The *= Operator in Java
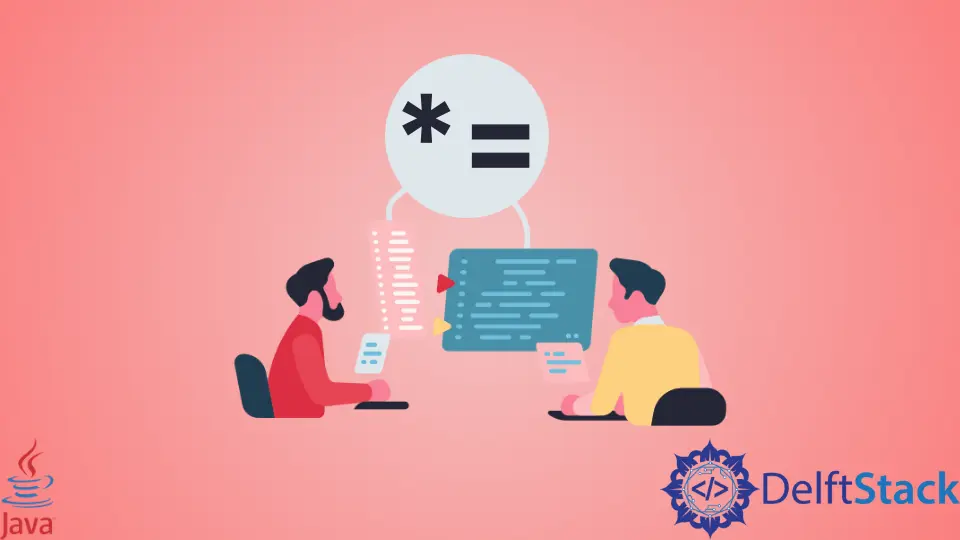
This tutorial introduces the *=
operator and how to use it in Java.
The *=
operator is a combined operator that consists of the *
(multiply) and =
(assignment) operators. This first multiplies and then assigns the result to the left operand.
This operator is also known as shorthand operator and makes code more concise. In this article, we will learn to use this operator with examples.
The Multiplication Operator in Java
In this example, we used the multiply operator to get the product of a value and then assign it to use the assignment operator. This is a simple way to multiply in Java.
public class SimpleTesting {
public static void main(String[] args) {
int val = 125;
int result = val * 10;
System.out.println("Multiplication of " + val + "*10 = " + result);
}
}
Output:
Multiplication of 125*10 = 1250
Shorthand Multiply Operator in Java
Now, let’s use the shorthand operator to get the remainder. See, the code has concise and produces the same result as the above code did.
public class SimpleTesting {
public static void main(String[] args) {
int val = 125;
int temp = val;
val *= 10; // shorthand operator
System.out.println("Multiplication of " + temp + "*10 = " + val);
}
}
Output:
Multiplication of 125*10 = 1250
Shorthand Operators in Java
Java supports several other compound assignment operators such as +=
, -=
, *=
, etc. In this example, we used other shorthand operators so that you can understand the use of these operators well.
See the example below.
public class SimpleTesting {
public static void main(String[] args) {
int val = 125;
System.out.println("val = " + val);
val += 10; // addition
System.out.println("val = " + val);
val -= 10; // subtraction
System.out.println("val = " + val);
val *= 10; // multiplication
System.out.println("val = " + val);
val /= 10; // division
System.out.println("val = " + val);
val %= 10; // compound operator
System.out.println("val = " + val);
}
}
Output:
val = 125
val = 135
val = 125
val = 1250
val = 125
val = 5