How to Fix Java Cannot Be Resolved to a Variable Error
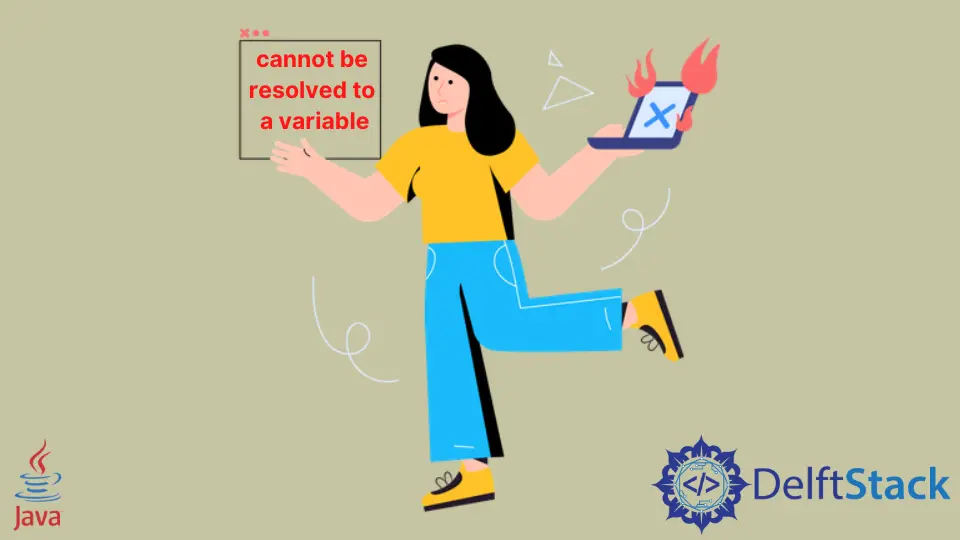
This guide will teach you how to fix the cannot be resolved to a variable
error in Java.
For this, you need to understand the scope of a programming language. Keep reading this compact guide to learn more and get your fix to this error.
Fix the cannot be resolved to a variable
Error in Java
In Java programming language, we use curly brackets {}
to identify the scope of a class, functions, and different methods.
For instance, take a look at the following code:
public static void calculateSquareArea(int x) {
System.out.println(x * x);
}
In the above code example, the scope of the variable x
is limited within the curly brackets {}
. You cannot call or use it outside this scope. If you try, the cannot be resolved to a variable
error will come out.
It means it cannot detect the initialization of variables within its scope. Similarly, if you make a private
variable, you cannot call it inside a constructor.
Its scope is out of bounds. Here’s the self-explanatory code.
public class Main {
public static void main(String args[]) {
int var = 3;
// scope is limited within main Block;
// The Scope of var Amount Is Limited..........
// Accessible only Within this block............
}
public static void Calculate(int amount) {
// The Scope of Variable Amount Is Limited..........
// Accessible only Within this block............
}
}
Haider specializes in technical writing. He has a solid background in computer science that allows him to create engaging, original, and compelling technical tutorials. In his free time, he enjoys adding new skills to his repertoire and watching Netflix.
LinkedInRelated Article - Java Scope
Related Article - Java Error
- How to Fix the Error: Failed to Create the Java Virtual Machine
- How to Fix the Missing Server JVM Error in Java
- How to Fix the 'No Java Virtual Machine Was Found' Error in Eclipse
- How to Fix Javax.Net.SSL.SSLHandShakeException: Remote Host Closed Connection During Handshake
- How to Fix the Error: Failed to Create the Java Virtual Machine
- How to Fix Java.Lang.VerifyError: Bad Type on Operand Stack