How to Compare Doubles in Java
-
Use
Compare(Double a, Double b)
to Compare Doubles in Java -
Use
d2.CompareTo(d1)
to Compare Doubles in Java
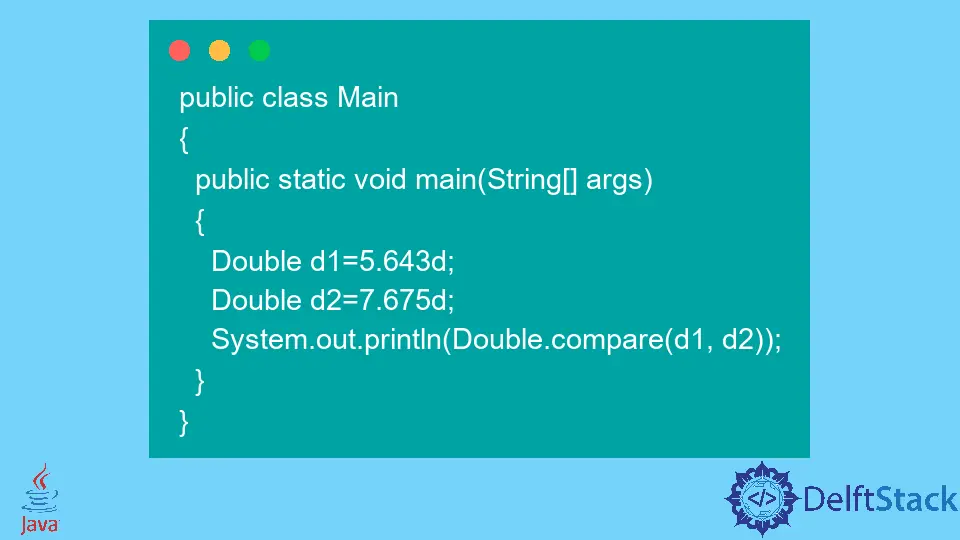
This guide will tell you how to compare doubles in the Java.
There are some built-in methods to compare doubles. The methods are explained in this guide.
Let’s dive in.
The built-in methods for comparing doubles in Java are called compare(d1, d2)
and d2.compareTo(d1)
.
The d1
and d2
are just variable names, not part of the syntax. Let’s understand these methods one by one.
Use Compare(Double a, Double b)
to Compare Doubles in Java
You can compare the two doubles using this method by passing them as arguments.
The two double values will be compared. If both the doubles are numerically equal, the function will give 0
as output.
If the first parameter, in this case, a
, is numerically less than the second parameter b
, the function will give a value less than 0
. It will give a value greater than 0
if a
is greater than b
.
Take a look at the following code.
public class Main {
public static void main(String[] args) {
Double d1 = 5.643d;
Double d2 = 7.675d;
System.out.println(Double.compare(d1, d2));
}
}
Output:
-1
Use d2.CompareTo(d1)
to Compare Doubles in Java
In this method, you compare d2
with d1
. The value will be 0
if both have the same value.
The value will be less than 0
if d2
is numerically less than d1
. The value will be greater than 0
if d2
is numerically greater than d1
.
public class Main {
public static void main(String[] args) {
Double d1 = 5.643d;
Double d2 = 7.675d;
System.out.println(d1.compareTo(d2));
}
}
Output:
-1
Haider specializes in technical writing. He has a solid background in computer science that allows him to create engaging, original, and compelling technical tutorials. In his free time, he enjoys adding new skills to his repertoire and watching Netflix.
LinkedIn