How to Break Nested Loops in Java
-
Break the Inner Loop Using a
break
Statement in Java -
Break a nested Loop Using a
break
Statement With a Label in Java -
Break a Nested Loop Using a
break
Statement in Java -
Break a nested Loop Using a
flag
Variable in Java -
Break a nested Loop Using a
return
Statement in Java
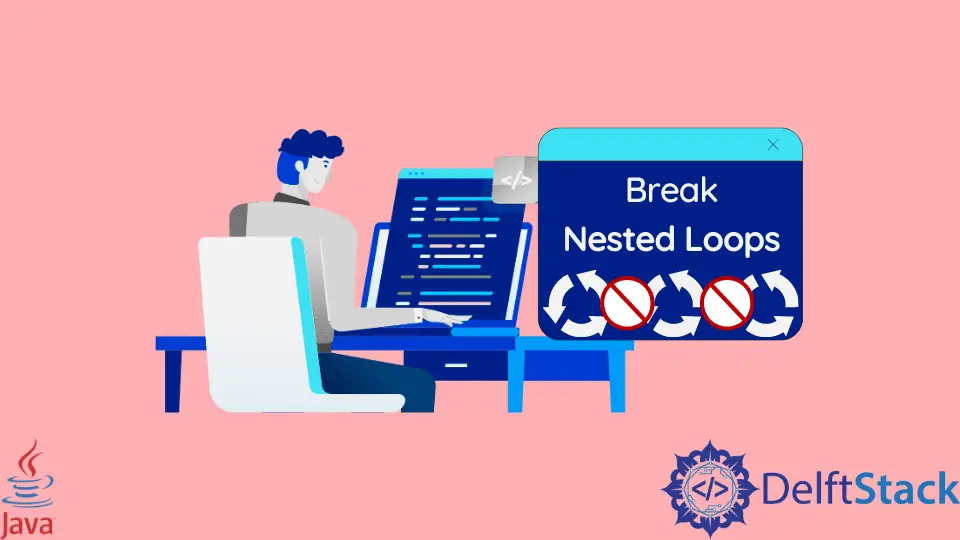
This tutorial introduces ways on how to break out nested loops in Java. We included some example programs you can follow as your guide.
A loop is a technique that allows us to repeat any code statement at any number of times depending on the given condition. Java supports several types of loops like the while
loop, do-while
loop, for
loop, and for-each
loop. We can nest these loops as well.
In this article, you’ll learn how to break out a nested loop in Java. There are several ways to break a nested loop; these include using break
and return
statements. Read on to find out more!
Break the Inner Loop Using a break
Statement in Java
If you want to break out a loop, then you can use the break
statement. This statement would break the inner loop only if you applied it in the inner loop. Here’s how you can do it:
public class SimpleTesting {
public static void main(String[] args) {
for (int i = 0; i < 5; i++) {
for (int j = 0; j < 5; j++) {
System.out.println(j);
if (i == 2) {
System.out.println("loop break");
break;
}
}
System.out.println();
}
}
}
Output:
0
1
2
3
4
0
1
2
3
4
0
loop break
0
1
2
3
4
0
1
2
3
4
Break a nested Loop Using a break
Statement With a Label in Java
If you want to break all the loops, both inner and outer, you can use a label with a break
statement that will cut out all the loops and move control to the outside loop. See the example below:
public class SimpleTesting {
public static void main(String[] args) {
out:
for (int i = 0; i < 5; i++) {
for (int j = 0; j < 5; j++) {
System.out.println(j);
if (i == 2) {
System.out.println("loop break");
break out;
}
}
System.out.println();
}
}
}
Output:
0
1
2
3
4
0
1
2
3
4
0
loop break
Break a Nested Loop Using a break
Statement in Java
A loop can of the while
, for
, or for-each
types, and we can use a break
statement in any of these loops. In this example, we are using a while
loop and breaking its flow by using a break
statement. See the example below:
public class SimpleTesting {
public static void main(String[] args) {
int i = 0;
out:
while (i < 5) {
int j = 0;
while (j < 5) {
System.out.println(j);
if (i == 2) {
System.out.println("loop break");
break out;
}
j++;
}
System.out.println();
i++;
}
}
}
Output:
0
1
2
3
4
0
1
2
3
4
0
loop break
Break a nested Loop Using a flag
Variable in Java
This method introduces another scenario where we are using a variable in the loop condition; when the condition has been met, the loop breaks out. This code is good if you don’t want to use a break
statement. This process is also better because it makes the code more readable. Follow the code block below:
public class SimpleTesting {
public static void main(String[] args) {
boolean flag = false;
for (int i = 0; i < 5 && !flag; i++) {
System.out.println(i);
if (i == 3) {
System.out.println("loop break");
flag = true;
}
}
}
}
Output:
0
1
2
3
loop break
Break a nested Loop Using a return
Statement in Java
The return
statement in Java is used to convey a response back to the caller method. We can use a return
statement in the loop to break it. It is an alternate of a break
statement but it can only work in certain scenarios only. See the example below:
public class SimpleTesting {
public static void main(String[] args) {
boolean isStop = iterate();
if (isStop)
System.out.println("Loop stop");
else
System.out.println("Loop not stop");
}
static boolean iterate() {
for (int i = 0; i < 5; i++) {
for (int j = 0; j < 5; j++) {
System.out.println(j);
if (i == 2) {
return true;
}
}
System.out.println();
}
return false;
}
}
Output:
0
1
2
3
4
0
1
2
3
4
0
Loop stop