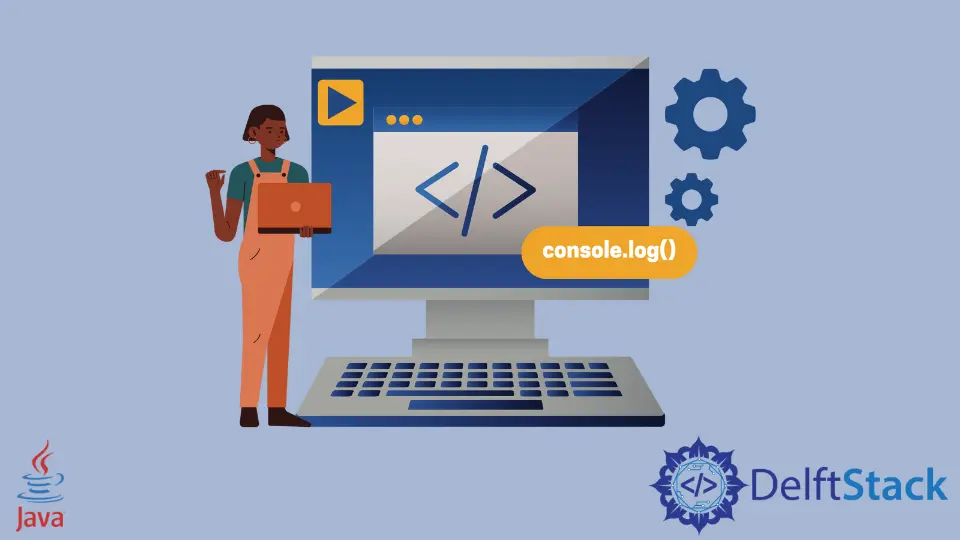
This tutorial introduces the console.log()
function in Java and how to display log to console in Java.
The console.log()
is a function of JavaScript that is used to display log messages to the browser console. There is no such message in Java, but System.out.println()
does a similar task.
So remember, if somebody asks you about this method and claim to belong to Java, there is no such thing because Java does not have any method with this name.
We can use the System.out.println()
method to display messages to the console as JavaScript in Java. Let’s understand with examples.
console.log
in Java
In Java, we can use the println()
method as console.log()
to display log messages to the console. See the example below.
public class SimpleTesting {
public static void main(String[] args) {
System.out.println("Hello DelftStack");
}
}
Output:
Hello DelftStack
Custom console.log()
Method in Java
If you want to use the console.log()
method in Java, you can create a Console
class and add a static log()
method. This method has a single print statement to print the message to the console.
After creating this class, you can call the log()
method by class name. See the example below.
public class SimpleTesting {
public static void main(String[] args) {
Console.log("Hello DelftStack");
}
}
class Console {
public static void log(Object obj) {
System.out.println(obj);
}
}
Output:
Hello DelftStack