How to Convert Boolean to String in Java
- Using String.valueOf() Method
- Using Boolean.toString() Method
- Using String concatenation
- Using String.format()
- Conclusion
- FAQ
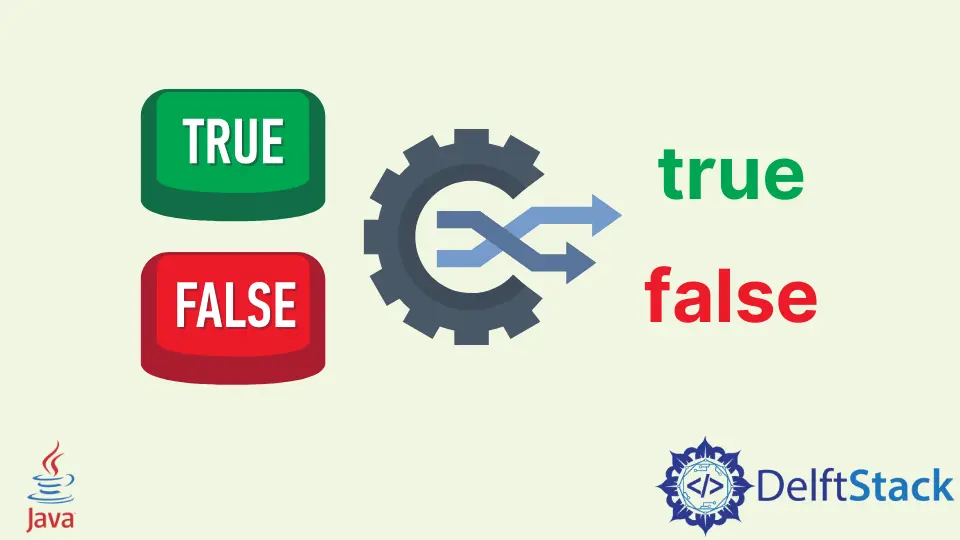
Java is a versatile programming language that often requires data type conversions for effective manipulation. One common scenario developers encounter is the need to convert a boolean value to a string. This might seem straightforward, but understanding the nuances can help avoid potential pitfalls.
In this tutorial, we will explore various methods to convert boolean values to strings in Java. Whether you are a beginner or an experienced programmer, this guide will provide you with clear examples and explanations to make the process seamless. Let’s dive in and unravel the simple yet crucial task of converting boolean to string in Java.
Using String.valueOf() Method
One of the simplest and most effective ways to convert a boolean to a string in Java is by using the String.valueOf()
method. This method is part of the String class and is designed to convert different data types to their string representations. When you pass a boolean value to this method, it returns the string “true” or “false” based on the boolean’s value.
Here’s how you can implement it:
boolean flag = true;
String result = String.valueOf(flag);
Output:
true
In this example, we declare a boolean variable named flag
and assign it the value true
. We then use String.valueOf(flag)
to convert this boolean into its string equivalent, which is stored in the variable result
. This method is advantageous due to its simplicity and readability. It is also a static method, meaning you can call it directly on the String class without needing an instance of it.
Using Boolean.toString() Method
Another effective way to convert a boolean to a string in Java is by using the Boolean.toString()
method. This method is specifically designed for boolean values and returns the string representation of the boolean.
Here’s a quick example:
boolean flag = false;
String result = Boolean.toString(flag);
Output:
false
In this snippet, we declare a boolean variable flag
with a value of false
. By calling Boolean.toString(flag)
, we convert this boolean into a string, which is then stored in the variable result
. This method is equally straightforward and enhances code clarity, especially when working specifically with boolean values. It’s a great choice when you want to emphasize that the conversion is intended for boolean types.
Using String concatenation
String concatenation is another method to convert a boolean to a string in Java. This approach involves appending the boolean value to an empty string. While it may seem less straightforward than the previous methods, it’s still a valid way to achieve the conversion.
Here’s how it works:
boolean flag = true;
String result = "" + flag;
Output:
true
In this example, we create a boolean variable flag
with a value of true
. By concatenating this boolean with an empty string (""
), Java automatically converts the boolean to its string representation. This method is simple and effective, but it can be less readable than the previous methods. It’s often used in scenarios where you are already working with strings and want to include boolean values without explicitly calling conversion methods.
Using String.format()
Another sophisticated way to convert a boolean to a string in Java is by using the String.format()
method. This method allows for formatted strings and can be particularly useful when you want to include boolean values within a larger string context.
Here’s an example:
boolean flag = false;
String result = String.format("%b", flag);
Output:
false
In this code snippet, we declare a boolean variable flag
with a value of false
. The String.format("%b", flag)
method converts the boolean to its string representation. The %b
format specifier is used to indicate that we want to format a boolean value. This method is powerful for creating complex strings that include various data types, making it a great choice for more advanced string manipulation tasks.
Conclusion
Converting a boolean to a string in Java is a fundamental task that can be accomplished using several methods. Whether you choose String.valueOf()
, Boolean.toString()
, string concatenation, or String.format()
, each method has its own advantages. Understanding these methods will not only enhance your coding skills but also improve your ability to write clean and efficient Java code. As you continue to work with Java, keep these techniques in mind, and you’ll find that converting data types becomes second nature.
FAQ
-
What is the easiest way to convert a boolean to a string in Java?
The easiest way is to use theString.valueOf()
method, which directly converts boolean values to their string representation. -
Can I use string concatenation to convert a boolean to a string?
Yes, string concatenation can be used to convert a boolean to a string by appending the boolean value to an empty string. -
Is there a method specifically for boolean values in Java?
Yes, theBoolean.toString()
method is specifically designed for converting boolean values to strings. -
What is the advantage of using String.format() for conversion?
String.format()
allows for formatted strings and can be useful when including boolean values within larger strings. -
Are there any performance differences between these methods?
Generally, the performance differences are negligible for small-scale applications, butString.valueOf()
andBoolean.toString()
are typically more efficient for simple conversions.
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedInRelated Article - Java Boolean
- How to Toggle a Boolean Variable in Java
- How to Print Boolean Value Using the printf() Method in Java
- How to Initialise Boolean Variable in Java
- How to Convert Boolean to Int in Java
- How to Convert String to Boolean in Java