Collision in Hashmap in Java
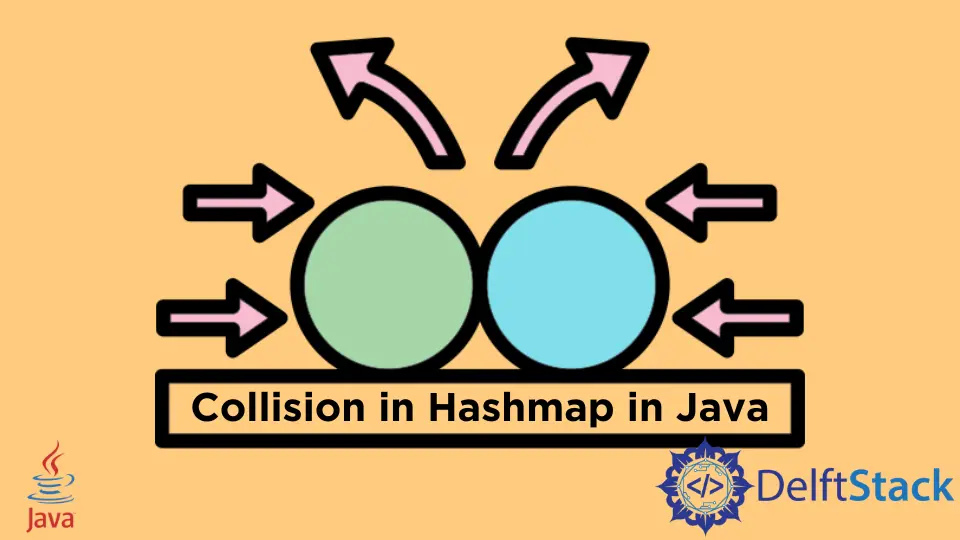
Java collections interface provides the functionality of the hash table data structure using its HashMap
class. This class stores the elements in a key-value pair where keys act as identifiers and are unique associated with a value in the map.
In this tutorial, we will discuss collision in Java.
The HashMap
key contains a hashcode, and a equals()
method. Whenever we insert a new entry to the Map, it checks for the hashcode. It parses through the entire pool of objects, searching for similarity of the hashcode using the equals()
method.
If any entry is existent, the new value will then replace the primarily existing value. Otherwise, it will simply create a whole new key-value pair.
Collision happens when multiple keys hash to the same bucket or, say when two or more objects have the same hashcode but are different. When two keys get hashed to the same value, a linked list is formed at the bucket location, where all the information is stored as an entry of the map, which contains the key-value pair.
Accessing any object could turn out to be cumbersome if the entries are present inside the lists. These linked lists were converted to binary trees from Java 8 version.
HashMap
handles collision cases very efficiently using a concept known as chaining, which suggests storing the values in a linked list or a binary tree as indicated by the conversion of methodology from Java 8.