How to Calculate Leap Year in Java
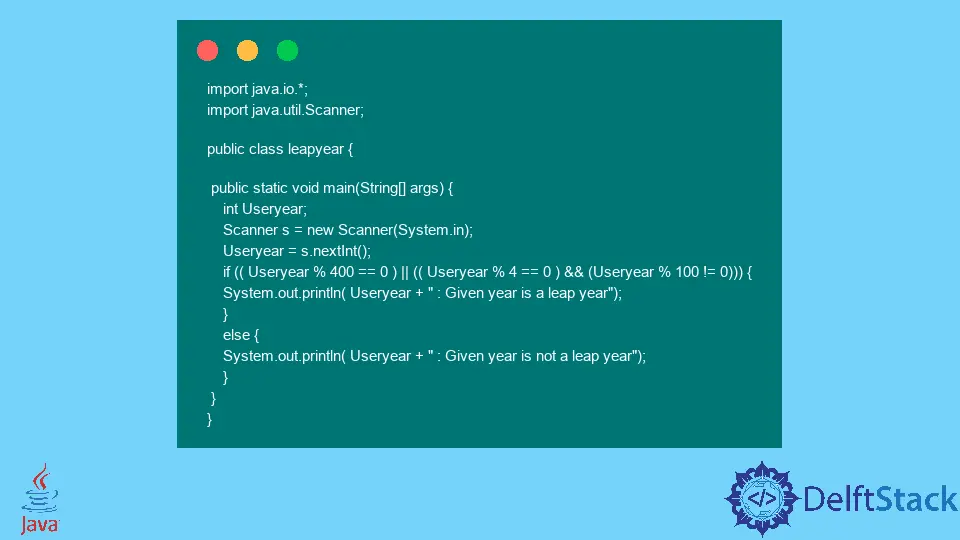
Every four(4) years, a Leap Year is added to the calendar by adding one additional day, February 29, to the calendar. In today’s tutorial, we’ll learn how to compute Leap Year using Java
.
Calculating Leap Year Using Java
Generally, a Leap Year occurs every four years, although regulations for leap years are stated below.
- If a year is equally divisible by four, it is a leap year.
- The years divisible by
100
, such as1900
or2000
, cannot be leap years unless they are also divisible by400
. As result, while the years1600
and2000
had leap years, the years1700
,1800
, and1900
did not. - It is a leap year if a year meets both preceding criteria.
Let’s have an example. First, the Scanner
class is imported here for the user can enter whatever year they like.
The remainder of the if-else
blocks is consolidated into a single line to verify if the input year is a leap year. The code example below shows how to check if a year is a leap year or not.
To begin, import the following libraries.
import java.io.*;
import java.util.Scanner;
To keep the year specified by the user, create an int
type variable named Useryear
in the main
class.
int Useryear;
Initialize a Scanner
class object. Then, using an object of the Scanner
class called s
, we take input from the user.
Scanner s = new Scanner(System.in);
Useryear = s.nextInt();
In the first condition, we check if it is a century leap year, and in the 2nd condition, we check if it is a leap year and not a century year.
if ((Useryear % 400 == 0) || ((Useryear % 4 == 0) && (Useryear % 100 != 0)))
If both conditions are true, it will print "Given year is a leap year"
. Otherwise, a "Given year is not a leap year"
.
Full Source Code:
import java.io.*;
import java.util.Scanner;
public class leapyear {
public static void main(String[] args) {
int Useryear;
Scanner s = new Scanner(System.in);
Useryear = s.nextInt();
if ((Useryear % 400 == 0) || ((Useryear % 4 == 0) && (Useryear % 100 != 0))) {
System.out.println(Useryear + " : Given year is a leap year");
} else {
System.out.println(Useryear + " : Given year is not a leap year");
}
}
}
Output:
// Input Year
2000
2000 : Given year is a leap year
// Input Year
2022
2022 : Given year is not a leap year
I have been working as a Flutter app developer for a year now. Firebase and SQLite have been crucial in the development of my android apps. I have experience with C#, Windows Form Based C#, C, Java, PHP on WampServer, and HTML/CSS on MYSQL, and I have authored articles on their theory and issue solving. I'm a senior in an undergraduate program for a bachelor's degree in Information Technology.
LinkedIn