Button Group in Java
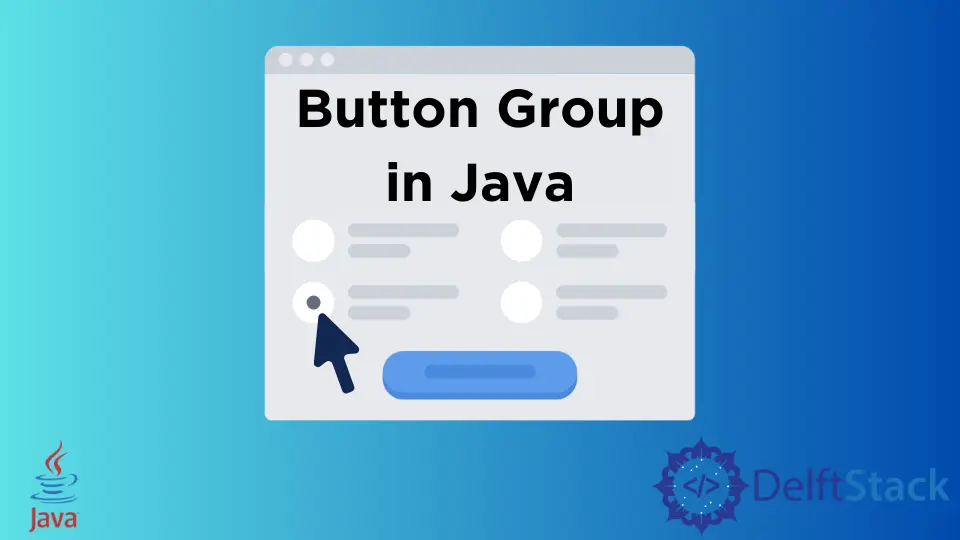
The button groups in Java are used with the radio button to ensure only one radio button is selected. Both Radio button and Button groups belong to Java’s Swing
library.
This tutorial demonstrates how to use button groups in Java.
Button Group in Java
Button group is used to create a group of radio buttons in Java, and to create the Button Group, we use the following methods and constructors.
JRadioButton Radio_Button1 = new JRadioButton("Radio Button Group 1"); // Creates a new radio button
JRadioButton Radio_Button2 = new JRadioButton(
"Radio Button Group 2", true); // Creates a radio button which is already selected
ButtonGroup Button_Group = new ButtonGroup(); // Creates new button group
Button_Group.add(Radio_Button1); // Add radio button to the button group
Let’s try an example of a button group with multiple radio buttons.
package delftstack;
import java.awt.BorderLayout;
import java.awt.FlowLayout;
import java.awt.LayoutManager;
import javax.swing.ButtonGroup;
import javax.swing.JFrame;
import javax.swing.JPanel;
import javax.swing.JRadioButton;
public class Button_Groups {
public static void main(String[] args) {
Create_Frame();
}
private static void Create_Frame() {
JFrame Demo_Frame = new JFrame("Button Groups");
Demo_Frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
CreateBG(Demo_Frame);
Demo_Frame.setSize(400, 200);
Demo_Frame.setLocationRelativeTo(null);
Demo_Frame.setVisible(true);
}
private static void CreateBG(final JFrame Demo_Frame) {
JPanel Demo_Panel = new JPanel();
LayoutManager Panel_Layout = new FlowLayout();
Demo_Panel.setLayout(Panel_Layout);
JRadioButton Radio_Button1 = new JRadioButton("Radio Button Group 1");
JRadioButton Radio_Button2 = new JRadioButton("Radio Button Group 2", true);
JRadioButton Radio_Button3 = new JRadioButton("Radio Button Group 3");
JRadioButton Radio_Button4 = new JRadioButton("Radio Button Group 4");
ButtonGroup Button_Group = new ButtonGroup();
Button_Group.add(Radio_Button1);
Button_Group.add(Radio_Button2);
Button_Group.add(Radio_Button3);
Button_Group.add(Radio_Button4);
Demo_Panel.add(Radio_Button1);
Demo_Panel.add(Radio_Button2);
Demo_Panel.add(Radio_Button3);
Demo_Panel.add(Radio_Button4);
Demo_Frame.getContentPane().add(Demo_Panel, BorderLayout.CENTER);
}
}
The code above creates a panel with four radio buttons in a button group. See the output in the animation below.
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook