CET Time in Java
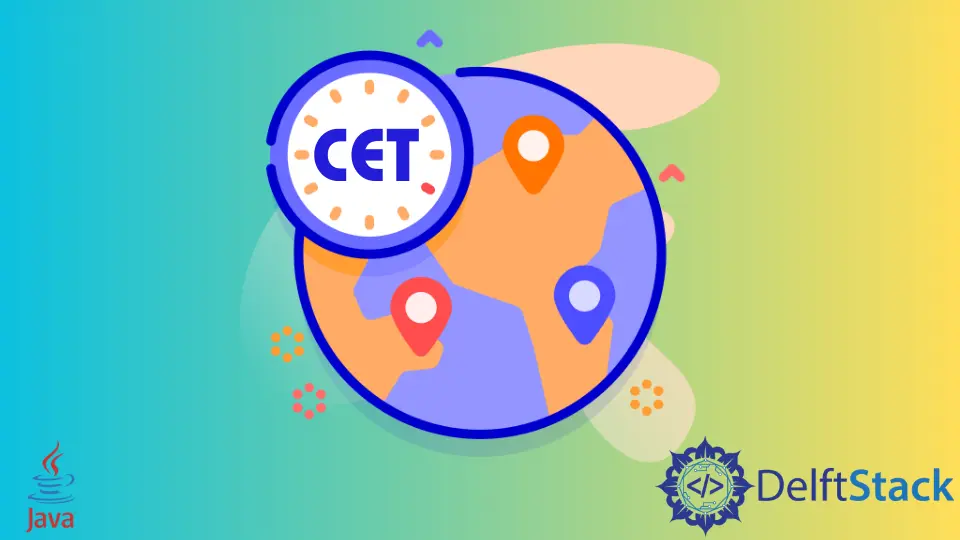
In the whole wide world, there are 190+ countries, where each country follows a certain time-zone. These time zones act as rules which are crucial for expressing time much conveniently as well as effectively. However, these time zones can turn out to be inexplicit due to certain variables such as daylight saving time.
Java has provided us with multiple classes that handle time zones. With new versions of Java coming up, it came up with much more extensive and useful classes such as ZoneId
and ZoneOffset
.
With the emergence of JSR-310, some new and much more productive APIs were added for managing time and time zones. ZoneId
was one such class added. It is a representation of a time zone such as Europe/Berlin
.
Most fixed offsets are represented by ZoneOffset
. We can ensure by calling normalized()
on any ZoneId
that a fixed offset ID will be represented as ZoneOffset
.
The IANA definition of CET says that it follows the time-zone rules of Central Europe, which does include both summers as well as winters time.
One should understand that the time-zone identifier and the short name of that identifier are two different elements. The name may change between CET
and CEST
, but the identifier is always fixed as CET
.
We can get the functionality related to the CET
time-zone using the getRules()
function. It can convey the offset at the given time.
See the following code.
import java.time.LocalDateTime;
import java.time.ZoneId;
import java.time.ZonedDateTime;
import java.time.format.DateTimeFormatter;
public class Main {
public static void main(String[] args) {
ZoneId zone = ZoneId.of("CET");
System.out.println("Zone Name: " + zone);
System.out.println("Offset " + zone.getRules());
}
}
Output:
Zone Name: CET
Offset ZoneRules[currentStandardOffset=+01:00]
Other way might be to use ZoneOffset.ofHours(1)
rather than using ZoneId.of("CET")
. We can get the total transitions and rules for the transition using the getTransitions()
and getTransitionRules()
.