Backslash Character in Java
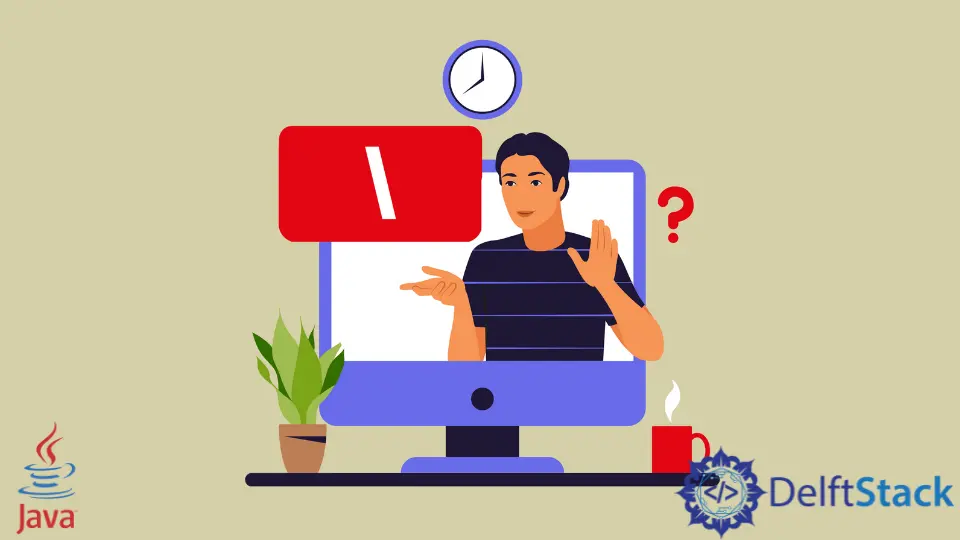
Escape characters or escape sequences play an important role in Java when it comes to formatting strings, and the backslash character is what makes a character an escape character. In this article, we will discuss the Backslash character.
Use Backslash to Escape Characters in Java
In the example below, we use a backslash to perform different tasks.
Although a backslash can escape several characters like \t
that inserts a tab, \b
that puts a backspace where it is placed, or \r
that is used for carriage return, but we talk about only three characters in the program.
The first string statement has the escape character \n
, used to insert a new line where it is placed. The output shows that the escape sequence breaks the statement and puts on a new line, even if it is a single string.
In Java, we use double quotes to represent a string, but if we want to show or use double quotes in the string itself, we cannot do it without escaping the quotes. We enclose the string with the escape characters \"
to escape the double-quotes.
The last string in the code below escapes the backslash itself, as it cannot be printed if a single backslash is used. This is why we use double backslashes.
public class JavaBackslash {
public static void main(String[] args) {
System.out.println("I am on the first line \nI am on the second line");
System.out.println(
"\"I am under double quotes because I am using a backslash to escape the double quotes.\"");
System.out.println("this\\is\\a\\path\\with\\escaped\\backslash");
}
}
Output:
I am on the first line
I am on the second line
"I am under double quotes because I am using a backslash to escape the double quotes."
this\is\a\path\with\escaped\backslash
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedIn