How to Check if a Character Is a Number in Java
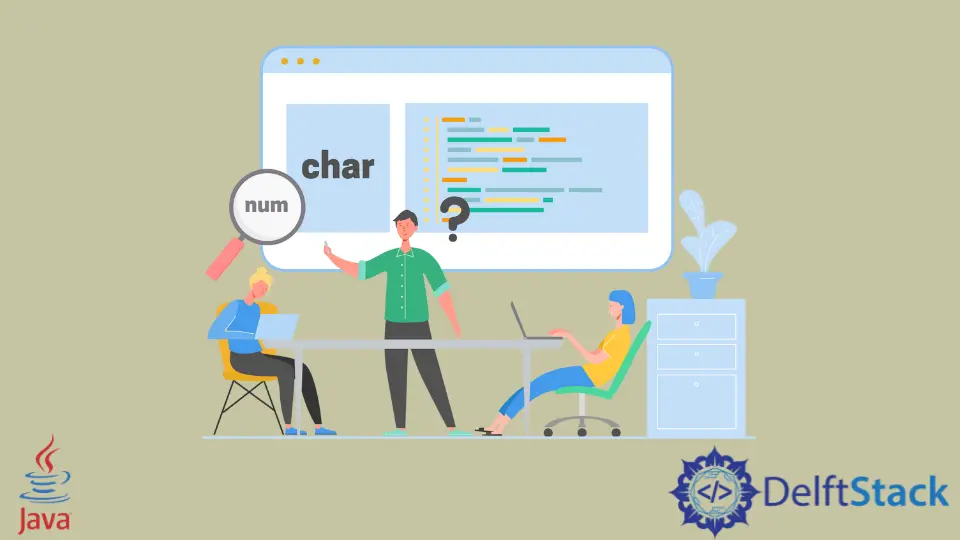
When working with Java, you may often find yourself needing to determine if a character is a numeric digit. This task can arise in various scenarios, such as input validation, parsing data, or processing user inputs. Thankfully, Java provides several straightforward methods to accomplish this.
In this article, we will explore different approaches to check if a character is a number in Java, complete with code examples and detailed explanations. Whether you’re a beginner or an experienced developer, you’ll find these methods easy to implement and understand. Let’s dive in!
Using Character.isDigit Method
One of the simplest and most efficient ways to check if a character is a number in Java is by using the Character.isDigit()
method. This method is part of the Character class and checks if the specified character is a digit (0-9).
Here’s how you can use it:
public class CheckIfDigit {
public static void main(String[] args) {
char ch = '5';
if (Character.isDigit(ch)) {
System.out.println(ch + " is a digit.");
} else {
System.out.println(ch + " is not a digit.");
}
}
}
Output:
5 is a digit.
In this example, we declare a character variable ch
and assign it the value ‘5’. By calling Character.isDigit(ch)
, we check if ch
is a digit. If it is, we print a message confirming that it’s a digit; otherwise, we indicate that it is not. This method is efficient and easy to understand, making it a popular choice among Java developers.
Using Regular Expressions
Another powerful way to check if a character is a number is by using regular expressions. This method allows for more complex patterns and can be very useful if you need to validate strings or characters against specific criteria.
Here’s an example of using regular expressions to check if a character is a digit:
import java.util.regex.Pattern;
public class CheckIfDigitRegex {
public static void main(String[] args) {
char ch = '8';
String str = String.valueOf(ch);
if (Pattern.matches("[0-9]", str)) {
System.out.println(ch + " is a digit.");
} else {
System.out.println(ch + " is not a digit.");
}
}
}
Output:
8 is a digit.
In this code, we first convert the character ch
into a string. We then use Pattern.matches()
with the regex pattern [0-9]
, which matches any digit from 0 to 9. If the character matches this pattern, we print that it is a digit; otherwise, we indicate it is not. Regular expressions provide a flexible way to perform validations, but they can be slightly more complex than using the Character.isDigit()
method.
Using ASCII Values
A more manual approach to check if a character is a number involves examining its ASCII value. Each character has a corresponding ASCII value, and for digits, these values range from 48 (‘0’) to 57 (‘9’). This method is less common but can be useful for those interested in low-level character manipulation.
Here’s how you can implement it:
public class CheckIfDigitASCII {
public static void main(String[] args) {
char ch = '3';
if (ch >= '0' && ch <= '9') {
System.out.println(ch + " is a digit.");
} else {
System.out.println(ch + " is not a digit.");
}
}
}
Output:
3 is a digit.
In this example, we check if the character ch
falls within the range of ‘0’ to ‘9’. If it does, we confirm that it is a digit; otherwise, we state that it is not. This method is straightforward and effective for simple digit checks, but it may not be as readable as the other methods.
Conclusion
In conclusion, checking if a character is a number in Java can be accomplished through various methods, including using Character.isDigit()
, regular expressions, and ASCII value comparisons. Each method has its advantages and can be chosen based on your specific needs or preferences. Whether you’re validating user input or processing data, these techniques will help you ensure that your Java applications handle numeric characters correctly. By mastering these methods, you can enhance your programming skills and improve the robustness of your applications.
FAQ
-
How does the Character.isDigit method work?
The Character.isDigit method checks if the specified character is a digit (0-9) and returns true or false. -
What are regular expressions in Java?
Regular expressions are a sequence of characters that define a search pattern, often used for string matching and validation. -
Why would I use ASCII values to check digits?
Using ASCII values allows for a low-level approach to character manipulation, which can be helpful in certain programming scenarios. -
Are there performance differences between these methods?
Generally, Character.isDigit is the most efficient, while regular expressions may have overhead due to their complexity. -
Can I check for other numeric types, like decimals or negative numbers?
Yes, you can modify the methods to check for decimal points or negative signs by adjusting the conditions or regex patterns accordingly.
Haider specializes in technical writing. He has a solid background in computer science that allows him to create engaging, original, and compelling technical tutorials. In his free time, he enjoys adding new skills to his repertoire and watching Netflix.
LinkedIn