How to Calculate Average in Java
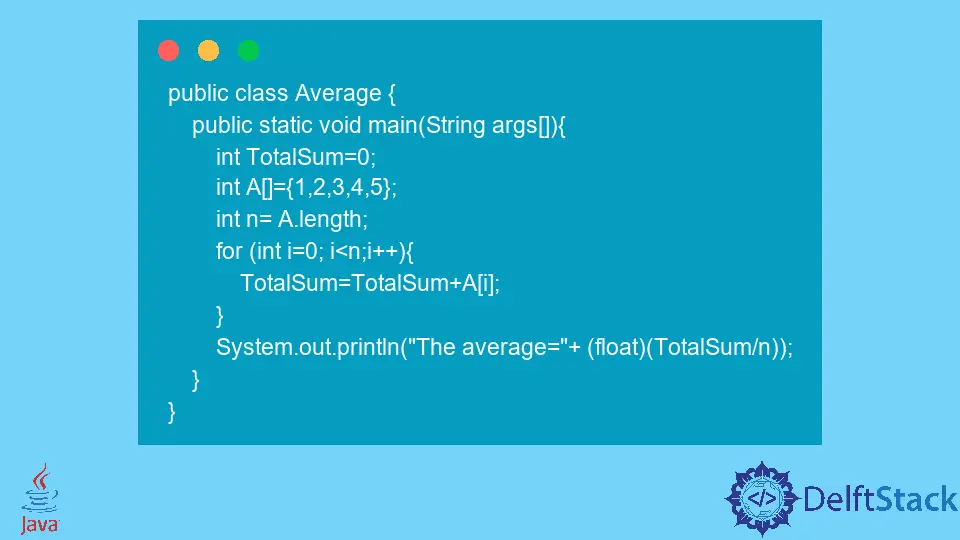
In this tutorial, we will calculate the average of an array of numbers in Java.
As we know, the average is the sum of all values divided by the total number of values. We will be applying this formula in our code.
First, we will collect some values from the user or specify them in the code itself. We will store these values in the form of an array in A
. Then, all the values stored in array A
will be added to the variable TotalSum
using a for
loop. The length()
function returns the total number of elements in the array. Finally, we will calculate the average by dividing the sum by the total number of values and display it.
See the code below.
public class Average {
public static void main(String args[]) {
int TotalSum = 0;
int A[] = {1, 2, 3, 4, 5};
int n = A.length;
for (int i = 0; i < n; i++) {
TotalSum = TotalSum + A[i];
}
System.out.println("The average=" + (float) (TotalSum / n));
}
}
Output:
The average=3.0
In the above example, we also typecast the final value using the float()
function because the average values usually contain a decimal.