How to Fix Class Names Are Only Accepted if Annotation Processing Is Explicitly Requested in Java
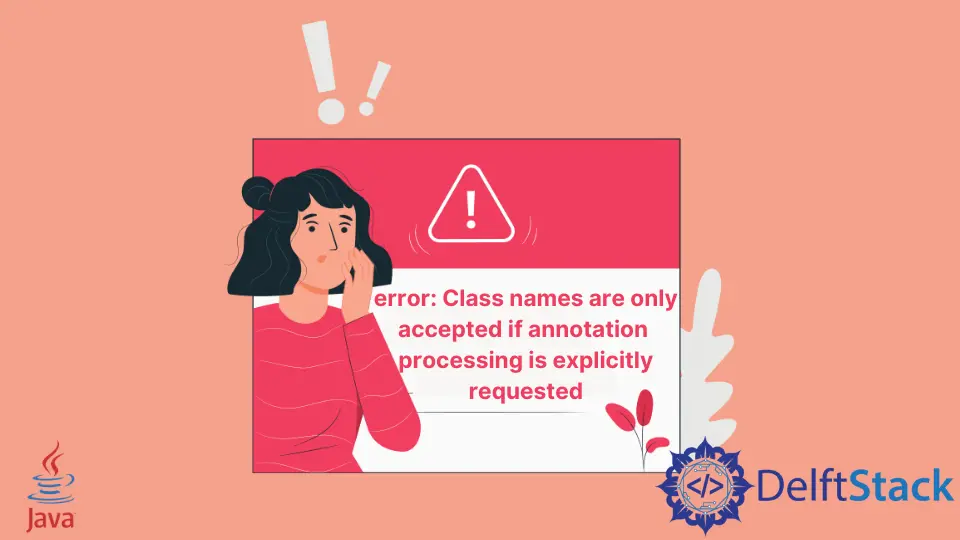
This tutorial highlights the reasons and guides how to fix this error using a sample Java program.
Fix Class Names, 'test.java', Are Only Accepted If Annotation Processing Is Explicitly Requested
in Java
Before moving towards the solution, let’s write the following Java program to understand the possible reasons for having this error.
Example Code:
// test class
public class test {
// main()
public static void main(String[] args) {
// print message
System.out.println("Hello, this is just a test program.");
} // end main()
} // end test class
Now, use the command given below to compile the Java code.
javac writeYourFileNameHere
Here, we will get the error saying class names, 'test.java', are only accepted if annotation processing is explicitly requested
(test.java
is our file name, you will see your file name there).
Why is it so? As per Java documentation, there are two possible reasons for having this error.
- We forget to add the
.java
suffix at the end of the file name. - We use improper capitalization of the
.java
suffix. For instance, we compile asjavac test.Java
You can find both commands in the following screenshot.
How to resolve this error?
The solution for this compile time error is very simple. We only need to add the .java
suffix (all in small letters).
Let’s do it by using the sample code below.
Example Code:
// test class
public class test {
// main()
public static void main(String[] args) {
// print message
System.out.println("Hello, this is just a test program.");
} // end main()
} // end test class
This time, we compiled the code using javac test.java
. If it compiles successfully, we use the java test
command to run the program (don’t forget to write your own file’s name).
Both of these commands are demonstrated in the following screenshot.
OUTPUT:
Related Article - Java Error
- How to Fix the Error: Failed to Create the Java Virtual Machine
- How to Fix the Missing Server JVM Error in Java
- How to Fix the 'No Java Virtual Machine Was Found' Error in Eclipse
- How to Fix Javax.Net.SSL.SSLHandShakeException: Remote Host Closed Connection During Handshake
- How to Fix the Error: Failed to Create the Java Virtual Machine
- How to Fix Java.Lang.VerifyError: Bad Type on Operand Stack